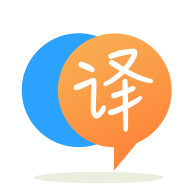
[英]compare two strings and remove common character from second and concatenate uncommon?
[英]Java | How to remove common word from a string and then concatenate uncommon words?
我在接受采访时被问到你有两个段落
P1 = I am Lalit
P2 = Lalit Kumar
现在找到常用词,而不是字符,然后打印不常见的。
Ex: I am Kumar
什么是解决这个问题的最佳方法?
这可能是一种矫枉过正,但我会拆分两个字符串,将它们收集到LinkedHashMap
(以保留原始顺序)并计算每个字符串出现的次数,然后过滤掉非唯一条目:
String p1 = "I am Lalit";
String p2 = "Lalit Kumar";
String result =
Stream.concat(Arrays.stream(p1.split("\\s")), Arrays.stream(p2.split("\\s")))
.collect(Collectors.groupingBy(Function.identity(),
LinkedHashMap::new,
Collectors.counting()))
.entrySet()
.stream()
.filter(e -> e.getValue() == 1)
.map(Map.Entry::getKey)
.collect(Collectors.joining(" "));
试试这段代码:
public static void main(String[] args) {
String str1 = "I am Lalit";
String str2 = "Lalit Kumar";
List<String> set1 = new ArrayList<>(Arrays.asList(str1.split(" ")));
for (String s : Arrays.asList(str2.split(" "))) {
if (set1.contains(s)) set1.remove(s);
else set1.add(s);
}
System.out.println(String.join(" ", set1));
}
你可以这样做......
public static void printUncommon(String s1, String s2) {
String[] a = s1.split(" ");
String[] b = s2.split(" ");
Arrays.sort(a);
Arrays.sort(b);
int n1 = a.length;
int n2 = b.length;
int i = 0;
int j = 0;
while(i < n1 && j < n2) {
int compare = a[i].compareToIgnoreCase(b[j]);
if(compare == 0) {
i++;
j++;
}
else if(compare < 0) {
System.out.println(a[i]);
i++;
}
else if(compare > 0) {
System.out.println(b[j]);
j++;
}
}
if(i == n1) {
while(j < n2) {
System.out.println(b[j]);
j++;
}
}
else if(j == n2) {
while(i < n1) {
System.out.println(a[i]);
i++;
}
}
}
因此,考虑到数组a
和b
的大小,可以说该算法以O(n1 + n2)的效率运行,其中我们排除了排序时间和比较字符串所花费的时间。
我希望你能理解算法。 如果没有,请告诉我,我可以一步一步指导您。 干杯!
使用ArrayList,下面的解决方案应该可行,
String p1 = "I am Lalit";
String p2 = "Lalit Kumar";
List p2_wordList = Arrays.asList(p2.split(" ")); // create List reference
List<String> listOfAlllWords = new ArrayList<String>(Arrays.asList(p1.split(" "))); // create a new ArrayList Object for all words in p1
listOfAlllWords.addAll(p2_wordList); // add all words from p2
System.out.println(listOfAlllWords); // output-> [I, am, Lalit, Lalit,Kumar]
List<String> listOfCommonWords = new ArrayList<String>(Arrays.asList(p1.split(" "))); // create a new ArrayList Object for all word in p1 one more time
listOfCommonWords.retainAll(p2_wordList); // retain only common words from p1 and p2
System.out.println(listOfCommonWords); // output--> [Lalit]
listOfAlllWords.removeAll(listOfCommonWords); // remove above common words from istOfAlllWords
System.out.println(listOfAlllWords); // output--> [I, am, Kumar]
StringBuffer sb = new StringBuffer(); // for final output
Iterator<String> itr = listOfAlllWords.iterator();
while (itr.hasNext()) {
sb.append(itr.next() + " ");
}
System.out.println(sb);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.