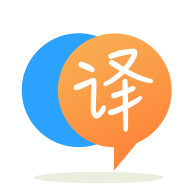
[英]How can I filter an array of dictionaries in 'updateSearchResultsForSearchController' to search a UITableView with Swift
[英]How can sort and categorize an array of dictionaries as alphabetically in the UITableView as sections in swift 3.0
我正在快速学习,并且有很多类似的字典,
[{“添加”:2017年12月24日,“ first_name”:阿卜杜拉,“电子邮件”:spaeker1@example.com,“ Last_name”:Jaleel,“ place”:印度,“称呼”:Mr},{“添加“:2017-12-24,” first_name“:Catherine,”电子邮件“:spaeker1@example.com,”姓氏“:Rose,”地点“:印度,”敬礼“:Mrs},{”添加“:2017- 12-24,“ first_name”:Alok,“ email”:spaeker1@example.com,“ Last_name”:Raj,“ place”:印度,“ salutation”:Mr},{“添加”:2017-12-24, “ first_name”:达尔文,“电子邮件”:spaeker1@example.com,“ Last_name”:Jose,“ place”:印度,“ salutation”:Mr}]。
在这里,我想根据first_name按字母顺序列出UITableView中的所有发言人。 我可以按字母顺序对字典数组进行排序,但是问题是我想在UITableViewSection中显示字母,并且该部分仅包含仅以该字母开头的名称(例如iPhone的联系人屏幕)。
{a:[{“添加”:2017年12月24日,“ first_name”:阿卜杜拉,“电子邮件”:spaeker1@example.com,“ Last_name”:Jaleel,“ place”:印度,“ salutation”:Mr}, {“添加”:2017-12-24,“名字”:Alok,“电子邮件”:spaeker1@example.com,“姓氏”:Raj,“地点”:印度,“称呼”:先生}],c:[ {“添加”:2017年12月24日,“ first_name”:凯瑟琳,“电子邮件”:spaeker1@example.com,“ Last_name”:Rose,“ place”:印度,“ salutation”:Mrs}],d:[ {“添加”:2017-12-24,“名字”:达尔文,“电子邮件”:spaeker1@example.com,“姓氏”:Jose,“地点”:印度,“称呼”:先生}]}。
是否可以如上所述对字典数组进行分组? 否则,如何在UITableViewSection中按字母顺序列出所有发言人。 请帮我。
您可以遍历字典数组,然后准备结果。
码
let dict = [ ["added": "2017-12-24", "first_name": "Abdullah", "email": "spaeker1@example.com", "Last_name": "Jaleel", "place": "India", "salutation":"Mr"], ["added": "2017-12-24", "first_name": "Catherine", "email": "spaeker1@example.com", "Last_name": "Rose", "place": "India", "salutation":"Mrs"], ["added": "2017-12-24", "first_name": "Alok", "email": "spaeker1@example.com", "Last_name": "Raj", "place": "India", "salutation":"Mr"], ["added": "2017-12-24", "first_name": "Darwin", "email": "spaeker1@example.com", "Last_name": "Jose", "place": "India", "salutation":"Mr"]]
var result: [String:[[String:String]]] = [:]
for d in dict {
var tempContactArray: [[String:String]] = []
let firstName = d["first_name"]?.lowercased()
let firstChar = firstName![0]
if let data = result[firstChar] {
tempContactArray = data
}
tempContactArray.append(d)
result[firstChar] = tempContactArray
}
let sortedKeysAndValues = result.sorted(by: { $0.0 < $1.0 })
print(sortedKeysAndValues)
将字符串的扩展写为
extension String {
subscript (i: Int) -> String {
return String(self[index(startIndex, offsetBy: i)] as Character)
}
}
输出sortedKeysAndValues
[(key: "a", value: [["added": "2017-12-24", "Last_name": "Jaleel", "place": "India", "email": "spaeker1@example.com", "first_name": "Abdullah", "salutation": "Mr"], ["added": "2017-12-24", "Last_name": "Raj", "place": "India", "email": "spaeker1@example.com", "first_name": "Alok", "salutation": "Mr"]]), (key: "c", value: [["added": "2017-12-24", "Last_name": "Rose", "place": "India", "email": "spaeker1@example.com", "first_name": "Catherine", "salutation": "Mrs"]]), (key: "d", value: [["added": "2017-12-24", "Last_name": "Jose", "place": "India", "email": "spaeker1@example.com", "first_name": "Darwin", "salutation": "Mr"]])]
您无法对字典进行排序,因为字典是元素的无序集合。 因此,如果要排序,则必须为数组。
我想您可能会发现通过使用reduce
+ sorted
组合可以发现有用,这样您可以按字母顺序对字典进行分组,并将first_name
第一个字符添加为键:
斯威夫特3.0
let arrayOfDict = [ ["added": "2017-12-24", "first_name": "Abdullah", "email": "spaeker1@example.com", "Last_name": "Jaleel", "place": "India", "salutation":"Mr"],["added": "2017-12-24", "first_name": "Catherine", "email": "spaeker1@example.com", "Last_name": "Rose", "place": "India", "salutation":"Mrs"],["added": "2017-12-24", "first_name": "Alok", "email": "spaeker1@example.com", "Last_name": "Raj", "place": "India", "salutation":"Mr"],["added": "2017-12-24", "first_name": "Darwin", "email": "spaeker1@example.com", "Last_name": "Jose", "place": "India", "salutation":"Mr"]]
var result = arrayOfDict.reduce([String: [[String:Any]]]()) { res, element in
var mutableRes = res
if let firstLetter = element["first_name"]?.characters.prefix(0) {
let initial = String(describing: firstLetter).lowercased()
if mutableRes[initial] == nil {
mutableRes[initial] = [[String:Any]]()
}
mutableRes[initial]?.append(element)
}
return mutableRes
}.sorted { return $0.key < $1.key }
迅捷4.0
let arrayOfDict = [ ["added": "2017-12-24", "first_name": "Abdullah", "email": "spaeker1@example.com", "Last_name": "Jaleel", "place": "India", "salutation":"Mr"],["added": "2017-12-24", "first_name": "Catherine", "email": "spaeker1@example.com", "Last_name": "Rose", "place": "India", "salutation":"Mrs"],["added": "2017-12-24", "first_name": "Alok", "email": "spaeker1@example.com", "Last_name": "Raj", "place": "India", "salutation":"Mr"],["added": "2017-12-24", "first_name": "Darwin", "email": "spaeker1@example.com", "Last_name": "Jose", "place": "India", "salutation":"Mr"]]
let result = arrayOfDict.reduce(into: [String: [[String:Any]]]()) { result, element in
if let firstLetter = element["first_name"]?.first {
let initial = String(describing: firstLetter).lowercased()
result[initial, default: [[String:Any]]() ].append(element)
}}.sorted { return $0.key < $1.key }
print(result)
最终结果将是:
[(key: "a", value: [["added": "2017-12-24", "Last_name": "Jaleel", "place": "India", "email": "spaeker1@example.com", "first_name": "Abdullah", "salutation": "Mr"], ["added": "2017-12-24", "Last_name": "Raj", "place": "India", "email": "spaeker1@example.com", "first_name": "Alok", "salutation": "Mr"]]), (key: "c", value: [["added": "2017-12-24", "Last_name": "Rose", "place": "India", "email": "spaeker1@example.com", "first_name": "Catherine", "salutation": "Mrs"]]), (key: "d", value: [["added": "2017-12-24", "Last_name": "Jose", "place": "India", "email": "spaeker1@example.com", "first_name": "Darwin", "salutation": "Mr"]])]
是否可以如上所述对字典数组进行分组?
let users = [
["added": "2017-12-24", "first_name": "Abdullah", "email": "spaeker1@example.com", "Last_name": "Jaleel", "place": "India", "salutation": "Mr"],
["added": "2017-12-24", "first_name": "Catherine", "email": "spaeker1@example.com", "Last_name": "Rose", "place": "India", "salutation": "Mrs"],
["added": "2017-12-24", "first_name": "Alok", "email": "spaeker1@example.com", "Last_name": "Raj", "place": "India", "salutation": "Mr"],
["added": "2017-12-24", "first_name": "Darwin", "email": "spaeker1@example.com", "Last_name": "Jose", "place": "India", "salutation": "Mr"]
]
func firstCharOfFirstName(_ aDict: [String:String]) -> Character {
return aDict["first_name"]!.first!
}
let groupedUsers = Dictionary(grouping: users, by: firstCharOfFirstName)
print(groupedUsers)
--output:--
["C":
[["added": "2017-12-24", "Last_name": "Rose", "place": "India", "email": "spaeker1@example.com", "first_name": "Catherine", "salutation": "Mrs"]],
"D":
[["added": "2017-12-24", "Last_name": "Jose", "place": "India", "email": "spaeker1@example.com", "first_name": "Darwin", "salutation": "Mr"]],
"A":
[["added": "2017-12-24", "Last_name": "Jaleel", "place": "India", "email": "spaeker1@example.com", "first_name": "Abdullah", "salutation": "Mr"],
["added": "2017-12-24", "Last_name": "Raj", "place": "India", "email": "spaeker1@example.com", "first_name": "Alok", "salutation": "Mr"]]]
然后,您可以根据需要对每个组进行排序。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.