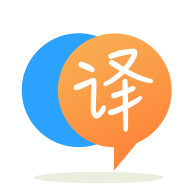
[英]How do you create a many-to-many relationship in Entity Framework if the database doesn't have a linking table?
[英]How do you connect a 1 to many table
我有一个ASP.NET Core项目,该项目具有以下两个表:City和Country。
public class City
{
[Key]
public int Id { get; set; }
public string CityName{ get; set; }
public string Region{ get; set; }
public int CountryID { get; set; }
public Country Country { get; set; }
}
public class Country
{
[Key]
public int Id { get; set; }
[Required]
public string CountryName{ get; set; }
[Required]
public string Continent{ get; set; }
[Required]
public string Capital{ get; set; }
[Required]
public int NumberOfPeople{ get; set; }
}
一个城市类继承一个国家的外键,并且一个国家可以拥有多个城市,即1-(0..M)。 它们都来自同一个DbContext
。
我希望能够单击“国家/地区”索引页面上的详细信息页面(显示所有国家/地区,其属性和选项),以便显示国家/地区信息以及该国家/地区的所有城市,但我无法确定了解如何通过DbContext
连接两个表。
如何将加载特定国家(地区)信息和特定ID以及内部所有城市的模型传递给详细信息视图
如果要在Country
包括所有City
,则需要从Country
到City
添加导航属性(已配置关系的另一部分):
public class Country
{
...
public ICollection<City> Cities { get; set; }
}
这使您可以执行单个数据库查询以带来所有结果,而不必进行手动联接。
然后,您可以将其用作:
var country = await context.Countries
.Include(x => x.Cities)
.SingleAsync(x => x.ID == someId);
您可以使用查询执行程序的非Async
版本,但是鉴于您使用的是ASP.NET Core应用程序,因此建议您不要这样做。
一种方法是对字段使用属性:
public class City
{
[Key]
public int Id { get; set; }
public string CityName{ get; set; }
public string Region{ get; set; }
public int CountryID { get; set; }
[ForeignKey("CountryID")]
public virtual Country Country { get; set; }
}
public class Country
{
[Key]
public int Id { get; set; }
[Required]
public string CountryName{ get; set; }
[Required]
public string Continent{ get; set; }
[Required]
public string Capital{ get; set; }
[Required]
public int NumberOfPeople{ get; set; }
[InverseProperty("Country")]
public virtual ICollection<City> Cities { get; set; }
}
有多种方法可以做到这一点。
首先,您应该声明您的类,例如:
public class City
{
[Key]
public int Id { get; set; }
public string CityName{ get; set; }
public string Region{ get; set; }
public int CountryId { get; set; }
public Country Country { get; set; }
}
public class Country
{
[Key]
public int Id { get; set; }
[Required]
public string CountryName{ get; set; }
[Required]
public string Continent{ get; set; }
[Required]
public string Capital{ get; set; }
[Required]
public int NumberOfPeople{ get; set; }
public virtual ICollection<City> Cities { get; set; }
}
其次,您应该配置关系:
protected override void OnModelCreating(Modelbuilder modelBuilder)
{
modelBuilder.Entity<Country>()
.HasMany(c => c.Cities)
.WithRequired(e => e.Country)
.HasForeignKey(c => c.CountryId);
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.