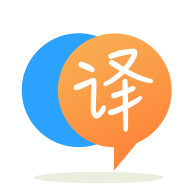
[英]Using `@ConfigurationProperties` annotation on `@Bean` Method
[英]Using @ConfigurationProperties in a @Scheduled annotation by referencing the bean name
我正在使用@ConfigurationProperties
来配置 Spring boot 中后台任务的延迟,并且我试图从另一个组件上的@Scheduled
注释中使用此值。 但是,为了使它工作,我必须使用 Spring 给 bean 的全名。
配置属性类如下:
@ConfigurationProperties("some")
class SomeProperties {
private int millis; //the property is some.millis
public int getMillis() {
return millis;
}
public void setMillis(int millis) {
this.millis = millis;
}
}
我在预定方法中使用如下值:
@Component
class BackgroundTasks {
@Scheduled(fixedDelayString = "#{@'some-com.example.demo.SomeProperties'.millis}") //this works.
public void sayHello(){
System.out.println("hello");
}
}
是否可以在不必使用 bean 的全名的情况下引用该值? 这个答案表明这是可能的,但我无法让它发挥作用。
在属性类上使用@Component
允许以"#{@someProperties.persistence.delay}
访问该属性。
弹簧启动文档中的更多信息。
一些属性.java
package com.example.demo;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
@ConfigurationProperties("some")
@Component("someProperties")
public class SomeProperties {
private int millis; // the property is some.millis
public int getMillis() {
return millis;
}
public void setMillis(int millis) {
this.millis = millis;
}
}
后台任务.java
package com.example.demo;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class BackgroundTasks {
/**
*
* Approach1: We can inject/access the property some.millis directly in @Value
* fashion even though we have configured it to bind with SomeProperties class
* using @ConfigurationProperties.
*
*
*/
@Scheduled(fixedRateString = "${some.millis}")
public void fromDirectInjection() {
System.out.println("Hi, I'm from DirectInjection method");
}
/**
*
* Approach2: Add @Component on SomeProperties and access the bean bean's
* property millis like below using Spring Expression language
*
*
*/
@Scheduled(fixedRateString = "#{@someProperties.millis}")
public void fromConfigurationProperty() {
System.out.println("Hi, I'm from ConfigurationProperty method");
}
}
演示应用程序.java
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableScheduling;
@SpringBootApplication
@EnableScheduling
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
应用程序属性
some.millis=2000
使用的版本
SpringBoot 2.6.0
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.0-SNAPSHOT</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>demo</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>11</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
<repositories>
<repository>
<id>spring-milestones</id>
<name>Spring Milestones</name>
<url>https://repo.spring.io/milestone</url>
<snapshots>
<enabled>false</enabled>
</snapshots>
</repository>
<repository>
<id>spring-snapshots</id>
<name>Spring Snapshots</name>
<url>https://repo.spring.io/snapshot</url>
<releases>
<enabled>false</enabled>
</releases>
</repository>
</repositories>
<pluginRepositories>
<pluginRepository>
<id>spring-milestones</id>
<name>Spring Milestones</name>
<url>https://repo.spring.io/milestone</url>
<snapshots>
<enabled>false</enabled>
</snapshots>
</pluginRepository>
<pluginRepository>
<id>spring-snapshots</id>
<name>Spring Snapshots</name>
<url>https://repo.spring.io/snapshot</url>
<releases>
<enabled>false</enabled>
</releases>
</pluginRepository>
</pluginRepositories>
</project>
输出:
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.6.0-SNAPSHOT)
2021-10-08 08:38:03.342 INFO 8092 --- [ main] com.example.demo.DemoApplication : Starting DemoApplication using Java 14.0.2 on TO-GZ9M403-L with PID 8092 (D:\workspaces\configprop\demo (1)\demo\target\classes started by D1 in D:\workspaces\configprop\demo (1)\demo)
2021-10-08 08:38:03.345 INFO 8092 --- [ main] com.example.demo.DemoApplication : No active profile set, falling back to default profiles: default
2021-10-08 08:38:04.884 INFO 8092 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http)
2021-10-08 08:38:04.902 INFO 8092 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat]
2021-10-08 08:38:04.902 INFO 8092 --- [ main] org.apache.catalina.core.StandardEngine : Starting Servlet engine: [Apache Tomcat/9.0.53]
2021-10-08 08:38:05.050 INFO 8092 --- [ main] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext
2021-10-08 08:38:05.050 INFO 8092 --- [ main] w.s.c.ServletWebServerApplicationContext : Root WebApplicationContext: initialization completed in 1631 ms
2021-10-08 08:38:05.644 INFO 8092 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path ''
Hi, I'm from DirectInjection method
Hi, I'm from ConfigurationProperty method
2021-10-08 08:38:05.662 INFO 8092 --- [ main] com.example.demo.DemoApplication : Started DemoApplication in 2.995 seconds (JVM running for 3.712)
Hi, I'm from DirectInjection method
Hi, I'm from ConfigurationProperty method
Hi, I'm from DirectInjection method
Hi, I'm from ConfigurationProperty method
Hi, I'm from DirectInjection method
Hi, I'm from ConfigurationProperty method
假设默认情况下使用someProperties
创建 bean 并且名称未被覆盖,以下应该可以正常工作。
@Scheduled(fixedDelayString = "#{@someProperties.getMillis()}")
来自Spring 文档:
当使用配置属性扫描或通过@EnableConfigurationProperties 注册@ConfigurationProperties bean 时,bean 具有常规名称:-,其中是@ConfigurationProperties 注释中指定的环境键前缀,并且是bean 的完全限定名称。 如果注释不提供任何前缀,则仅使用 bean 的完全限定名称。 上面示例中的 bean 名称是 com.example.app-com.example.app.SomeProperties。
所以答案归结为:
@Scheduled(fixedRateString = "#{@'other-com.example.demo.OtherProperties'.millis}")
这是示例项目 - https://github.com/marksutic/scheduler_fixedratestring ,从 Arun Sai 分叉
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.