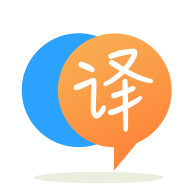
[英]How to Download file in internal Storage using Volley in Android Studio?
[英]how to download pdf form a url, to internal storage and open it : Android studio using kotlin
class Eco9 : AppCompatActivity() {
lateinit internal var uri: Uri
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_eco9)
this.getActionBar();
this.supportActionBar!!.title = "Class 9th Economics";
fab.setOnClickListener { view ->
Snackbar.make(view, "Replace with your own action", Snackbar.LENGTH_LONG)
.setAction("Action", null).show()
}
supportActionBar?.setDisplayHomeAsUpEnabled(true)
val openActivityDownload: Button = findViewById(R.id.eco9ch1)
openActivityDownload.setOnClickListener {
val s = "https://drive.google.com/file/d/0B71LXrqWr0mFUTk5WnVyVEQ3MFE/export?format=pdf"
val fname = "123.pdf"
if (FileExists(fname)) { previewpdf(fname) }
else { download(s)
Toast.makeText(applicationContext, "File will download", Toast.LENGTH_LONG).show()
}
}
}
fun FileExists(name: String): Boolean {
val file = File(Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DOWNLOADS).path + separator + name)
return file.exists()
}
private fun download(s: String) {
val downloadManager = getSystemService(Context.DOWNLOAD_SERVICE) as DownloadManager
val uri = Uri.parse(s)
val request = DownloadManager.Request(uri)
val nameOfFile = URLUtil.guessFileName(uri.toString(), null, MimeTypeMap.getFileExtensionFromUrl(uri.toString()))
val destinationInExternalPublicDir = request.setDestinationInExternalPublicDir(Environment.DIRECTORY_DOWNLOADS)
request.setAllowedOverMetered(true)
request.setAllowedOverRoaming(true)
request.setNotificationVisibility(DownloadManager.Request.VISIBILITY_VISIBLE)
request.allowScanningByMediaScanner()
downloadManager.enqueue(request)
}
private fun previewpdf(name: String) {
val file = File(Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DOWNLOADS).path + separator + name)
val path = fromFile(file)
intent.addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION)
val intent = Intent(Intent.ACTION_VIEW, path)
val chooser = Intent.createChooser(intent, "Open with")
if (intent.resolveActivity(packageManager) != null)
startActivity(chooser)
else
Toast.makeText(applicationContext, "No suitable application to open file", Toast.LENGTH_LONG).show()
}
}
我想从给定的URL下载pdf,按下名为“ echo9ch1”的按钮,如果内部公共下载文件夹中不存在文件,则调用函数download(s),否则,应打开pdf ,无需再次下载。 我在哪里想念? 我可以打开先前下载的123.pdf。 但是我删除了pdf文件, 下载功能现在无法使用 。 其实一个小问题就来了,因为我是用代码打在下载function.Help我下载()函数,并看到预览()和FILEEXISTS()函数也。 我也在manifest.xml中添加了权限。 我也想下载没有wifi的pdf文件。
清单权限为:
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
要下载,您必须使用异步任务。
像本例中一样,我正在下载mutliple文件,它可以是PDF,Image等
class DownloadFileAsync(paths: Array<String?>, listener: AsyncResponse?, size: Int) : AsyncTask<String, String, Array<String?>>() {
private val listener: AsyncResponse? = listener
var current = 0
var paths: Array<String?>
val downPaths = arrayOfNulls<String>(size)
lateinit var fpath: String
var show = false
init {
this.paths = paths
}
protected override fun onPreExecute() {
super.onPreExecute()
}
protected override fun doInBackground(vararg aurl: String): Array<String?> {
val rows = aurl.size
while (current < rows) {
var count: Int
try {
println("Current: " + current + "\t\tRows: " + rows)
fpath = getFileName(this.paths[current]!!)
val url = URL(this.paths[current])
val conexion = url.openConnection()
conexion.connect()
val lenghtOfFile = conexion.getContentLength()
val input = BufferedInputStream(url.openStream(), 512)
val file = File(Environment.getExternalStorageDirectory().path.plus(File.separator).plus(fpath))
downPaths.set(current, file.absolutePath)
if (!file.exists()) file.createNewFile()
val output = FileOutputStream(file)
val data = ByteArray(512)
var total: Long = 0
while (true) {
count = input.read(data)
if (count == -1) break
total += count
output.write(data, 0, count)
}
show = true
output.flush()
output.close()
input.close()
current++
} catch (e: Exception) {
Log.d("Exception", "" + e)
}
} // while end
onPostExecute(downPaths)
return downPaths
}
override fun onProgressUpdate(progress: Array<String?>) {
}
override fun onPostExecute(result: Array<String?>) {
listener?.processFinish(result)
}
private fun getFileName(wholePath: String): String {
var name: String? = null
val start: Int
val end: Int
start = wholePath.lastIndexOf('/')
end = wholePath.length //lastIndexOf('.');
name = wholePath.substring((start + 1), end)
return name
}
}
之后,我通过接口侦听器获取文件路径的回调?.processFinish(result)
注意:也许我应该为此使用注释部分,但是我的声誉仍然很低,所以...
我尝试运行您的代码,唯一缺少的是下载功能。 它应该是:
val destinationInExternalPublicDir = request.setDestinationInExternalPublicDir(Environment.DIRECTORY_DOWNLOADS, nameOfFile)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.