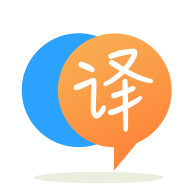
[英]How do I post the row id in a custom built ASP.NET Core Razor Pages editable Grid?
[英]How do I post an IFormFile in ASP.Net Core 2.0 Razor Pages?
我正在使用带有ASP.Net Core 2.0和Razor Pages的应用程序。 我一直在关注有关如何将文件上传到Azure blob存储的Microsoft文档,但到目前为止还无法正常工作。
我有两个单独的模型类。 一种用于文件上传,另一种用于Word。
文件上传类:
public class WordUpload
{
public IFormFile SoundFile { get; set; }
public IFormFile SoundFileSentence { get; set; }
}
其他类:
public class Word
{
public int ID { get; set; }
public string Answer { get; set; }
public string AlternativeAnswer { get; set; }
public string Hint { get; set; }
public int Length { get; set; }
public int Vowels { get; set; }
public string Language { get; set; }
public string Category { get; set; }
public int Module { get; set; }
public string Difficulty { get; set; }
public string Sound { get; set; }
public string SoundSentence { get; set; }
}
未传递WordUpload.SoundFile的页面。 这是问题所在,因为它始终返回null。
public class CreateModel : PageModel
{
private readonly Data.ApplicationDbContext _context;
private readonly IWordRepository _wordRepository;
public CreateModel(Data.ApplicationDbContext context, IWordRepository wordRepository)
{
_context = context;
_wordRepository = wordRepository;
}
/// <summary>
/// OnGet triggers when the page is opened
/// </summary>
/// <returns></returns>
public IActionResult OnGet()
{
Word = new Word
{
Answer = "",
AlternativeAnswer = "",
Hint = "Hint",
Length = 0,
Vowels = 0,
Language = "DK",
Category = "",
Module = 0,
Difficulty = "",
Sound = "",
SoundSentence = ""
};
return Page();
}
[BindProperty]
public WordUpload WordUpload { get; set; }
[BindProperty]
public Word Word { get; set; }
/// <summary>
/// Posts the data to the database async
/// </summary>
/// <returns></returns>
public async Task<IActionResult> OnPostAsync()
{
if (!ModelState.IsValid)
{
return Page();
}
// Get the number of vowels in the word
Word.Vowels = _wordRepository.NumberOfVowels(Word.Answer);
// Save the length
Word.Length = Word.Answer.Length;
// upload file to blob storage
Word.Sound = _wordRepository.UploadAudio(WordUpload.SoundFile);
// save to db
_context.Word.Add(Word);
await _context.SaveChangesAsync();
return RedirectToPage("./Index");
}
}
和视图-页面:
@page
@model Fabetio.Web.Pages.Words.CreateModel
@{
ViewData["Title"] = "Create";
}
<div class="container-fluid darkblue-background">
<br>
<br>
<div class="row">
<div class="col-md-6 col-md-offset-3">
<div class="task--card">
<div class="dasboard--title">
<h1>@ViewData["Title"]</h1>
<p class="subtitle">Subtitle.</p>
</div>
<form method="post">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<input type="hidden" asp-for="Word.ID" />
<div class="form-group">
<label asp-for="Word.Answer" class="control-label"></label>
<input asp-for="Word.Answer" class="form-control" />
<span asp-validation-for="Word.Answer" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Word.AlternativeAnswer" class="control-label"></label>
<input asp-for="Word.AlternativeAnswer" class="form-control" />
<span asp-validation-for="Word.AlternativeAnswer" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Word.Hint" class="control-label"></label>
<input asp-for="Word.Hint" class="form-control" />
<span asp-validation-for="Word.Hint" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Word.Language" class="control-label"></label>
<input asp-for="Word.Language" class="form-control" />
<span asp-validation-for="Word.Language" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Word.Category" class="control-label"></label>
<input asp-for="Word.Category" class="form-control" />
<span asp-validation-for="Word.Category" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Word.Module" class="control-label"></label>
<input asp-for="Word.Module" class="form-control" />
<span asp-validation-for="Word.Module" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Word.Difficulty" class="control-label"></label>
<input asp-for="Word.Difficulty" class="form-control" />
<span asp-validation-for="Word.Difficulty" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="WordUpload.SoundFile" class="control-label"></label>
<input asp-for="WordUpload.SoundFile" type="file" class="form-control" />
<span asp-validation-for="WordUpload.SoundFile" class="text-danger"></span>
</div>
<br>
<br>
<div class="form-group">
<input type="submit" value="Create" class="btn btn--blue" />
</div>
</form>
</div>
</div>
</div>
<br>
<br>
</div>
@section Scripts {
@{await Html.RenderPartialAsync("_ValidationScriptsPartial");}
}
WordUpload.SoundFile不随模型传递。 当我调试应用程序时,它在控制器/页面中返回为null。 所有其他属性都可以毫无问题地传递。
你知道如何传递文件吗?
您需要在表单上添加以下内容:
<form method="post" enctype="multipart/form-data">
在您的post方法中,如果您对模型绑定有任何麻烦,无法将文件绑定到模型,则可以直接从Form.Files获取它,如下所示:
var formFile = HttpContext.Request.Form.Files[0];
您应该先检查HttpContext.Request.Form.Files.Length> 0,否则该代码将引发错误。 然后将字节复制到模型中进行保存。 另请参阅文档
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.