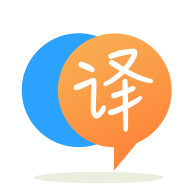
[英]How do you provide AWS keys to Amazon SES service when sending emails?
[英]Add embedded image in emails in AWS SES service
我正在尝试编写一个 Java 应用程序,它可以发送电子邮件以指定电子邮件。 在 email 我也想附上一些照片。
请在下面找到我的代码:-
public class AmazonSESSample {
static final String FROM = "abc@gmail.com";
static final String TO = "def@gmail.com";
static final String BODY = "This email was sent through Amazon SES by using the AWS SDK for Java. hello";
static final String SUBJECT = "Amazon SES test (AWS SDK for Java)";
public static void main(String[] args) throws IOException {
Destination destination = new Destination().withToAddresses(new String[] { TO });
Content subject = new Content().withData(SUBJECT);
Message msg = new Message().withSubject(subject);
// Include a body in both text and HTML formats
//Content textContent = new Content().withData("Hello - I hope you're having a good day.");
Content htmlContent = new Content().withData("<h2>Hi User,</h2>\n"
+ " <h3>Please find the ABC Association login details below</h3>\n"
+ " <img src=\"logo.png\" alt=\"Mountain View\">\n"
+ " Click <a href=\"http://google.com">here</a> to go to the association portal.\n"
+ " <h4>Association ID - 12345</h4>\n" + " <h4>Admin UID - suny342</h4>\n"
+ " <h4>Password - poass234</h4>\n" + " Regards,\n" + " <br>Qme Admin</br>");
Body body = new Body().withHtml(htmlContent);
msg.setBody(body);
SendEmailRequest request = new SendEmailRequest().withSource(FROM).withDestination(destination)
.withMessage(msg);
try {
System.out.println("Attempting to send an email through Amazon SES by using the AWS SDK for Java...");
AWSCredentials credentials = null;
credentials = new BasicAWSCredentials("ABC", "CDF");
try {
// credentialsProvider.
} catch (Exception e) {
throw new AmazonClientException("Cannot load the credentials from the credential profiles file. "
+ "Please make sure that your credentials file is at the correct "
+ "location (/Users/iftekharahmedkhan/.aws/credentials), and is in valid format.", e);
}
AmazonSimpleEmailService client = AmazonSimpleEmailServiceClientBuilder.standard()
.withCredentials(new AWSStaticCredentialsProvider(credentials)).withRegion("us-west-2").build();
client.sendEmail(request);
System.out.println("Email sent!");
} catch (Exception ex) {
System.out.println("The email was not sent.");
System.out.println("Error message: " + ex.getMessage());
}
}
}
该图像位于资源目录中,但未嵌入 email 中。任何人都可以帮忙。
您需要使用图像本身的绝对公共路径或数据 URL来代替相对路径。 例如:
<img src=\"https://example.com/logo.png\" alt=\"Mountain View\" />
或
<img src=\"data:image/png;base64, {BASE64_ENCODED_DATA}\" alt=\"Mountain View\" />
编辑
截至 2020 年 1 月,Gmail 仍不支持 base64 编码的图像。
@sebagra 发布的方法效果很好。
在Python使用boto3
和ses
客户端的情况下,设置Content-Disposition
为inline的方式是:
att.add_header('Content-ID', '<myImage>')
att.add_header('Content-Disposition', 'inline', filename=os.path.basename(IMAGE_PATH))
完整示例基于AWS 文档中的 python 示例:
import os
import boto3
from botocore.exceptions import ClientError
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.application import MIMEApplication
# Replace sender@example.com with your "From" address.
# This address must be verified with Amazon SES.
SENDER = "Sender Name <sender@example.com>"
# Replace recipient@example.com with a "To" address. If your account
# is still in the sandbox, this address must be verified.
RECIPIENT = "recipient@example.com"
# Specify a configuration set. If you do not want to use a configuration
# set, comment the following variable, and the
# ConfigurationSetName=CONFIGURATION_SET argument below.
CONFIGURATION_SET = "ConfigSet"
# If necessary, replace us-west-2 with the AWS Region you're using for Amazon SES.
AWS_REGION = "us-west-2"
# The subject line for the email.
SUBJECT = "Customer service contact info"
# The full path to the file that will be attached to the email.
IMAGE_PATH = "path/to/myImage.png"
# The email body for recipients with non-HTML email clients.
BODY_TEXT = "Hello,\r\nPlease see the attached file for a list of customers to contact."
# The HTML body of the email.
BODY_HTML = """\
<html>
<head></head>
<body>
<h1>Hello!</h1>
<p>Please see the attached file for a list of customers to contact.</p>
</body>
</html>
"""
# The character encoding for the email.
CHARSET = "utf-8"
# Create a new SES resource and specify a region.
client = boto3.client('ses',region_name=AWS_REGION)
# Create a multipart/mixed parent container.
msg = MIMEMultipart('mixed')
# Add subject, from and to lines.
msg['Subject'] = SUBJECT
msg['From'] = SENDER
msg['To'] = RECIPIENT
# Create a multipart/alternative child container.
msg_body = MIMEMultipart('alternative')
# Encode the text and HTML content and set the character encoding. This step is
# necessary if you're sending a message with characters outside the ASCII range.
textpart = MIMEText(BODY_TEXT.encode(CHARSET), 'plain', CHARSET)
htmlpart = MIMEText(BODY_HTML.encode(CHARSET), 'html', CHARSET)
# Add the text and HTML parts to the child container.
msg_body.attach(textpart)
msg_body.attach(htmlpart)
# Define the attachment part and encode it using MIMEApplication.
att = MIMEApplication(open(IMAGE_PATH, 'rb').read())
# Add a header to tell the email client to treat this part as an attachment,
# and set an id and content disposition.
att.add_header('Content-ID', '<myImage>')
att.add_header('Content-Disposition', 'inline', filename=os.path.basename(IMAGE_PATH))
# Attach the multipart/alternative child container to the multipart/mixed
# parent container.
msg.attach(msg_body)
# Add the attachment to the parent container.
msg.attach(att)
try:
response = client.send_raw_email(
Source=SENDER,
Destinations=[
RECIPIENT
],
RawMessage={
'Data': msg.as_string(),
}
)
# Display an error if something goes wrong.
except ClientError as e:
print(e.response['Error']['Message'])
else:
print("Email sent! Message ID:"),
print(response['MessageId'])
在使用sesv2
的情况下, msg
构建相同,但要使用的 api 是send_email
:
...
client = boto3.client('sesv2',region_name=AWS_REGION)
...
response = client.send_email(
FromEmailAddress=SENDER,
Destination={
'ToAddresses': [
RECIPIENT
]
},
Content={
'Raw': {
'Data': msg.as_string()
}
}
)
...
我使用 AWS SES 将内联 base 64 图像发送到雅虎帐户没有问题。 当我尝试发送到 GMail 帐户时遇到了问题。 我发送的文本已呈现,但图像未显示。
我发现 GMail 没有剥离图像。 它只是没有显示它。 我通过在 GMail 中查看邮件时选择“更多”->“显示原件”来确认这一点。
通过将图像附加到消息并使用对它们的内联处置引用,我能够使用 AWS SES 发送一封电子邮件,其中包含可以在 GMail 客户端中看到的图像。
我使用AWS 文档中解释的代码将图像附加到 MimeMessage,然后使用来自 HTML 的cid
引用到这些图像(如本文回答中所述)。
首先,我们将图像附加到消息中,添加几个特定属性(Header 和 Disposition):
MimeMultipart msg = new MimeMultipart("mixed");
DataSource fds = new FileDataSource("/path/to/my/image.png");
att.setDataHandler(new DataHandler(fds));
att.setFileName(fds.getName());
att.setHeader("Content-ID","<myImage>");
att.setDisposition("inline; filename=\"image.png\"");
msg.addBodyPart(att);
请注意,Content-ID 属性中的<
和>
必须包含您选择的任何 id(在我的示例中为myImage
)。
然后,在消息正文的 HTML 中,我们只需要添加每个图像的 cid(内容 id):
<img src="cid:myImage">
对于完整的代码,我几乎使用了上面的 AWS 参考(使用相同的变量名),所做的唯一更改是setHeader
和setDisposition
方法。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.