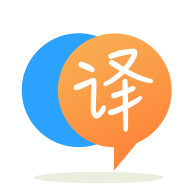
[英]How to read all contents of a column of excel file into an array C#? Without using ADO.net approach
[英]How to read decimal and dates from Excel with C# and ADO.NET
我正在尝试使用以下代码(ADO.NET)在ASP.NET应用程序中读取Excel文件:
// Create OleDbCommand object and select data from worksheet Sheet1
String query = String.Format("Select * From [{0}$]", sheetName);
OleDbCommand cmd = new OleDbCommand(query, oledbConn);
// Create new OleDbDataAdapter
OleDbDataAdapter oleda = new OleDbDataAdapter();
oleda.SelectCommand = cmd;
//Fills Data to DataTable
oleda.Fill(dt);
问题在于数据表中的值被表示
1)对于带逗号(3,14)或带点(3.14)的小数
2)对于格式为“ DD / MM / YYYY”或“ MM / DD / YYYY”的日期
对于相同的Excel文件,取决于服务器的语言环境设置。
有什么方法可以读取特定语言环境中的数据,以便从Excel文件中获取正确的值?
对于带有小数的列,请使用以下内容对其进行解析:( Decimal.Parse
)
string decimal1 = "3,14";
string decimal2 = "3.14";
decimal d1 = decimal.Parse(decimal1, new NumberFormatInfo { NumberDecimalSeparator = "," });
decimal d2 = decimal.Parse(decimal2);
请注意,使用,
您需要使用自定义NumberFormatInfo
对其进行正确解析。
然后为您的DateTime
列:
string date1 = "14/03/2018";
string date2 = "03/14/2018";
DateTime dt1 = DateTime.ParseExact(date1, "dd/MM/yyyy", CultureInfo.InvariantCulture);
DateTime dt2 = DateTime.ParseExact(date2, "MM/dd/yyyy", CultureInfo.InvariantCulture);
在这里,您将要指定日期的不同格式。
编辑:
为了更全面地解析未知的DateTime
格式,如果您知道可能有多个,可以尝试遍历CultureInfo
或多种CultureInfo
所有格式。 以下是如何执行此操作的示例:
string dateTimeString = @"03/14/2018";
string[] possibleStandardFormats = new CultureInfo("en-US")
.DateTimeFormat.GetAllDateTimePatterns();
DateTime? result = null;
foreach (string format in possibleStandardFormats) {
if (DateTime.TryParse(dateTimeString, out DateTime dateTime)) {
// this format could work
result = dateTime;
break;
}
}
if (result == null) {
// no luck with any format
// try one last parse
if (DateTime.TryParse(dateTimeString, out DateTime dateTime)) {
// finally worked
result = dateTime;
}
else {
// no luck
}
}
在这里,首先尝试常规的DateTime.TryParse
可能更有效(如本示例结尾所示),因为它可以为您节省其他格式的迭代。 由您决定如何处理,但是此示例应处理大多数情况。
编辑2:
为了获得标准的DateTime
格式,您可以使用CurrentCulture
,它将对您的日期有所帮助。 在我之前的编辑中,我对new CultureInfo("en-US")
硬编码,但以下内容更为通用。
string[] possibleStandardFormats = new CultureInfo(CultureInfo.CurrentCulture.Name)
.DateTimeFormat.GetAllDateTimePatterns();
编辑3:要进一步解释小数点以前的解析,请首先检查字符串是否有逗号,然后根据我上面列出的方法解析它。
string decimal1 = "3,14";
if (decimal1.Contains(",")) {
decimal d1 = decimal.Parse(decimal1, new NumberFormatInfo { NumberDecimalSeparator = "," });
}
else {
decimal d1 = decimal.Parse(decimal1);
}
编辑4:
要将区域性纳入解析小数,可以尝试使用Convert.ToDecimal
方法。 它的参数之一是CultureInfo
,您可以在其中将当前文化作为CultureInfo.CurrentCulture
传递。 例如,在下面的示例中,我使用的是de-de
(德语),因为这是1.234,14
的有效区域性
string decimal1 = "1.234,14";
string decimal2 = "1,234.14";
decimal d1 = Convert.ToDecimal(decimal1, new CultureInfo("de-de"));
decimal d2 = Convert.ToDecimal(decimal2, CultureInfo.CurrentCulture);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.