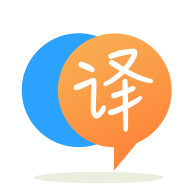
[英]How do I bind an ObservableConcurrentDictionary to WPF TreeView
[英]How do I properly bind a WPF TreeView control and effectively display a hierarchy of the data?
好的,所以我通常会等到最后一刻提交问题,但我真的可以使用一些帮助。
我正在尝试将ObservableCollection<Contact_Info>
绑定到WPF TreeView
控件。 Contact_Info包含联系信息的属性,并实现INotifyPropertyChanged
。 它还包含给定Contact
的更详细信息的列表。
我遇到两件事情有困难。 1)我需要在TreeViewItem
标题上显示公司的名称,但是,很多联系人都缺少公司名称,我想使用名字和姓氏。 我试图使用像FallBackValue
和TargetNullValue
这样的东西,但是CompanyName不是null,它只是空的所以我似乎无法做到这一点。 我需要转换器吗? 2)我似乎无法使层次结构正确,我希望同一AccountID的所有联系人在TreeViewItem
组合在一起。 目前,我只能在TreeViewItem
上显示公司名称并展开它显示一个空白值,并且仍然显示重复的公司名称。
我在DockPanel.Resources下放置HierarchicalDataTemplate的代码(不确定这是不是正确的地方)。
<local:Main_Interface x:Key="MyList" />
<HierarchicalDataTemplate DataType = "{x:Type
local:Contact_Info}" ItemsSource = "{Binding Path=OppDataList}">
<TextBlock Text="{Binding Path=CompanyName}"/>
</HierarchicalDataTemplate>
<DataTemplate DataType = "{x:Type sharpspring:SharpspringOpportunityDataModel}" >
<TextBlock Text="{Binding Path=ProjectName}"/>
</DataTemplate>
上面的代码是我在无数其他方面尝试过的最新代码。 我觉得我非常接近但是在这一点上我真的可以使用SO的帮助。
其他课程有点长,如果您需要更多,请告诉我。 非常感谢您提供的任何帮助!
编辑1:Contact_Info
public class Contact_Info : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
internal SharpspringLeadDataModel LeadDataModel;
internal SharpspringOpportunityDataModel OppData;
public List<SharpspringOpportunityDataModel> OppDataList { get; set; }// Should i make this ObservableCollection?
public Project_Info ProjectInfo { get; set; }
public bool IsDirty { get; set; }
public bool IsNew { get; set; }
#region Properties
public long AccountID
{
get => LeadDataModel.AccountID;
set
{
if (value != LeadDataModel.AccountID)
{
LeadDataModel.AccountID = value;
NotifyPropertyChanged();
}
}
}
public long LeadID
{
get => LeadDataModel.LeadID;
set
{
if (value != LeadDataModel.LeadID)
{
LeadDataModel.LeadID = value;
NotifyPropertyChanged();
}
}
}
// Lead Info
public string CompanyName
{
get => LeadDataModel.CompanyName;
set
{
if (value != LeadDataModel.CompanyName)
{
LeadDataModel.FaxNumber = value;
NotifyPropertyChanged();
}
}
}
public Contact_Info(SharpspringLeadDataModel lead, SharpspringOpportunityDataModel opp)
{
LeadDataModel = lead;
OppData = opp;
ProjectInfo = new Project_Info(this);
}
public SharpspringLeadDataModel GetDataModel()
{
return LeadDataModel;
}
public SharpspringOpportunityDataModel GetOppModel()
{
return OppData;
}
public Project_Info GetProjectInfoModel()
{
return ProjectInfo;
}
public void NotifyPropertyChanged([CallerMemberName] string propertyName = "")
{
IsDirty = true;
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
编辑2:当前填充树,但不会将公司组合在一起......
private void PopulateTreeView(List<SharpspringLeadDataModel> lead_list, List<SharpspringOpportunityDataModel> opp_list)
{
Contacts = new ObservableCollection<Contact_Info>();
var complete2 =
from lead in lead_list
let oppList = from o in opp_list
where o.PrimaryLeadID == lead.LeadID
select o
select new Contact_Info()
{
LeadDataModel = lead,
OppData = oppList.DefaultIfEmpty(new SharpspringOpportunityDataModel()).First(),
OppDataList = oppList.ToList(),
};
Contacts = complete2.ToObservableCollection();
Lead_Treeview.ItemsSource = Contacts;
}
我认为您的问题实际上是使用List
而不是OppDataList
的ObservableCollection
。 使用你的代码(减少到最小需要),但使用ObservableCollection
驱动集合,它对我来说很好。
请注意,我的代码可以正常使用List,因为它全部是静态的,但在适当的应用程序中,您需要OC来跟踪集合中的更改。
免责声明:我正在使用代码来回答这个问题,以保持简单,但我不建议在任何真正的应用程序中使用任何此类
MainWindow.xaml <Window x:Class="WpfApp1.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:local="clr-namespace:WpfApp1" mc:Ignorable="d" Title="MainWindow" Height="240" Width="320"> <TreeView ItemsSource="{Binding Contacts}"> <TreeView.ItemTemplate> <HierarchicalDataTemplate DataType="{x:Type local:Contact_Info}" ItemsSource="{Binding Path=OppDataList}"> <HierarchicalDataTemplate.ItemTemplate> <DataTemplate DataType="{x:Type local:SharpspringOpportunityDataModel}"> <TextBlock Text="{Binding Path=ProjectName}" /> </DataTemplate> </HierarchicalDataTemplate.ItemTemplate> <TextBlock Text="{Binding Path=CompanyName}" /> </HierarchicalDataTemplate> </TreeView.ItemTemplate> </TreeView> </Window>
MainWindow.xaml.cs
using System.Collections.ObjectModel; using System.ComponentModel; using System.Runtime.CompilerServices; using WpfApp1.Annotations; namespace WpfApp1 { public partial class MainWindow : INotifyPropertyChanged { public MainWindow() { InitializeComponent(); DataContext = this; } public ObservableCollection<Contact_Info> Contacts { get; } = new ObservableCollection<Contact_Info> { new Contact_Info { CompanyName = "Apple", OppDataList = {new SharpspringOpportunityDataModel {ProjectName = "World take over"}} }, new Contact_Info { CompanyName = "Google", OppDataList = {new SharpspringOpportunityDataModel {ProjectName = "World take over"}} }, new Contact_Info { CompanyName = "Microsoft", OppDataList = {new SharpspringOpportunityDataModel {ProjectName = "World take over"}} } }; public event PropertyChangedEventHandler PropertyChanged; [NotifyPropertyChangedInvocator] protected virtual void OnPropertyChanged([CallerMemberName] string propertyName = null) { PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName)); } } public class Contact_Info { public string CompanyName { get; set; } public ObservableCollection<SharpspringOpportunityDataModel> OppDataList { get; } = new ObservableCollection<SharpspringOpportunityDataModel>(); } public class SharpspringOpportunityDataModel { public string ProjectName { get; set; } } }
截图
根据我的理解,您的OppDataList是给定合同信息的子属性,您希望它是可折叠的。
在这种情况下你可以做到
<ListBox ItemsSource="{Binding YourContactList}">
<ListBox.ItemTemplate>
<DataTemplate>
<Expander Header="{Binding ProjectName}">
<Expander Header="{Binding CompanyName}">
<ListBox ItemsSource="{Binding OppDataList}">
<!--Your OppDataList display-->
</ListBox>
</Expander>
</Expander>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
最终结果看起来与此类似
+Contract1
-Contract2
-Project2
-Company2
-Opp
1
2
3
+Contract3
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.