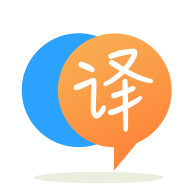
[英]How to get Json Array in Json Object using volley in android and set this json data in listview code and Xml Avilavble
[英]How do I POST a JSON array and get a JSON object in response using preferably android Volley?
我需要发布的API需要一个JSONArray,但使用JSONObject进行响应。 不幸的是,据我所知,Android Volley库对此没有任何方法。
有没有一种方法可以编写自定义请求,并且该如何完成我上面解释的工作?
如果Volley无法做到这一点,您会如何建议我这样做?
我相信该方法看起来像这样:
//数组:
JSONArray itemArray = new JSONArray();
try {
for (MenuItem menuItem : listOfItems) {
JSONObject item = new JSONObject();
Log.d(LOG_TAG, "Item ID--> " + menuItem.getId());
Log.d(LOG_TAG, "Item Quantity--> " + menuItem.getNumOrdered());
Log.d(LOG_TAG, "Item Price Lvl--> " +
menuItem.getPrice_levels().get(0).getId().toString());
Log.d(LOG_TAG, "Item Comments--> " +
menuItem.getSpecialInstructions());
item.put("menu_item", menuItem.getId());
item.put("quantity", menuItem.getNumOrdered());
item.put("price_level",
menuItem.getPrice_levels().get(0).getId().toString());
item.put("comment", menuItem.getSpecialInstructions());
JSONArray discounts = new JSONArray();
JSONObject discount = new JSONObject();
discount.put("discount", null);
item.put("discounts", discounts);
JSONArray modifiers = new JSONArray();
JSONObject modifier = new JSONObject();
modifier.put("modifier",
menuItem.getModifierGroups().get(0).getId());
item.put("modifiers", modifiers);
itemArray.put(item);
}
//排球要求
JsonArrayRequest jsArrayRequest = new JsonArrayRequest(Request.Method.POST,
url, itemArray, new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
try {
finalized();
} catch (Exception e) {
Log.v("volley ex", e.toString());
}
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
Log.v("volley error", error.toString());
}
}) {
@Override
public Map<String, String> getHeaders() throws AuthFailureError {
Map<String, String> headers = new HashMap<>();
headers.put("Api-Key", "api key");
headers.put("Content-Type", "application/json");
return headers;
}
};
requestQueue.add(jsArrayRequest);
}
// menuitem类
package com.garcon.garcon;
import java.io.Serializable;
import java.util.ArrayList;
public class MenuItem implements Serializable {
public String id;
public String name;
public Integer price;
protected ArrayList<PriceLevel> price_levels;
protected Boolean in_stock;
protected int modifier_groups_count;
protected ArrayList<ModifierGroup> mGroups;
//not defined in API but will give Category's ID to MenuItem
protected String categoryID;
//user defined variable
public int numOrdered = 0;
private String specialInstructions = "";
//http://stackoverflow.com/questions/18814076/how-to-make-intellij-show-
//eclipse-like-api-documentation-on-mouse-hover
/**
* Complete constructor for MenuItem.
*
* @param id The menu item’s id as stored in the POS. Sometimes a compound
value derived from other data
* @param name The name of the Menu Item as stored in the POS
* @param price The price, in cents
* @param price_levels Array of Hashes (id String Price Level Identifier,
price Integer The price of the menu item at this price level, in cents)
* @param in_stock Whether or not the item is currently available for order.
* @param mGroups Modifier Groups associated with the Menu Item.
* @param modifier_groups_count The number of Modifier Groups associated
with the Menu Item.
* @param categoryID parent category's id NOT name
*/
public MenuItem(String id, String name, Integer price, ArrayList<PriceLevel>
price_levels,
Boolean in_stock, ArrayList<ModifierGroup> mGroups, Integer
modifier_groups_count, String categoryID){
this.id = id;
this.name = name;
this.price = price;
this.price_levels = price_levels;
this.in_stock = in_stock;
this.mGroups = mGroups;
this.modifier_groups_count = modifier_groups_count;
this.categoryID = categoryID;
}
public ArrayList<PriceLevel> getPrice_levels() {
return price_levels;
}
public void setPrice_levels(ArrayList<PriceLevel> price_levels) {
this.price_levels = price_levels;
}
public String getId() {
return id;
}
public String getName(){
return name;
}
String getCategoryID() {
return categoryID;
}
public Integer getPrice(){
return this.price;
}
ArrayList<ModifierGroup> getModifierGroups(){ return this.mGroups;}
int getNumOrdered(){return this.numOrdered;}
void setNumOrdered(int amount){
numOrdered = amount;
}
String getSpecialInstructions(){return this.specialInstructions;}
void setSpecialInstructions(String instructions){
specialInstructions = instructions;
}
static class ModifierGroup implements Serializable{
private String id, name;
private Integer minimum, maximum;
private boolean required;
private ArrayList<ItemModifier> modifier_list;
public ModifierGroup(String id, String name, int minimum, int maximum,
boolean required, ArrayList<ItemModifier> modifier_list){
this.id = id;
this.name = name;
this.minimum = minimum;
this.maximum = maximum;
this.required = required;
this.modifier_list = modifier_list;
}
public ModifierGroup(){}
String getId(){return id;}
String getName(){return name;}
Integer getMinimum(){return minimum;}
Integer getMaximum(){return maximum;}
boolean isRequired(){return required;}
ArrayList<ItemModifier> getModifierList(){ return
this.modifier_list;}
static class ItemModifier implements Serializable{
private String id, name;
private Integer price_per_unit;
private ArrayList<PriceLevel> priceLevelsList;
//user defined variable
private boolean added = false;
public ItemModifier(String id, String name, Integer
price_per_unit, ArrayList<PriceLevel> priceLevelsList){
this.id = id;
this.name = name;
this.price_per_unit = price_per_unit;
this.priceLevelsList = priceLevelsList;
}
String getId(){return id;}
String getName(){return name;}
Integer getPricePerUnit(){return price_per_unit;}
ArrayList<PriceLevel> getPriceLevelsList(){return
priceLevelsList;}
boolean isAdded(){ return added;}
void setAdded(boolean b){added = b;}
}
static class ItemModifierGrouped extends ItemModifier implements
Serializable{
private int group_id;
public ItemModifierGrouped(String id, String name, Integer
price_per_unit, ArrayList<PriceLevel> priceLevelsList, int
group_id){
super(id,name,price_per_unit,priceLevelsList);
this.group_id = group_id;
}
}
}
public static class PriceLevel implements Serializable{
public String id;
public Integer price;
public PriceLevel(){}
public PriceLevel(String id, Integer price){
this.id = id;
this.price = price;
}
public String getId(){return id;}
public Integer getPrice(){return price;}
}
}
尝试这个
final String httpUrl = url;
Log.i(TAG,httpUrl.toString());
try{
JSONArray parametersForPhp = new JSONArray();
JSONObject jsonObject = new JSONObject();
jsonObject.put(key,"0");
jsonObject.put("key","");
jsonObject.put(key,sharedPreferences.getString(PATIENT_ID,BLANK));
jsonObject.put(APP_LANGUAGE,sharedPreferences.getString(APP_LANGUAGE,BLANK));
parametersForPhp.put(jsonObject);
JsonArrayRequest arrayRequest = new JsonArrayRequest(Request.Method.POST, httpUrl, parametersForPhp,
new Response.Listener<JSONArray>() {
@Override
public void onResponse(JSONArray response) {
Log.i(TAG,response.toString());
if (response==null){
Toast.makeText(getApplicationContext(),"Please Try Again!",Toast.LENGTH_SHORT).show();
}else {
try {
//you code
} catch (JSONException e) {
e.printStackTrace();
}
}
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
error.printStackTrace();
}
});
RequestQueueSingleton.getInstance(getApplicationContext()).addToRequestQueue(arrayRequest);
}catch (Exception e){
e.printStackTrace();
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.