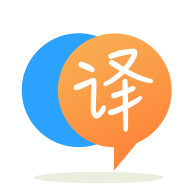
[英]Exceptions while integrating : java.security.InvalidKeyException: Illegal key size
[英]Java Cryptography throwing Exception while initializing Cipher: java.security.InvalidKeyException: Illegal key size
我正在尝试使用“ AES / GCM / NoPadding”对一个简单的测试“ sometext”进行加密 。
我有一个主要方法,在该方法中,我首先要传递一个字符串作为应该被加密的参数。 加密的文本将出现在控制台上。 然后,随后调用解密(通过传递加密的文本)来检查解密是否工作正常,即,解密后是否恢复了相同的文本。 我正在调用主类,并且它在我的getCipher()
自定义方法中失败,但异常:
Exception in thread "main" my.package.EncryptionException: java.security.InvalidKeyException: Illegal key size
at my.package.Encryptor.encrypt(Encryptor.java:76)
at my.package.Encryptor.encrypt(Encryptor.java:61)
at my.package.Encryptor.main(Encryptor.java:151)
Caused by: java.security.InvalidKeyException: Illegal key size
at javax.crypto.Cipher.checkCryptoPerm(Cipher.java:1039)
at javax.crypto.Cipher.implInit(Cipher.java:805)
at javax.crypto.Cipher.chooseProvider(Cipher.java:864)
at javax.crypto.Cipher.init(Cipher.java:1396)
at javax.crypto.Cipher.init(Cipher.java:1327)
at my.package.Encryptor.getCipher(Encryptor.java:134)
at my.package.Encryptor.encrypt(Encryptor.java:69)
... 2 more
我不确定为什么此非法密钥大小会产生错误。 我的密钥“ SecREtKeY”在16个字符内。
这是下面的代码:
public class Encryptor {
private static final String ALGORITHM_AES256 = "AES/GCM/NoPadding";
private static final int IV_LEN = 16;
private static final int KEY_LEN = 32;
private final SecureRandom random;
private final byte[] inputKey;
private final SecretKeySpec secretKeySpec;
private final Cipher cipher;
Encryptor() throws EncryptionException
{
String secretKey = "SecREtKeY";
byte[] key = secretKey.getBytes(StandardCharsets.UTF_8);
if (key == null) {
throw new EncryptionException("Null Key");
}
inputKey = new byte[key.length];
System.arraycopy(key, 0, inputKey, 0, inputKey.length);
byte[] aesKey = trimKey(key);
System.out.println("aesKey.length: "+aesKey.length);
try
{
random = SecureRandom.getInstance("SHA1PRNG");
this.secretKeySpec = new SecretKeySpec(aesKey, "AES");
this.cipher = Cipher.getInstance(ALGORITHM_AES256);
} catch (NoSuchAlgorithmException | NoSuchPaddingException e) {
throw new EncryptionException(e);
}
}
/*
* Encrypts plaint text. Returns Encrypted String.
*/
String encrypt(String plaintext) throws EncryptionException
{
return encrypt(plaintext.getBytes(StandardCharsets.UTF_8));
}
String encrypt(byte[] plaintext) throws EncryptionException
{
try
{
byte[] iv = getRandom(IV_LEN);
Cipher msgcipher = getCipher(Cipher.ENCRYPT_MODE, iv);
byte[] encryptedTextBytes = msgcipher.doFinal(plaintext);
byte[] payload = concat(iv, encryptedTextBytes);
return Base64.getEncoder().encodeToString(payload);
}
catch (BadPaddingException | InvalidKeyException | IllegalBlockSizeException | InvalidAlgorithmParameterException e){
throw new EncryptionException(e);
}
}
/*
* Decrypts plaint text. Returns decrypted String.
*/
String decrypt(String ciphertext) throws EncryptionException
{
return decrypt(Base64.getDecoder().decode(ciphertext));
}
String decrypt(byte[] cipherBytes) throws EncryptionException
{
byte[] iv = Arrays.copyOf(cipherBytes, IV_LEN);
byte[] cipherText = Arrays.copyOfRange(cipherBytes, IV_LEN, cipherBytes.length);
try {
Cipher cipher = getCipher(Cipher.DECRYPT_MODE, iv);
byte[] decrypt = cipher.doFinal(cipherText);
return new String(decrypt, StandardCharsets.UTF_8);
} catch (BadPaddingException | InvalidKeyException | IllegalBlockSizeException | InvalidAlgorithmParameterException e) {
throw new EncryptionException(e);
}
}
private byte[] trimKey(byte[] key)
{
byte[] outkey = new byte[KEY_LEN];
if(key.length >= KEY_LEN) {
System.arraycopy(key, key.length - KEY_LEN, outkey, 0, KEY_LEN);
}
else {
System.arraycopy(key, 0, outkey, 0, key.length);
}
return outkey;
}
private byte[] getRandom(int size)
{
byte[] data = new byte[size];
random.nextBytes(data);
return data;
}
private byte[] concat(byte[] first, byte[] second)
{
byte[] result = Arrays.copyOf(first, first.length + second.length);
System.arraycopy(second, 0, result, first.length, second.length);
return result;
}
private Cipher getCipher(int encryptMode, byte[] iv) throws InvalidKeyException, InvalidAlgorithmParameterException
{
GCMParameterSpec gcmSpec = new GCMParameterSpec(IV_LEN * 8, iv);
cipher.init(encryptMode, getSecretKeySpec(), gcmSpec);
return cipher;
}
private SecretKeySpec getSecretKeySpec() {
return secretKeySpec;
}
public static void main(String[] args) throws Exception
{
if(args.length == 1) {
String plainText = args[0];
Encryptor aes = new Encryptor();
String encryptedString = aes.encrypt(plainText);
System.out.println(plainText + ":" + encryptedString);
String decryptedString = aes.decrypt(encryptedString);
System.out.println(encryptedString+":"+decryptedString);
}
else {
System.out.println("USAGE: java my.package.Encryptor [text to encrypt]");
}
}
}
知道为什么我会收到此错误吗?
java.security.InvalidKeyException: Illegal key size
表示您尚未安装JCE(Java密码学扩展)。 AES256需要它
对于Java 8,您可以在此处下载它: http : //www.oracle.com/technetwork/java/javase/downloads/jce8-download-2133166.html
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.