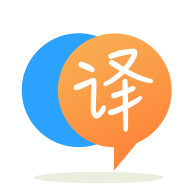
[英]What is causing segmentation fault in append Node function in doubly linked list?
[英]C: Segmentation fault in doubly linked list, in append and delete function
我在C中创建双向链接列表时遇到问题,因为它在添加和删除中返回分段错误。 好像我将其中一个指针指向了错误的位置,但是我很难找到它。
我所讨论的功能如下:
附加:
int list_append(list_t *list, int val) {
if(!list) { return 1; }
// create a new node, then assign the value
struct node_t *new = (node_t *) malloc(sizeof(node_t));
new->val = val;
new->prev = NULL;
new->next = NULL;
// place the new node (since it is tail, make next null)
new->next = NULL;
// if there's no head(null), make the new node the head (and tail)
if (list->head == NULL)
{
list->head = new;
}
// if the next value is not null, traverse until it is (=tail)
struct node_t *node = list->head;
while (node)
{
node = list->tail;
}
// place the new node at the next of tail
list->tail->next = new;
// set the old tail as the previous of new node
new->prev = list->tail;
list->tail->next = NULL;
CHECK_LIST(list);
return 0;
}
删除 :
int list_delete(list_t *list, int val) {
if(!list) { return 1; }
CHECK_LIST(list);
// make a temporary node to help find the value
struct node_t *temp = list->head;
// start traversing the list
while (temp)
{
// if match is found
if (temp->val == val)
{
// if the match is actually the head, make the next node the new head,
// then make old head null
if (temp == list->head)
{
list->head = list->head->next;
list->head->prev = NULL;
}
// if the match is at the tail, make the previous node the new tail,
// then make the old tail null
if (temp == list->tail)
{
list->tail = list->tail->prev;
temp->prev->next = NULL;
} else
{
// set new next of temp as the old next of temp, and vice versa
// then, free temp from memory
temp->next = temp->prev->next;
temp->prev = temp->next->prev;
temp = NULL;
free(temp);
}
}
// keep moving
temp = temp->next;
}
CHECK_LIST(list);
return 0;
}
谢谢您的帮助。
下面的append()
函数的代码块中有一个错误
while (node) {
node = list->tail; /*what is tail here ?? */
}
/* after this loop you are not using node anywhere, what's the use
of iterating through loop */
// place the new node at the next of tail
list->tail->next = new;
// set the old tail as the previous of new node
new->prev = list->tail;
list->tail->next = NULL;
用。。。来代替
struct node_t *node = list->head;
while (node->next) {/* when loop fails node holds last node address */
node = list->next;
}
node->next = new; /* replace node->next as new node */
new->prev = node->next; /* new node previous make it as old node next */
new->next = NULL;/* last node next make it as 0*/
最好先运行gdb
并执行bt
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.