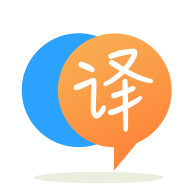
[英]How to run JUnit SpringJUnit4ClassRunner with Parametrized?
[英]How to mock absent bean definitions in SpringJUnit4ClassRunner?
我有一个 Spring 4 JUnit 测试,它应该只验证我的应用程序的特定部分。
@WebAppConfiguration
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration("classpath:context-test.xml")
@ActiveProfiles("test")
public class FooControllerIntegrationTest {
...
}
所以我不想配置和实例化所有那些实际上不涉及我的测试范围的bean。 例如,我不想配置在我不打算在这里测试的另一个控制器中使用的 bean。
但是,因为我不想缩小组件扫描路径,所以出现“No qualifying bean of type”异常:
由以下原因引起:org.springframework.beans.factory.NoSuchBeanDefinitionException:没有 [...
如果我确定它们不涉及我正在测试的功能,有什么办法可以忽略这些遗漏的定义吗?
如果我确定它们不涉及我正在测试的功能,有什么办法可以忽略这些遗漏的定义吗?
不,没有用于此目的的自动或内置机制。
如果您指示 Spring 加载对其他 bean 具有强制依赖性的 bean,则其他 beans 必须存在。
出于测试目的,限制哪些 bean 处于活动状态的范围的最佳实践包括配置的模块化(例如,水平切片允许您有选择地选择加载应用程序的哪些层)和 bean 定义配置文件的使用。
如果您使用的是 Spring Boot,则还可以在 Spring Boot 测试中使用“测试切片”或@MockBean
/ @SpyBean
。
但是,您应该记住,在给定的集成测试中加载您未使用的 bean 通常不是一件坏事,因为您正在(希望)测试其他组件,而这些组件实际上在您的测试中的其他测试类中需要这些 bean套件,然后ApplicationContext
将只加载一次并缓存在不同的集成测试类中。
问候,
Sam( Spring TestContext Framework 的作者)
我找到了一种如何自动模拟缺少的 bean 定义的方法。
核心思想是创建自己的BeanFactory
:
public class AutoMockBeanFactory extends DefaultListableBeanFactory {
@Override
protected Map<String, Object> findAutowireCandidates(final String beanName, final Class<?> requiredType, final DependencyDescriptor descriptor) {
String mockBeanName = Introspector.decapitalize(requiredType.getSimpleName()) + "Mock";
Map<String, Object> autowireCandidates = new HashMap<>();
try {
autowireCandidates = super.findAutowireCandidates(beanName, requiredType, descriptor);
} catch (UnsatisfiedDependencyException e) {
if (e.getCause() != null && e.getCause().getCause() instanceof NoSuchBeanDefinitionException) {
mockBeanName = ((NoSuchBeanDefinitionException) e.getCause().getCause()).getBeanName();
}
this.registerBeanDefinition(mockBeanName, BeanDefinitionBuilder.genericBeanDefinition().getBeanDefinition());
}
if (autowireCandidates.isEmpty()) {
final Object mock = mock(requiredType);
autowireCandidates.put(mockBeanName, mock);
this.addSingleton(mockBeanName, mock);
}
return autowireCandidates;
}
}
它还应该通过基于GenericXmlWebContextLoader
创建自己的AbstractContextLoader
实现来注册。 不幸的是,后者有一个final
的loadContext(MergedContextConfiguration mergedConfig)
方法,因此需要完全复制它的实现(比如到类AutoMockGenericXmlWebContextLoader
),但有一个区别:
GenericWebApplicationContext context = new GenericWebApplicationContext(new AutoMockBeanFactory());
不可以在测试中使用:
@ContextConfiguration(
value = "classpath:context-test.xml",
loader = AutoMockGenericXmlWebContextLoader.class)
如果你不缩小你的组件扫描范围,那么通常你会拥有所有可用于测试的bean,除了一些有条件可用的特定bean(例如由spring-batch定义的bean)
在这种情况下,对我有用的一个选项是将此类依赖项和组件标记为@Lazy
。 这将确保它们只在需要时加载。 请注意(取决于场景)您可能必须将@Autowired
依赖项和@Component
标记为@Lazy
就像发布的 OP 一样,这里是相当于注入任何模拟缺失 bean 的注释上下文:
context = new CustomAnnotationConfigApplicationContext(SpringDataJpaConfig.class);
public class CustomAnnotationConfigApplicationContext extends AnnotationConfigApplicationContext {
public CustomAnnotationConfigApplicationContext() {
super(new AutoMockBeanFactory());
}
public CustomAnnotationConfigApplicationContext(Class<?>... annotatedClasses) {
this();
this.register(annotatedClasses);
this.refresh();
}
}
public class AutoMockBeanFactory extends DefaultListableBeanFactory {
@Override
protected Map<String, Object> findAutowireCandidates(final String beanName, final Class<?> requiredType, final DependencyDescriptor descriptor) {
String mockBeanName = Introspector.decapitalize(requiredType.getSimpleName());
Map<String, Object> autowireCandidates = new HashMap<>();
try {
autowireCandidates = super.findAutowireCandidates(beanName, requiredType, descriptor);
} catch (UnsatisfiedDependencyException e) {
if (e.getCause() != null && e.getCause().getCause() instanceof NoSuchBeanDefinitionException) {
mockBeanName = ((NoSuchBeanDefinitionException) e.getCause().getCause()).getBeanName();
}
this.registerBeanDefinition(mockBeanName, BeanDefinitionBuilder.genericBeanDefinition().getBeanDefinition());
}
if (autowireCandidates.isEmpty()) {
System.out.println("Mocking bean: " + mockBeanName);
final Object mock = Mockito.mock(requiredType);
autowireCandidates.put(mockBeanName, mock);
this.addSingleton(mockBeanName, mock);
}
return autowireCandidates;
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.