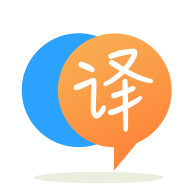
[英]Animate div to full screen size from current position on click, click again to animate back to original size
[英]Animate a div to full screen from its position
我想在单击它时将 div 扩展到全屏。 就像这里的 Fiddle js 链接
我想从它的位置动画。 如果我单击该框,感觉就像从其位置扩展,请帮助我实现这一目标
$('.myDiv').click(function(e){ $(this).toggleClass('fullscreen'); });
.myDiv{background:#cc0000; width:100px; height:100px;float:left:margin:15px;} .myDiv.fullscreen{ z-index: 9999; width: 100%; height: 100%; position: fixed; top: 0; left: 0; }
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <div class="myDiv"> my div <button>Full Screen</button> </div> <div class="myDiv"> my div 2 <button>Full Screen</button> </div>
现在制作一个元素全屏非常简单。 它可以单独使用 css 完成。
.content { display: inline-grid; width: 150px; height: 100px; background-color: cornflowerblue; border-radius: 3px; transition: width 2s, height 2s; margin: 10px; } .content button { display: inline-block; justify-self: center; align-self: center; height: 2em; } .content:hover { width: 100vw; height: 1200vh; }
<div class="container"> <div class="content"> <button>Fullscreen</button> </div> </div> <div class="content"></div>
只需添加一个过渡就会使元素破坏布局。
为了不破坏您需要的布局:
//Function is run on page load $(function() { var full = $(".fullscreen"); //Loops over all elements that have the class fullscreen full.each(function(index, elem) { $(elem).click(fullscreenClick); }); function fullscreenClick() { //The button is this //We want to clone the parent var box = $(this).parent(); //create a holder box so the layout stays the same var holder = $(box).clone(false, true); //and make it not visible $(holder).css({ "visibility": "hidden" }); //Get its position var pos = $(box).position(); //Substitute our box with our holder $(box).before($(holder)); //Set the position of our box (not holder) //Give it absolute position (eg. outside our set structure) $(box).css({ "position": "absolute", "left": pos.left + "px", "top": pos.top + "px", }); //Set class so it can be animated $(box).addClass("fullscreen"); //Animate the position $(box).animate({ "top": 0, "left": 0, }, 3000); } });
* { margin: 0; padding: 0; } .container { display: inline-block; } .container .element { display: inline-block; background-color: cornflowerblue; margin: 5px; padding: 10px; width: 70px; height: 30px; transition: width 3s, height 3s; ; } .container .element.fullscreen { width: calc(100vw - 30px); height: calc(100vh - 30px); }
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <div class="container"> <div class="element"> <button class="fullscreen">Fullscreen</button> </div> <div class="element"> <button class="fullscreen">Fullscreen</button> </div> <div class="element"> <button class="fullscreen">Fullscreen</button> </div> <div class="element"> <button class="fullscreen">Fullscreen</button> </div> <div class="element"> <button class="fullscreen">Fullscreen</button> </div> <div class="element"> <button class="fullscreen">Fullscreen</button> </div> <div class="element"> <button class="fullscreen">Fullscreen</button> </div> <div class="element"> <button class="fullscreen">Fullscreen</button> </div> </div>
您可以在所有样式更改上添加动画,将下一个属性添加到myDiv类:
/* Animate all changes */
-webkit-transition: all 0.5s ease;
-moz-transition: all 0.5s ease;
-o-transition: all 0.5s ease;
transition: all 0.5s ease;
我将展示您示例的更改:
$('.myDiv').click(function(e) { $(this).toggleClass('fullscreen'); });
.myDiv{ background:#cc0000; width:100px; height:100px; float:left: margin:15px; /*Animations*/ -webkit-transition: all 0.5s ease; -moz-transition: all 0.5s ease; -o-transition: all 0.5s ease; transition: all 0.5s ease; } .myDiv.fullscreen{ z-index: 9999; width: 100%; height: 100%; position: fixed; top: 0; left: 0; }
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <div class="myDiv"> my div 1 <button>Full Screen</button> </div> <div class="myDiv"> my div 2 <button>Full Screen</button> </div>
这是一项复杂的任务,但这应该让您了解它是如何完成的。 这段代码会遇到一些问题(快速单击会堆叠setTimeout
),但可以解决基础问题。
这个想法是您使用getBoundingClientRect()
计算元素的当前位置并使用它设置初始位置值,这样当您将位置调整为fixed
时,它看起来好像仍然在同一个位置 - 然后当您覆盖这些带有.fullscreen
css 的值, transition
属性将允许它们进行动画处理。
如果单击第一个 div,您会注意到这里最大的问题是它从布局中消失并导致第二个 div 跳到原来的位置,您可能需要一种保留布局的方法。 希望这无论如何作为一个起点是有帮助的。
function getPosition(elem){ const rect = elem.getBoundingClientRect() return { top: rect.top, left: rect.left, width: rect.width, height: rect.height } } function toPx(val){ return [val, 'px'].join('') } $('.myDiv').click(function(e){ if(this.classList.contains('fullscreen')){ this.classList.remove('fullscreen') setTimeout(e => this.style.position = 'static', 1000) //close } else { //open let pos = getPosition(this) this.style.width = toPx(pos.width) this.style.height = toPx(pos.height) this.style.top = toPx(pos.top) this.style.left = toPx(pos.left) console.log(pos) this.classList.add('fullscreen') this.style.position = 'fixed' } });
.myDiv{background:#cc0000; width:100px; height:100px;float:left:margin:15px;} .myDiv.fullscreen{ z-index: 9999; width: 100% !important; height: 100% !important; top: 0 !important; left: 0 !important; } .animateTransitions { transition: all 1s; }
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <div class="myDiv animateTransitions"> my div <button>Full Screen</button> </div> <div class="myDiv animateTransitions"> my div 2 <button>Full Screen</button> </div>
这是我的做法:
在行动中看到它: https ://codepen.io/illegalmexican/pen/NWYNVvg
请注意,我正在回答一个 JQuery 帖子。 fowlloing 可以用 Vanilla Javascript 制作以获得更好的性能。 另请记住,这也可以通过关闭按钮在可访问性标准方面得到改善。
html:
<div class="myDiv-Container">
<div class="row">
<div class="col-6">
<div class="d-flex flex-wrap position-relative">
<div class="myDiv w-50">
<div class="front bg-primary top-left">
<h1>Hello<br>World</h1>
</div>
</div>
<div class="myDiv w-50">
<div class="front bg-success top-right">
<h1>Hello<br>World</h1>
</div>
</div>
<div class="myDiv w-50">
<div class="front bg-danger bottom-left">
<h1>Hello<br>World</h1>
</div>
</div>
<div class="myDiv w-50">
<div class="front bg-warning bottom-right">
<h1>Hello<br>World</h1>
</div>
</div>
</div>
</div>
</div>
</div>
SCSS:
.myDiv-Container {
height: 500px;
width: 500px;
position: relative;
}
.front {
position: relative;
text-align: center;
display: flex;
&.position-absolute {
z-index: 1;
}
&.top-left {
top: 0;
left: 0;
}
&.bottom-left {
bottom: 0;
left: 0;
}
&.top-right {
top: 0;
right: 0;
}
&.bottom-right {
bottom: 0;
right: 0;
}
}
查询:
document.addEventListener('DOMContentLoaded', function () {
jQuery('.myDiv').each(function () {
var frontElem = jQuery(this).find('.front');
jQuery(this).on("click", function () {
if (jQuery(this).hasClass('active')) {
jQuery(this).removeClass('active');
jQuery(frontElem).animate(
{
width: jQuery(this).width(),
height: jQuery(this).height(),
},
500, function() {
jQuery(frontElem).removeClass('position-absolute');
jQuery(frontElem).css({
width: '100%',
height: '100%',
});
});
} else {
jQuery(frontElem).css({
width: jQuery(this).width(),
height: jQuery(this).height(),
});
jQuery(frontElem).addClass('position-absolute');
jQuery(this).addClass('active');
jQuery(frontElem).animate({
'width': '100%',
'height': '100%',
}, 500);
}
});
});
});
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.