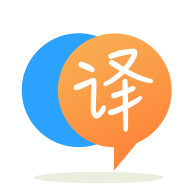
[英]Error: System.ArgumentOutOfRangeException: Index was out of range. Must be non-negative and less than the size of the collection
[英]ArgumentOutOfRangeException - index was out of range. Must be non-negative and less than the size of the collection
错误:ArgumentOutOfRangeException-索引超出范围。 必须为非负数且小于集合的大小
场景:一个桌面应用程序,它从SQL Server数据库中读取数据。 当我想单击按钮时,它将打开表单并从数据库中读取数据,然后将其自动放入“数据网格视图”中,但这会出现异常。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace BashgaheVarzeshiI2
{
public partial class frmuser : Form
{
public frmuser()
{
InitializeComponent();
}
SqlConnection con = new SqlConnection("Data source=(local);initial catalog=BashgahDB; integrated security = true");
SqlCommand cmd = new SqlCommand();
void Display()
{
DataSet ds = new DataSet();
SqlDataAdapter adp = new SqlDataAdapter();
adp.SelectCommand = new SqlCommand();
adp.SelectCommand.Connection = con;
adp.SelectCommand.CommandText = "select * from Karbar";
adp.Fill(ds, "Karbar");
dgvUser.DataSource = ds;
dgvUser.DataMember = "Karbar";
dgvUser.Columns[0].HeaderText = "code";
dgvUser.Columns[1].HeaderText = "username";
dgvUser.Columns[2].HeaderText = "password";
dgvUser.Columns[0].Width = 50;
}
private void bunifuImageButton1_Click(object sender, EventArgs e)
{
this.Close();
}
private void bunifuImageButton2_Click(object sender, EventArgs e)
{
this.WindowState = FormWindowState.Minimized;
}
private void bunifuImageButton6_Click(object sender, EventArgs e)
{
this.Close();
}
private void bunifuImageButton3_Click(object sender, EventArgs e)
{
try
{
cmd.Parameters.Clear();
cmd.Connection = con;
cmd.CommandText = "insert into Karbar(UName,Password)values(@Uname,@Password)";
cmd.Parameters.AddWithValue("@Uname", bunifuMaterialTextbox1.Text);
cmd.Parameters.AddWithValue("@Password", bunifuMaterialTextbox2.Text);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
Display();
MessageBox.Show("Username Saved");
}
catch (Exception)
{
MessageBox.Show("Error!");
}
}
private void frmuser_Load(object sender, EventArgs e)
{
Display();
}
private void bunifuImageButton4_Click(object sender, EventArgs e)
{
try
{
int x = Convert.ToInt32(dgvUser.SelectedCells[0].Value);
cmd.Parameters.Clear();
cmd.Connection = con;
cmd.CommandText = "Delete From Karbar where id=@N";
cmd.Parameters.AddWithValue("@N", x);
con.Open();
cmd.ExecuteNonQuery();
con.Close();
Display();
MessageBox.Show("User Deleted!");
}
catch (Exception)
{
MessageBox.Show("Error!");
}
}
private void dgvUser_SelectionChanged(object sender, EventArgs e)
{
}
}
}
我该如何克服这个错误?
您正在尝试访问不存在且在范围之外的数组元素。 查看您的代码,在Load
调用Display
方法,因此看来Columns索引是问题所在,即您在尝试访问它们之前未设置任何列。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.