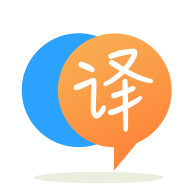
[英]How to upload an image to the ASP.NET CORE WEB API in BYTE ARRAY format using React JS, 400 bad request
[英]How download a byte array (byte[]) from web API (.Net) with Angular JS
我正在与 SOA 架构师合作解决方案,我在 WS 层上将 a.zip 文件转换为字节数组并通过 web API 从表示层下载时遇到问题。
zip 文件下载成功,但无法解压文件。 让我用代码解释一下:
业务层
在业务层,我们定义了一个方法,将文件 zip 转换为字节数组
//This method is defined on business layer and exposed on WS in WCF Layer
//Class: BusinessLayer
public byte[] convertingZip(){
try{
pathFile = "directoryOnServer/myZipFile.zip"
byte[] arr = File.ReadAllBytes(pathFile);
return arr;
}catch(Exception ex){ /*Do something*/ }
}
WCF 服务层
在WCF服务层,我们编写了一个返回字节数组的方法并将其公开
//Class: ServiceLayer
public byte[] getByteArray(){
try{
BusinessLayer blObject = new BusinessLayer();
return blObject.convertingZip();
}catch(Exception ex){ /*Do something*/ }
}
Web API
在Web API 项目中,我们编写了一个使用 WCF 服务层并将字节数组返回到内容中的方法
//This controller must be return the zip file
[HttpGet]
[AuthorizeWebApi]
[Route("downloadZip")]
public async Task<IHttpActionResult> downloadZipFile(){
try{
using(ServiceLayer services = new ServiceLayer()){
arr = services.getByteArray();
var result = new HttpResponseMensage(HttpStatusCode.OK){
Content = new ByteArrayContent(arr); }
result.Content.Headers.ContentDisposition
= new ContentDispostionHeaderValue("attachment"){
FileName = "zip-dowload.zip" };
result.Content.Headers.ContentType
= new MediaTypeHeaderValue("application/octec-stream");
var response = ResponseMessage(result);
return result;
}
}cacth(Exception ex){ /*Do something*/ }
}
表示层
在表示层上,我使用 angular JS 1.6.5 下载文件
//On Web App project consume the WebApi with Angular
//MyController.js
$scope.DonwloadZip = function(){
$http.get('api/myControllerUrlBase/downloadZip')
.success(function(data, status, headers, config){
if(status === true && data != null){
var file = new Blob([data], {type: "application/zip"});
var fileURL = URL.createObjectUrl(file);
var a = document.createElement(a);
a.href = fileURL;
a.target = "_blank";
a.download = "MyZipFileName.zip";
document.body.appendChild(a);
a.click();
document.body.removeChild(a);
}else { /*Do something */}
})
.error(function(data, status, headers, config) {
//show error message
});
}
我不确定这样做是否正确。 我测试了一些类似的 with.xml,.txt。 and.csv 文件和作品。 但不要使用 zip 文件。
那么,将 zip 文件转换为字节数组并从 web 应用程序项目获取我的 web API 的正确方法是什么?
我会非常感谢你的帮助。
好吧,我已经解决了这个问题,我想分享解决方案。
核心问题在web-api和表示层上,然后我们需要在此处修改代码:
在Api Controller上 ,将字节数组转换为base64上的字符串,并发送http响应内容
[HttpGet]
[AuthorizeWebApi]
[Route("downloadZip")]
public async Task<IHttpActionResult> downloadZipFile(){
try{
//Using WS reference
using(ServiceLayer services = new ServiceLayer()){
//Catch the byte array
arr = services.getByteArray();
//Encode in base64 string
string base64String = System.Convert.ToBase64String(arr, 0, arr.length);
//Build the http response
var result = new HttpResponseMensage(HttpStatusCode.OK){
Content = new StringContent(base64String); }
result.Content.Headers.ContentDisposition
= new ContentDispostionHeaderValue("attachment"){
FileName = "zip-dowload.zip" };
result.Content.Headers.ContentType
= new MediaTypeHeaderValue("application/octec-stream");
var response = ResponseMessage(result);
return result;
}
}cacth(Exception ex){ /*Do something*/ }
}
在Angular Controller上 ,在Blob上不转换数据响应,定义有关数据响应的元数据并下载:
$scope.DonwloadZip = function(){
$http.get('api/myControllerUrlBase/downloadZip')
.success(function(data, status, headers, config){
if(status === true && data != null){
//No convert data on Blob
var fileURL = 'data:application/octec-stream;charset=utf-8;base64,'+ data;
var a = document.createElement(a);
a.href = fileURL;
a.target = "_blank";
a.download = "MyZipFileName.zip";
document.body.appendChild(a);
a.click();
document.body.removeChild(a);
}else { /*Do something */}
})
.error(function(data, status, headers, config) {
//show error message
});
}
请记住,如果您使用的是Angular 1.6.5或更高版本,则http请求必须类似于:
$http({
method: 'GET',
url: 'api/myControllerUrlBase/downloadZip'
}).then(function (response){
//Success
},function (error){
//Error
});
我希望对某人有用,感谢尝试帮助!
我可以通过下面的方法实现这个
downloadFile(base64: string,filename: string, mimetype: string) {
var fileURL = 'data:application/octec-stream;charset=utf-8;base64,' + base64;
var a = document.createElement('a');
a.href = fileURL;
a.target = "_blank";
a.download = filename + ".pdf";
document.body.appendChild(a);
a.click();
document.body.removeChild(a);
}
<a [href]="fileUrl" (click)="downloadFile(type.base64String,type.name,type.extension)"> {{type.name}}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.