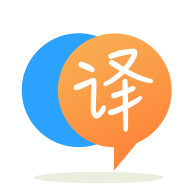
[英]Firestore expressjs: how to get document where specific field exists and update the document
[英]How do I get documents where a specific field exists/does not exists in Firebase Cloud Firestore?
在 Firebase Cloud Firestore 中,我在集合中有“user_goals”,目标可能是预定义的目标(master_id:“XXXX”)或自定义目标(没有“master_id”键)
在 JavaScript 中,我需要编写两个函数,一个用于获取所有预定义目标,另一个用于获取所有自定义目标。
我有一些解决方法可以通过将“master_id”设置为“”空字符串来获得自定义目标,并且能够获得如下:
db.collection('user_goals')
.where('challenge_id', '==', '') // workaround works
.get()
仍然这不是正确的方法,我继续将其用于具有“master_id”的预定义目标,如下所示
db.collection('user_goals')
.where('challenge_id', '<', '') // this workaround
.where('challenge_id', '>', '') // is not working
.get()
由于 Firestore 没有“!=”运算符,我需要使用“<”和“>”运算符但仍然没有成功。
问题:忽略这些变通方法,通过检查特定字段是否存在来获取文档的首选方法是什么?
作为@Emile Moureau 解决方案。 我更喜欢
.orderBy(`field`)
用字段来查询文档是否存在。 因为它可以处理任何类型的数据,即使是null
也是如此。
但正如@Doug Stevenson 所说:
您无法查询 Firestore 中不存在的内容。 字段需要存在才能让 Firestore 索引知道它。
您无法查询没有该字段的文档。 至少现在。
获取存在指定字段的文档的首选方法是使用:
.orderBy(fieldPath)
如Firebase 文档中所述:
因此@hisoft 提供的答案是有效的。 我只是决定提供官方来源,因为问题是针对首选方式。
我使用的解决方案是:
使用: .where('field', '>', ''),
其中“字段”是我们正在寻找的字段!
Firestore 是一个索引数据库。 对于文档中的每个字段,该文档会根据您的配置适当地插入到该字段的索引中。 如果文档不包含特定字段(如challenge_id
),它将不会出现在该字段的索引中,并且将从对该字段的查询中省略。 通常,由于 Firestore 的设计方式,查询应该在一次连续扫描中读取索引。 在引入!=
和not-in
运算符之前,这意味着您不能排除特定值,因为这需要跳过索引的各个部分。 尝试在单个查询中使用独占范围 ( v<2 || v>4
) 时,仍然会遇到此限制。
字段值根据实时数据库排序顺序进行排序,但在遇到重复时,结果可以按多个字段排序,而不仅仅是文档的 ID。
Firestore 值排序顺序
优先 | 排序值 | 优先 | 排序值 |
---|---|---|---|
1 | null |
6 | 字符串 |
2 | false |
7 | 文件参考 |
3 | true |
8 | 地理点 |
4 | 数字 | 9 | 数组 |
5 | 时间戳 | 10 | 地图 |
!=
/ <>
本节记录了在2020 年 9 月发布!=
和not-in
运算符之前不等式是如何工作的。 请参阅有关如何使用这些运算符的文档。 以下部分将留作历史用途。
要在 Firestore 上执行不等式查询,您必须重新编写查询,以便可以通过读取 Firestore 的索引来读取它。 对于不等式,这是通过使用两个查询来完成的——一个用于小于equality
的值,另一个用于大于等于的值。
作为一个简单的例子,假设我想要不等于 3 的数字。
const someNumbersThatAreNotThree = someNumbers.filter(n => n !== 3)
可以写成
const someNumbersThatAreNotThree = [
...someNumbers.filter(n => n < 3),
...someNumbers.filter(n => n > 3)
];
将此应用于 Firestore,您可以转换此( 以前)不正确的查询:
const docsWithChallengeID = await colRef
.where('challenge_id', '!=', '')
.get()
.then(querySnapshot => querySnapshot.docs);
进入这两个查询并合并它们的结果:
const docsWithChallengeID = await Promise.all([
colRef
.orderBy('challenge_id')
.endBefore('')
.get()
.then(querySnapshot => querySnapshot.docs),
colRef
.orderBy('challenge_id')
.startAfter('')
.get()
.then(querySnapshot => querySnapshot.docs),
]).then(results => results.flat());
重要提示:请求用户必须能够读取与查询匹配的所有文档,以免出现权限错误。
简而言之,在 Firestore 中,如果某个字段未出现在文档中,则该文档将不会出现在该字段的索引中。 这与实时数据库形成对比,其中省略字段的值为null
。
由于 NoSQL 数据库的性质,您正在使用的架构可能会发生变化,从而使您的旧文档缺少字段,您可能需要一个解决方案来“修补您的数据库”。 为此,您将遍历您的集合并将新字段添加到缺少它的文档中。
为避免权限错误,最好使用带有服务帐户的 Admin SDK 进行这些调整,但您可以使用对数据库具有适当读/写访问权限的用户使用常规 SDK 来执行此操作。
此函数是递归的,旨在执行一次。
async function addDefaultValueForField(queryRef, fieldName, defaultFieldValue, pageSize = 100) {
let checkedCount = 0, pageCount = 1;
const initFieldPromises = [], newData = { [fieldName]: defaultFieldValue };
// get first page of results
console.log(`Fetching page ${pageCount}...`);
let querySnapshot = await queryRef
.limit(pageSize)
.get();
// while page has data, parse documents
while (!querySnapshot.empty) {
// for fetching the next page
let lastSnapshot = undefined;
// for each document in this page, add the field as needed
querySnapshot.forEach(doc => {
if (doc.get(fieldName) === undefined) {
const addFieldPromise = doc.ref.update(newData)
.then(
() => ({ success: true, ref: doc.ref }),
(error) => ({ success: false, ref: doc.ref, error }) // trap errors for later analysis
);
initFieldPromises.push(addFieldPromise);
}
lastSnapshot = doc;
});
checkedCount += querySnapshot.size;
pageCount++;
// fetch next page of results
console.log(`Fetching page ${pageCount}... (${checkedCount} documents checked so far, ${initFieldPromises.length} need initialization)`);
querySnapshot = await queryRef
.limit(pageSize)
.startAfter(lastSnapshot)
.get();
}
console.log(`Finished searching documents. Waiting for writes to complete...`);
// wait for all writes to resolve
const initFieldResults = await Promise.all(initFieldPromises);
console.log(`Finished`);
// count & sort results
let initializedCount = 0, errored = [];
initFieldResults.forEach((res) => {
if (res.success) {
initializedCount++;
} else {
errored.push(res);
}
});
const results = {
attemptedCount: initFieldResults.length,
checkedCount,
errored,
erroredCount: errored.length,
initializedCount
};
console.log([
`From ${results.checkedCount} documents, ${results.attemptedCount} needed the "${fieldName}" field added.`,
results.attemptedCount == 0
? ""
: ` ${results.initializedCount} were successfully updated and ${results.erroredCount} failed.`
].join(""));
const errorCountByCode = errored.reduce((counters, result) => {
const code = result.error.code || "unknown";
counters[code] = (counters[code] || 0) + 1;
return counters;
}, {});
console.log("Errors by reported code:", errorCountByCode);
return results;
}
然后,您将使用以下方法应用更改:
const goalsQuery = firebase.firestore()
.collection("user_goals");
addDefaultValueForField(goalsQuery, "challenge_id", "")
.catch((err) => console.error("failed to patch collection with new default value", err));
也可以调整上述函数以允许根据文档的其他字段计算默认值:
let getUpdateData;
if (typeof defaultFieldValue === "function") {
getUpdateData = (doc) => ({ [fieldName]: defaultFieldValue(doc) });
} else {
const updateData = { [fieldName]: defaultFieldValue };
getUpdateData = () => updateData;
}
/* ... later ... */
const addFieldPromise = doc.ref.update(getUpdateData(doc))
正如您正确指出的那样,无法根据!=
进行过滤。 如果可能,我会添加一个额外的字段来定义目标类型。 可以在安全规则中使用!=
以及各种字符串比较方法,因此您可以根据您的challenge_id
格式强制执行正确的目标类型。
创建一个type
字段并基于此字段进行过滤。
type: master
或type: custom
并搜索.where('type', '==', 'master')
或搜索 custom。
创建一个可以为true
或false
的customGoal
字段。
customGoal: true
并搜索.where('customGoal', '==', true)
或 false(根据需要)。
现在可以在 Cloud Firestore 中执行 != 查询
Firestore 确实接受了布尔值,这是一回事! 并且可以orderBy
'd。
所以经常,就像现在一样,为此,我将它添加到来自onSnapshot
或get
的数组推送中,使用.get().then(
用于开发...
if (this.props.auth !== undefined) {
if (community && community.place_name) {
const sc =
community.place_name && community.place_name.split(",")[1];
const splitComma = sc ? sc : false
if (community.splitComma !== splitComma) {
firebase
.firestore()
.collection("communities")
.doc(community.id)
.update({ splitComma });
}
const sc2 =
community.place_name && community.place_name.split(",")[2];
const splitComma2 =sc2 ? sc2 : false
console.log(splitComma2);
if (community.splitComma2 !== splitComma2) {
firebase
.firestore()
.collection("communities")
.doc(community.id)
.update({
splitComma2
});
}
}
这样,我可以使用orderBy
而不是where
browseCommunities = (paginate, cities) => {
const collection = firebase.firestore().collection("communities");
const query =
cities === 1 //countries
? collection.where("splitComma2", "==", false) //without a second comma
: cities //cities
? collection
.where("splitComma2", ">", "")
.orderBy("splitComma2", "desc") //has at least two
: collection.orderBy("members", "desc");
var shot = null;
if (!paginate) {
shot = query.limit(10);
} else if (paginate === "undo") {
shot = query.startAfter(this.state.undoCommunity).limit(10);
} else if (paginate === "last") {
shot = query.endBefore(this.state.lastCommunity).limitToLast(10);
}
shot &&
shot.onSnapshot(
(querySnapshot) => {
let p = 0;
let browsedCommunities = [];
if (querySnapshot.empty) {
this.setState({
[nuller]: null
});
}
querySnapshot.docs.forEach((doc) => {
p++;
if (doc.exists) {
var community = doc.data();
community.id = doc.id;
这不是一个理想的解决方案,但是当字段不存在时,这是我的解决方法:
let user_goals = await db.collection('user_goals').get()
user_goals.forEach(goal => {
let data = goal.data()
if(!Object.keys(data).includes(challenge_id)){
//Perform your task here
}
})
请注意,它会极大地影响您的读取计数,因此只有在您的收藏量较小或负担得起读取时才使用它。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.