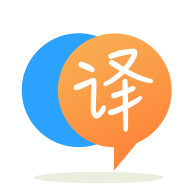
[英]Java: Most efficient way to map properties of one list of objects to another
[英]Java - map object properties in one list to another list of objects
我有以下两个列表,其中包含具有以下属性的对象。 数据已包含在示例中。
清单1-ShopOwnerMapList
+------------+-------------+
| Owner | ShopId |
+------------+-------------+
| Jack | 67 |
| Sarah | 69 |
| Sarah | B7 |
| Tom | 83 |
| Harry | 20 |
+------------+-------------+
ShopOwner与ShopIds是“一对多”的关系。 一个店主可以有多个ID。
清单2-ShopOwnerList
+------------+------+--------+
| Owner | Age | ShopId |
+------------+------+--------+
| Jack | 32 | NULL |
| Sarah | 30 | NULL |
| Tom | 45 | NULL |
| Harry | 55 | NULL |
+------------+------+--------+
ShopOwnerList具有一个称为ShopId的属性,默认情况下为NULL
。
现在如何使用所有者作为种类的“键”将ShopOwnerMapList映射到ShopOwnerList? 如果ShopOwnerMapList中存在两个Owner实例(与Sarah一样),那么我希望ShopOwnerList中的对应项重复。 因此,实际上,合并之后我将得到:
+------------+------+--------+
| Owner | Age | ShopId |
+------------+------+--------+
| Jack | 32 | 67 |
| Sarah | 30 | 69 |
| Sarah | 30 | B7 |
| Tom | 45 | 83 |
| Harry | 55 | 20 |
+------------+------+--------+
如何在Java中完成此操作?
我尝试了以下操作,但是在存在两个所有者实例的情况下,它不会扩展ShopOwnerList:
for (int i = 0; i < shopOwnerMapList.size(); i++) {
for (int j = 0; j < shopOwnerList.size(); j++) {
ShopOwnerMap shopOwnerMap = shopOwnerMapList.get(i);
ShopOwner shopOwner = shopOwnerList.get(j);
if(shopOwnerMap.getOwner().equals(shopOwner.getOwner()) {
if(shopOwner.getShopId() == null) {
shopOwner.setShopId(shopOwnerMap.getShopId());
}
}
}
}
类
public class ShopOwnerMap {
String Owner;
String ShopId;
public MyClass() {
}
public String getOwner() {
return this.Owner;
}
public void setOwner(String value) {
this.Owner = value;
}
public String getShopId() {
return this.ShopId;
}
public void setShopId(String value) {
this.ShopId = value;
}
}
public class ShopOwner {
String Owner;
String ShopId;
Integer Age;
String DateOfBirth;
public ShopOwner() {
}
public String getOwner() {
return this.Owner;
}
public void setOwner(String value) {
this.Owner = value;
}
public String getShopId() {
return this.ShopId;
}
public void setShopId(String value) {
this.ShopId = value;
}
public Integer getAge() {
return this.Age;
}
public void setAge(Integer value) {
this.Age = value;
}
public String getDateOfBirth() {
return this.DateOfBirth;
}
public void setDateOfBirth(String value) {
this.DateOfBirth = value;
}
}
正如我在评论中所说,建议您重新考虑一下模型。 首先介绍一个Shop
类:
public class Shop {
private final String id; // Id of this shop.
private final ShopOwner owner; // Owner of this shop.
public Shop(String id, ShopOwner owner) {
this.id = id;
this.owner = owner;
}
public String getId() { return id; }
// Overwrite equals and hashcode to allow using Shop as a key in a HashMap (only necessary if you want to enforce uniqueness of a ShopOwner's shops by maintaining a ShopOwner's owned shops using a Map).
// We simply define equality based on id equality and rely on String.hashCode() (note that this will have to be changed if you want to add additional properties and define equality based on those):
@Override
public boolean equals(Object o) {
return o instanceof Shop && o.toString().equals(this.toString());
}
@Override
public int hashCode() {
return toString().hashCode();
}
@Override
public String toString() {
return id;
}
// Other properties etc.
}
更改ShopOwner
以保留此ShopOwner
拥有的商店的List
:
public class ShopOwner {
String owner;
List<Shop> shops = new ArrayList<>(); // Shops owned by this ShopOwner.
int age;
String dateOfBirth;
public void addShop(Shop s) {
// Note that this does NOT guarantee uniqueness of shops if your DB query returns multiple instances of the same shop.
// If you want this, you could use a Map instead.
this.shops.add(s);
}
// Your other getters etc.
}
根据您的评论,由于与数据库的交互方式,您希望保留ShopOwnerMap
(我个人将其删除,而仅实例化虚拟对象(临时;将owner
字段设置为null
))将Shop
s和其各自的虚拟对象(请阅读:临时的-稍后将由在您的匹配代码期间找到的“真实”(已经存在) ShopOwner
实例替换) ShopOwner
立即添加,但这需要更多了解导致此问题的代码的知识。 现在要做的是将List<ShopOwnerMap>
与List<ShopOwner>
匹配,并将Shop
实例添加到List<ShopOwner>
中的每个ShopOwner
中,如下所示:
List<ShopOwnerMap> soms = ...; // the list you produced from the DB query.
List<ShopOwner> sos = ...; // your list of ShopOwners, each of which needs to be initialized with the shops they own, i.e. their initial lists of shops are empty.
// Note that the below could be reduced from O(N^2) to O(N) if you manage your ShopOwners using a Map (using an equals+hashCode scheme as suggested for Shop).
for (ShopOwnerMap som : soms) {
for (ShopOwner so : sos) {
// We assume that the 'owner' property (perhaps 'name' is more suitable?) is unique among ShopOwners.
if (som.getOwner().equals(so.getOwner()) {
// Found the corresponding shop owner; create shop instance and add to list of owned shops.
so.addShop(new Shop(som.getShopId(), so));
}
}
}
免责声明:我很累并且有点急于执行此操作,因此它可能不是100%正确的,但是它应该为您提供总体思路。 另外,如上面括号中的注释所示,该提议的设计也不是完美的。 那将需要对您的数据模型有更多的了解。
我建议使用番石榴的Multimap。 您可以使用键将多个值插入多图的单个位置。 要实施您的解决方案,您实际上需要两张地图。 一个名为ShopOwnerMultimap的Multimap,它包含ShopOwnerMapList中的数据;还有一个称为ShopOwnerMap的常规Map,它包含ShopOwnerList中的数据。 两张地图都将使用所有者名称作为密钥。 执行映射时,请使用ShopOwnerMap中的键集来获取ShopOwnerMultimap中的值。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.