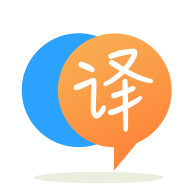
[英]How to add body in the request and get in second rest service in nodejs express
[英]How to extract body from request with express in NodeJs?
我正在使用Node.Js并为我的应用程序express
框架。
我建立HTML表单,但提交后无法收到API请求的form
数据。
我的HTML:
<form method="post" action="/create">
<input type="text" name="user.name" />
<input type="text" name="user.email" />
<input type="text" name="user.address.city" />
<input type="text" name="user.address.land" />
<input type="submit" value="Submit">
</form>
JSON对象应该在我的API中获得:
{
"user": {
"name": "toto",
"email": "toto@mail.com",
"address": {
"city": "yyyyy",
"land": "zzzz"
}
}
}
如何使用Node.js Express 4做到这一点,还有另一个库吗?
您可以准备自己的中间件,该中间件使用body-parser的urlencoded()
解析传入的表单数据,并将其转换为结构化JSON:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
function setDeepValue(path, obj, value) {
const tokens = path.split('.');
const last = tokens.pop();
for (const token of tokens) {
if (!obj.hasOwnProperty(token)) {
obj[token] = {};
}
obj = obj[token];
}
obj[last] = value;
}
app.use(bodyParser.urlencoded(), function(req, res, next) {
let obj = {};
for (const key in req.body) {
setDeepValue(key, obj, req.body[key]);
}
req.body = obj;
next();
});
app.post('/create', function(req, res) {
console.log(req.body)
})
在您的HTML代码中,您要发布到创建路径。
因此,您需要创建一条路线
const express = require('express')
const bodyParser = require('body-parser')
const app = express()
app.use(bodyParser.json())
app.use(bodyParser.urlencoded({ extended: true }))
app.post('/create', function(req, res) {
console.log('----- MY BODY -----')
console.log(req.body)
// do something more clever
res.send('We posted to this route')
})
首先,我们需要express,然后我们需要body-parser,最后初始化我们的express应用。
然后,我们使用body-parser的json middlewere解析正文,以便我们可以在处理程序中轻松访问它。
然后,我们定义到'/create'
的路由,该路由接受发布请求(请记住您的表单正在发布到此位置)。
我们的处理程序所做的全部工作就是console.log请求的主体,然后显示消息我们已发布到此路由
遵循专门为指导新生而创建的本指南资料库,以指导nodejs-frontend-sample-for-freshers
编辑:
您可以使用Ajax调用提交表单,这在单页应用程序中也有帮助
客户端JS:
function submit() {
// JavaScript uses `id` to fetch value
let email = $("#email").val(),
name = $("#name").val(),
city = $("#city").val(),
land = $("#land").val();
// Can validate each field here and show error like:
if ( validateEmail(email) ) {
$("#emailError").addClass("hide");
} else {
$("#emailError").removeClass("hide");
return;
}
// form request data, doing this will get you data in correct form at NodeJs API
let data = {
user: {
email,
name,
address: {
city,
land
}
}
};
$.ajax({
"url": "/create",
"method": "POST",
"data": data
})
.then( result => {
// On success empty all the input fields.
$("#email").val('');
$("#name").val('');
$("#city").val('');
$("#land").val('');
// Message to notify success submition
alert("Successfully added user.");
return;
})
.catch( err => {
// Notify in case some error occured
alert("An error occured.");
return;
});
}
// Validate Email based upon pattern
function validateEmail (email) {
if ( email && email.match(/^([A-z0-9_.]{2,})([@]{1})([A-z]{1,})([.]{1})([A-z.]{1,})*$/) ) {
return true;
}
return false;
}
HTML:
<form>
<input type="text" id="name" />
<input type="text" id="email" />
<span id="emailError" class="hide error">Valid Email Required</span>
<input type="text" id="city" />
<input type="text" id="land" />
<p onclick="submit()">Submit</p>
</form>
会建议您也使用cors.js
:
const cors = require('cors');
// Cross-Origin Resource Sharing
app.use(cors());
您可以通过两种方式获取对象:
1:不使用任何其他类似这样的模块
app.post('/create', function (request, response, next) {
let body = [];
request.on('error', (err) => {
console.error(err);
}).on('data', (chunk) => {
body.push(chunk);
}).on('end', () => {
body = Buffer.concat(body).toString();
console.log(body); // Your object
request.body = body; // Store object in request again
next();
});
}, (req, res) => {
console.log(req.body); // This will have your object
});
express
使用body-parser
: ```
// configure the app to use bodyParser() to extract body from request.
// parse urlencoded types to JSON
app.use(bodyParser.urlencoded({
extended: true
}));
// parse various different custom JSON types as JSON
app.use(bodyParser.json({ type: 'application/*+json' }));
// parse some custom thing into a Buffer
app.use(bodyParser.raw({ type: 'application/vnd.custom-type' }));
// parse an HTML body into a string
app.use(bodyParser.text({ type: 'text/html' }));
app.post('/create', function (request, response, next) {
console.log(request.body); // `body-parser did what I did earlier`
});
```
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.