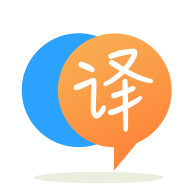
[英]In ASP how do I call a JavaScript function in one .asp file from another .asp file written in VB?
[英]How do I call up another JavaScript file from one main file?
我正在使用discord.js库为Discord创建一个机器人。 我正在尝试创建一个文件,以便在执行该文件时,它将加载每个模块,而无需多次运行node file.js
。 该文件(我们称为startup.js
)位于modules文件夹中。 假设我要加载Bot/modules/diagnostics
File1.js
, File2.js
和File3.js
,而startup.js是Bot/modules
。 我希望文件异步加载,并且顺序并不重要。
感谢所有帮助。
您可以将File1,File2和File3导入为模块。 第一种方法:
//File1.js:
module.exports = {
nameOfYourFunction: () => {
//Your code here
},
otherFunction: (args) => {
//Your code here
}
};
//Do the same for File2, File3, and other files.
//startup.js:
const file1 = require("./diagnostics/File1.js"), file2 = require("./diagnostics/File2.js"), file3 = require("./diagnostics/File3.js");
// Then use this to run your code from File1.js:
file1.nameOfYourFunction();
file1.otherFunction(args);
//Do the same thing to run your code from other files
第二种方法:
/* If you only have one function that you want to run,
you can set the module's entire exports to a function. This method will
not allow you to create multiple functions.*/
//File1.js:
module.exports = (args) => {
//Your code here
};
//Do the same thing or the First method for other files.
//startup.js:
const file1 = require("./diagnostics/File1.js"), file2 = require("./diagnostics/File2.js"), file3 = require("./diagnostics/File3.js");
//To run your code:
file1(args);
编辑:使用这些方法时要小心,因为它们的范围与startup.js中的主要代码不同。
要给出更详细的答案,有几种方法可以做到这一点。 第一种方法是将module.exports与对象一起使用。 这将允许您使用require
导入模块(或使用babel或webpack等编译器import
),并且可以调用对象中的任何函数。 这是使用@MaxxiBoi的响应方法。
// File 1
module.exports = {
"myFunction1": (arg1, arg2) => {
console.log("Function 1 with 2 args: "+ arg1 + " " + arg2);
},
"myFunction2": () => {
console.log("Function 2");
}
}
// File 2
const myModule = require("./file1.js");
myModule.myFunction1(null, "Hi"); // Logs "Function 1 with 2 args: null Hi"
myModule.myFunction2(); // Logs "Function 2"
虽然这在您要输出多个功能的情况下很有用,但是如果每个模块只需要一个功能,我就不会这样做。
第二种方法是使用带有变量或函数的module.exports而不是对象。 这样可以保持混乱并使其更易于理解。
// File 1
module.exports = myFunction1(arg1, arg2) {
console.log("Function 1 with 2 args: " + arg1 + " " + arg2);
}
// File 2
const myFunction = require("./file1.js");
myFunction(null, "Hi"); // Logs "Function 1 with 2 args: null Hi"
最后,还有另一种使用ES5或ES6创建构造函数的方法(在本例中,我使用ES6),这将使您能够向其中传递更多变量,然后可以在所述类中引用该变量。 在此示例中,我使用Discord.js客户端,并从构造函数中获取客户端的名称。 假设客户的名字是“乔治”。
// File 1
module.exports = class MyClass {
constructor(client) {
this.client = client;
}
myFunction1(myVar2) {
console.log("Function 1 with 2 args: " + this.client.user.username + " " + myVar2);
}
myFunction2() {
console.log("Function 2");
}
}
// File 2
const MyClass = require("./MyClass.js");
const myClassInstance = new MyClass(client);
myClassInstance.myFunction1("Hi"); // Logs "Function1 with 2 args: George Hi"
myClassInstance.myFunction2(); // Logs "Function 2"
最后,这完全取决于您的喜好以及您的操作方式。 每种方法都有其起伏。 如果您想了解有关我如何制作所有这些内容以及模块一般如何工作的更多信息,请查看Node.js文档说明 。 要了解有关类(第三个模块中使用的类)的更多信息,请查看MDN文档 。 希望我能够为您提供帮助。 您可能还想看看这个StackOverflow问题 ,因为它将解决您有关引用不同目录中文件的问题。 编码愉快!
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.