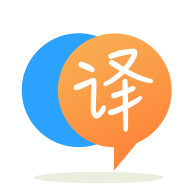
[英]Javascript wait for one animation to finish before the next one begins
[英]How to wait for one javascript to be loaded before loading the next
我想知道如何在加载下一个脚本之前等待加载一个脚本。 例如(并且实际上将另一个页面完全加载到页面中):
<html>
<script src = "script1"></script>
<script src = "script2"></script>
<script>
$(document).ready( function() {
$("#content1").load("index2.html");
});
</script>
</html>
因此,在正确加载 script1(并运行其中的所有内容)后,我想加载 script 2,在加载完之后,我想将文档 index2.html 加载到此 html 文档中。
到目前为止我一直在尝试的是这个,但它并没有像我想要的那样工作。
文件 1:scriptOrderTest.html
<html>
<div id ="content1"></div>
<script src="js/jquery-3.2.1.min.js"></script>
<script>
var variable1 = undefined;
$(document).ready( function() {
$.ajax({
url: "script1.js",
dataType: "script",
success: () => {
$.ajax({
url: "script2.js",
dataType: "script",
success: () =>{
// do the stuff you need
$("#content1").load("index2.html");
}
});
}
});
});
</script>
</html>
文件 2:script1.js
console.log("Starting script 1");
function revealTime(){
console.log("Oh, I just wasted 5 seconds of my life")
}
//This script is just wasting time
setTimeout(revealTime,5000);
文件 3:script2.js
console.log("Starting script 2");
function revealTime(){
console.log("Oh, I just wasted 5 seconds of my life AGAIN") ;
variable1 = "Pizza";
}
//This script is just wasting time
setTimeout(revealTime,5000);
文件 4:index2.html
<html>
<script src="js/jquery-3.2.1.min.js"></script>
<script>
document.write("The variable is:"+variable1);
</script>
</html>
首先,所以目标是等待脚本完成,以便变量在将其写入页面之前可以获得一个值。 上面的代码不是这种情况。
把它放在 document.ready 中:
$.ajax({
url: url1,
dataType: "script",
success: () => {
$.ajax({
url: url2,
dataType: "script",
success: () =>{
// do the stuff you need
}
});
}
});
您可以通过同时使用Promise
和链式操作来解决此问题。
如果您创建一个添加脚本的函数,然后告诉您何时完成。
就像是:
function addScript(url){
return new Promise((resolve, reject) => {
console.log("Download", url);
var script = document.createElement("script")
script.type = "text/javascript";
script.onload = function(){
console.log(`Download done (${url})`);
resolve(1);
};
script.src = url;
document.getElementsByTagName("head")[0].appendChild(script);
});
}
并定义您需要的所有脚本,我只是用 vue 做了一个无用的例子。
const scripts = [
"https://cdnjs.cloudflare.com/ajax/libs/vue/2.5.13/vue.js",
"https://cdnjs.cloudflare.com/ajax/libs/vue-router/3.0.1/vue-router.js"
];
顺序的
您在问题中要求的内容-当时下载,将下一个脚本的下载延迟到第一个脚本完成。
const tasks = scripts[Symbol.iterator]();
function addAllScripts(callback){
const url = tasks.next().value;
if(url){
addScript(url).then( () => { addAllScripts(callback); } );
}else{
callback();
}
};
addAllScripts(() => {
console.log("Ready");
new Vue({
el: '#app',
template: '<p>hello world</p>'
});
});
function addScript(url){ return new Promise((resolve, reject) => { console.log("Download", url); var script = document.createElement("script") script.type = "text/javascript"; script.onload = function(){ console.log(`Download done (${url})`); resolve(1); }; script.src = url; document.getElementsByTagName("head")[0].appendChild(script); }); } const scripts = [ "https://cdnjs.cloudflare.com/ajax/libs/vue/2.5.13/vue.js", "https://cdnjs.cloudflare.com/ajax/libs/vue-router/3.0.1/vue-router.js" ]; const tasks = scripts[Symbol.iterator](); function addAllScripts(callback){ const url = tasks.next().value; if(url){ addScript(url).then( () => { addAllScripts(callback); } ); }else{ callback(); } }; addAllScripts(() => { console.log("Ready"); new Vue({ el: '#app', template: '<p>hello world</p>' }); });
<div id="app"></div>
平行线
最佳解决方案,执行速度更快 - 一次添加所有脚本,并等待所有脚本完成
const tasks = scripts.map(addScript);
Promise.all( tasks )
.then(function(){
console.log("Ready");
new Vue({
el: '#app',
template: '<p>hello world</p>'
});
});
function addScript(url){ return new Promise((resolve, reject) => { console.log("Download", url); var script = document.createElement("script") script.type = "text/javascript"; script.onload = function(){ console.log(`Download done (${url})`); resolve(1); }; script.src = url; document.getElementsByTagName("head")[0].appendChild(script); }); } const scripts = [ "https://cdnjs.cloudflare.com/ajax/libs/vue/2.5.13/vue.js", "https://cdnjs.cloudflare.com/ajax/libs/vue-router/3.0.1/vue-router.js" ]; const tasks = scripts.map(addScript); Promise.all( tasks ) .then(function(){ console.log("Ready"); new Vue({ el: '#app', template: '<p>hello world</p>' }); });
<div id="app"></div>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.