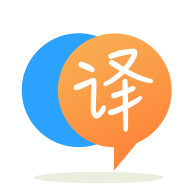
[英]when Pressed Back Button on RecyclerView it takes to the Home Screen
[英]Is RecyclerView refreshed when AlertDialog's positive button gets pressed
我的MainActivity有一个RecyclerView
适配器,并且通过AlertDialog将数据添加到此RecyclerView,AlertDialog将输入的文本传递给MainActivity。 即使在传递新输入后从不调用notifyItemInserted()或notifyDatasetChange(),单击对话框中的肯定按钮时,回收者视图也会以某种方式刷新。 我想知道这是怎么发生的,我的猜测是在对话框中按下肯定按钮后,recyclerview会以某种方式刷新
自定义AlertDialog代码:
public class CustomDialog extends AppCompatDialogFragment {
OnNoteAddedListener onNoteAddedListener;
public interface OnNoteAddedListener {
public void onClick(String note);
}
public CustomDialog(OnNoteAddedListener onNoteAddedListener) {
this.onNoteAddedListener = onNoteAddedListener;
}
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
// Get the layout inflater
final LayoutInflater inflater = getActivity().getLayoutInflater();
final View dialogLayout = inflater.inflate(R.layout.dialog_box, null);
// Inflate and set the layout for the dialog
// Pass null as the parent view because its going in the dialog layout
builder.setView(dialogLayout).setPositiveButton("Ok", new DialogInterface.OnClickListener() {@Override
public void onClick(DialogInterface dialog, int id) {
EditText addNote = dialogLayout.findViewById(R.id.note_text);
String note = addNote.getText().toString();
onNoteAddedListener.onClick(note);
}
}).setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
CustomDialog.this.getDialog().cancel();
}
});
return builder.create();
}
}
适配器代码:
class RecyclerViewAdapter extends RecyclerView.Adapter<RecyclerViewAdapter.ViewHolder>
{
private static final String TAG = "RecyclerViewAdapter";
private List<String> notesList;
private Context mContext;
private SendPositionConnector sendPositionConnector;
public interface SendPositionConnector
{
public void sendPosition(int position);
}
public RecyclerViewAdapter(List<String> notesList, Context mContext)
{
this.notesList = notesList;
this.mContext = mContext;
this.sendPositionConnector = (MainActivity)mContext;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType)
{
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.layout_listitem, parent, false);
ViewHolder holder = new ViewHolder(view);
return holder;
}
@Override
public void onBindViewHolder(@NonNull ViewHolder viewHolder, final int position)
{
Log.d(TAG, "onBindViewHandler: called");
viewHolder.noteContent.setText(notesList.get(position));
viewHolder.parentLayout.setOnLongClickListener(new View.OnLongClickListener(){
@Override
public boolean onLongClick(View view)
{
Log.d(TAG, "onLongClick: long clicked on");
sendPositionConnector.sendPosition(position);
return false;
}
});
}
@Override
public int getItemCount()
{
return notesList.size();
}
public class ViewHolder extends RecyclerView.ViewHolder
{
TextView noteContent;
RelativeLayout parentLayout;
ImageView bullet;
public ViewHolder(@NonNull View itemView)
{
super(itemView);
bullet = itemView.findViewById(R.id.bullet);
noteContent = itemView.findViewById(R.id.text_content);
parentLayout = itemView.findViewById(R.id.parent_layout);
}
}
}
活动代码:
public class MainActivity extends AppCompatActivity implements RecyclerViewAdapter.SendPositionConnector
{
private static final String TAG = "MainActivity";
private List<String> notesList = new ArrayList<>();
private RecyclerView recyclerView;
private RecyclerViewAdapter adapter;
private int position;
public AgentAsyncTask agentAsyncTask;
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
recyclerView = findViewById(R.id.my_recycler_view);
registerForContextMenu(recyclerView);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
agentAsyncTask = new AgentAsyncTask(notesList, getApplicationContext(), true, new AgentAsyncTask.OnRead(){
@Override
public void onRead(List<String> notesList)
{
if(!notesList.isEmpty())
MainActivity.this.notesList = notesList;
adapter = new RecyclerViewAdapter(notesList, MainActivity.this);
recyclerView.setAdapter(adapter);
}
});
agentAsyncTask.execute();
}
@Override
public boolean onCreateOptionsMenu(Menu menu)
{
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.add_menu, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item)
{
// Handle item selection
switch (item.getItemId())
{
case R.id.add_note:
showDialogBox(item);
return true;
default:
return super.onOptionsItemSelected(item);
}
}
@Override
protected void onStop()
{
super.onStop();
new AgentAsyncTask(notesList, getApplicationContext(), false, new AgentAsyncTask.OnRead(){
@Override
public void onRead(List<String> notesList)
{
if(!notesList.isEmpty())
MainActivity.this.notesList = notesList;
}
}).execute();
}
@Override
protected void onDestroy()
{
super.onDestroy();
}
private boolean showDialogBox(MenuItem menuItem)
{
AppCompatDialogFragment dialogFragment = new CustomDialog(new CustomDialog.OnNoteAddedListener(){
@Override
public void onClick(String note)
{
Log.d(TAG, "onClick: "+ note);
notesList.add(note);
}
});
dialogFragment.show(getSupportFragmentManager(),"Adding");
return true;
}
@Override
public void onCreateContextMenu(ContextMenu menu, View v, ContextMenu.ContextMenuInfo menuInfo)
{
super.onCreateContextMenu(menu, v, menuInfo);
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.context_menu, menu);
}
@Override
public boolean onContextItemSelected(MenuItem menuItem)
{
switch(menuItem.getItemId())
{
case R.id.delete:
notesList.remove(position);
adapter.notifyItemRemoved(position);
adapter.notifyItemRangeChanged(position, notesList.size());
return true;
default:
return false;
}
}
@Override
public void sendPosition(int position)
{
this.position = position;
}
private static class AgentAsyncTask extends AsyncTask<Void, Void, List<String>>
{
private List<String> notesList;
private boolean flag;
OnRead onRead;
Context context;
AppDataBase dataBase;
private static final String TAG = "AgentAsyncTask";
public interface OnRead
{
public void onRead(List<String> notesList);
}
private AgentAsyncTask(List<String> notesList,Context context,boolean flag, OnRead onRead)
{
this.notesList = notesList;
this.onRead = onRead;
this.flag = flag;
this.context = context;
}
@Override
protected List<String> doInBackground(Void... params)
{
dataBase = Room.databaseBuilder(context, AppDataBase.class, "database-name").build();
if(!flag)
{
Gson gson = new Gson();
Type type = new TypeToken<List<String>>() {}.getType();
String json = gson.toJson(notesList, type);
Log.d(TAG, "doInBackground: "+json);
Notes notes = new Notes();
notes.setNoteContent(json);
notes.setUid(1);
dataBase.notesDao().insertNotes(notes);
return notesList;
}
else
{
Gson gson = new Gson();
String notesListContent = dataBase.notesDao().getNotes();
if(dataBase.notesDao().getCount() != 0)
{
notesList = gson.fromJson(notesListContent, new TypeToken<List<String>>()
{
}.getType());
}
else
{
return notesList;
}
return notesList;
}
}
@Override
protected void onPostExecute(List<String> notesList)
{
super.onPostExecute(notesList);
if(flag)
onRead.onRead(notesList);
}
}
}
当您按下肯定按钮后将文本从对话框中移至主要活动时。 在列表中添加要传递给适配器和调用方法的新文本
adapter.notifyDataSetChanged();
可能发生的情况是,当对话框返回时,它将导致RecyclerView的重新布局,从而重新绑定视图。 但是,这很容易出现错误,因为它可能尚未更新回收站的列表长度或项目视图类型等内容,因此应始终使用适当的notify
方法。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.