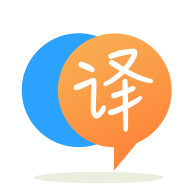
[英]**[UPDATE]** How to animating background expandation of cardview in android?
[英]Android CardView not animating properly
实施CardView
并尝试将动画应用于箭头文本视图后,单击箭头时动画显示异常行为。 如何修复动画并获得与预期动画图像相似的结果?
预期动画
当前动画
recyclerview_item
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.CardView
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:animateLayoutChanges="true"
android:clickable="true"
android:focusable="true"
android:foreground="?android:attr/selectableItemBackground"
android:id="@+id/cv"
android:layout_marginBottom="20dp"
>
<LinearLayout
android:id="@+id/cardview_main"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="10dp"
android:animateLayoutChanges="true">
<LinearLayout
android:id="@+id/cardview_titlerow"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:layout_marginBottom="2dp"
android:weightSum="100">
<TextView
android:id="@+id/tv_A"
android:layout_weight="90"
android:layout_width="0dp"
android:layout_height="wrap_content"
style="@android:style/TextAppearance.Medium" />
<TextView
android:id="@+id/tv_expandcollapsearrow"
android:clickable="true"
android:focusable="true"
android:layout_weight="10"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="10dp"
android:textColor="@android:color/white"
style="@android:style/TextAppearance.Medium" />
</LinearLayout>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
>
<TextView
android:id="@+id/tv_B"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="@android:style/TextAppearance.Large"
/>
</RelativeLayout>
</LinearLayout>
</android.support.v7.widget.CardView>
MyRecyclerAdapter.java
public class MyRecyclerAdapter extends RecyclerView.Adapter<RecyclerView.ViewHolder> {
private static final int TYPE_HEADER = 0;
private static final int TYPE_ITEM = 1;
private Context mContext;
RecyclerViewHeader header;
List<MyRecyclerItem> listItems;
private Animation animationCollapse;
private Animation animationExpand;
public MyRecyclerAdapter(Context context, RecyclerViewHeader header, List<MyRecyclerItem> listItems)
{
this.mContext = context;
this.header = header;
this.listItems = listItems;
}
@Override
public RecyclerView.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
if(viewType == TYPE_HEADER)
{
View v = LayoutInflater.from(parent.getContext()).inflate(R.layout.recyclerview_header_expandcollapsebuttons, parent, false);
return new MyRecyclerAdapter.VHHeader(v);
}
else if(viewType == TYPE_ITEM)
{
View v = LayoutInflater.from(parent.getContext()).inflate(R.layout.recyclerview_item, parent, false);
return new MyRecyclerAdapter.VHItem(v);
}
throw new RuntimeException("there is no type that matches the type " + viewType + " + make sure your using types correctly");
}
private MyRecyclerItem getItem(int position)
{
return listItems.get(position);
}
@Override
public void onBindViewHolder(RecyclerView.ViewHolder holder, int position) {
final Typeface iconFont = FontManager.getTypeface(mContext, FontManager.FONTAWESOME);
if (holder instanceof VHHeader)
{
VHHeader VHheader = (VHHeader)holder;
}
else if (holder instanceof VHItem)
{
RecyclerViewListItemTransportConnections currentItem = getItem(position-1);
final VHItem VHitem = (VHItem)holder;
VHitem.txtA.setText(currentItem.getConnectionMode());
VHitem.txtB.setText(currentItem.getConnectionName());
VHitem.txtB.setVisibility(View.GONE);
VHitem.txtExpandCollapseArrow.setText(R.string.fa_icon_chevron_down);
VHitem.txtExpandCollapseArrow.setTypeface(iconFont);
VHitem.txtExpandCollapseArrow.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (VHitem.txtB.getVisibility() == View.VISIBLE) {
VHitem.txtB.setVisibility(View.GONE);
RotateAnimation rotate = new RotateAnimation(180, 0, Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f);
rotate.setDuration(300);
rotate.setInterpolator(new LinearInterpolator());
VHitem.txtExpandCollapseArrow.startAnimation(rotate);
VHitem.txtExpandCollapseArrow.setText(R.string.fa_icon_chevron_down);
} else {
VHitem.txtB.setVisibility(View.VISIBLE);
RotateAnimation rotate = new RotateAnimation(0, 180, Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f);
rotate.setDuration(300);
rotate.setInterpolator(new LinearInterpolator());
VHitem.txtExpandCollapseArrow.startAnimation(rotate);
TransitionManager.beginDelayedTransition(VHitem.cardview);
VHitem.txtExpandCollapseArrow.setText(R.string.fa_icon_chevron_up);
}
}
});
}
}
@Override
public int getItemViewType(int position) {
if(isPositionHeader(position))
return TYPE_HEADER;
return TYPE_ITEM;
}
private boolean isPositionHeader(int position)
{
return position == 0;
}
@Override
public int getItemCount() {
return listItems.size()+1;
}
class VHHeader extends RecyclerView.ViewHolder{
public VHHeader(View itemView) {
super(itemView);
}
}
class VHItem extends RecyclerView.ViewHolder{
CardView cardview;
TextView txtExpandCollapseArrow, txtA, txtB;
public VHItem(View itemView) {
super(itemView);
this.cardview = itemView.findViewById(R.id.cv);
this.txtExpandCollapseArrow = itemView.findViewById(R.id.tv_expandcollapsearrow);
this.txtA = itemView.findViewById(R.id.tv_A);
this.txtB = itemView.findViewById(R.id.tv_B);
}
}
}
苏拉卜的建议
一种更简单的方法是设置可隐藏视图的可见性,并使用TransitionManager
设置这些布局更改的动画。
TransitionManager.beginDelayedTransition(rootView)
// rootView can be the container view of all cards
这样可以增加卡的高度并淡化这些视图。我不确定是否也可以旋转箭头,但是您可以尝试。 或者您可以使用RotateAnimation
预期的动画似乎是矢量可绘制的动画,而您遵循的方法使视图(而非可绘制的)动画化。
您需要使用Animated Vector drawable才能获得所需的结果。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.