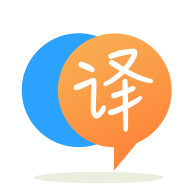
[英]Increment clickcount label value in tableview cell whenever i click that row
[英]Swift:- Increment label value by 1 on button click in custom tableview cell
我试图在自定义表格单元格内的按钮单击时将标签值增加 1。 我能够在不同的单元格中以不同的方式更改值,但问题是如果我在第一个单元格标签中点击 + 从 0 更改为 1,当我在第二个单元格标签中点击 + 直接从 0 更改为 2 时,在其他单元格中依此类推。 我怎样才能解决这个问题?
var counts = 0
@IBAction func addPressed(_ sender: UIButton) {
let indexPath = IndexPath(row: sender.tag, section: 0)
let cell = tblview.cellForRow(at: indexPath) as! HomeDetailsTableViewCell
counts = (counts + 1)
cell.lblNoOfItems.text = "\(counts)"
}
有两种方法可以实现这一点:
1.在controller
级别保持Array
中每个单元格的计数,每次按下button
,从数组中获取计数并使用它,即
var counts = [Int](repeating: 0, count: 10) //10 is the number of cells here
@IBAction func addPressed(_ sender: UIButton) {
let indexPath = IndexPath(row: sender.tag, section: 0)
let cell = tblview.cellForRow(at: indexPath) as! HomeDetailsTableViewCell
counts[indexPath.row] += 1
cell.lblNoOfItems.text = "\(counts[indexPath.row])"
}
2.在自定义单元格中保持每个单元格的计数,即在HomeDetailsTableViewCell
,即
class HomeDetailsTableViewCell: UITableViewCell {
var count = 0
//Rest of the code...
}
@IBAction func addPressed(_ sender: UIButton) {
let indexPath = IndexPath(row: sender.tag, section: 0)
let cell = tblview.cellForRow(at: indexPath) as! HomeDetailsTableViewCell
cell.count += 1
cell.lblNoOfItems.text = "\(cell.count)"
}
此外,您实现添加+ button
的代码的方式不正确。 您必须在HomeDetailsTableViewCell
本身中实现它,即
class HomeDetailsTableViewCell: UITableViewCell {
@IBOutlet weak var lblNoOfItems: UILabel!
var count = 0
@IBAction func addPressed(_ sender: UIButton) {
self.count += 1
self.lblNoOfItems.text = "\(self.count)"
}
//Rest of the code...
}
您可以在Swift 5 中使用以下代码获得此问题的答案
// 第一步
在你的TableViewCell里面
import UIKit
@objc protocol CellDelagate : NSObjectProtocol {
func addPressed(_ cell: TableViewCell)
}
class TableViewCell: UITableViewCell {
var cellDelagate: CellDelagate?
var indexPath : IndexPath?
@IBOutlet weak var gPaybutton: UIButton!
@IBOutlet weak var proceesingLabel: UILabel!
var count = 0
override func awakeFromNib() {
super.awakeFromNib()
// Initialization code
}
@IBAction func proccessingButtonTapped(_ sender: UIButton) {
self.cellDelagate!.addPressed(self)
if gPaybutton.tag == indexPath?.row {
}
}
}
// 第二步
在你的ViewController里面
class ViewController: UIViewController {
@IBOutlet weak var tableview: UITableView!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
}
extension ViewController: UITableViewDataSource, UITableViewDelegate, CellDelagate {
func addPressed(_ cell: TableViewCell) {
cell.count += 1
cell.proceesingLabel.text = "Processing : \(cell.count)"
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return yourArray.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "TableViewCell")! as? TableViewCell//1.
cell?.indexLabel?.text = yourArray[indexPath.row] //2.
cell?.cellDelagate = self //3.
cell?.tag = indexPath.row //4.
return cell! //5.
}
}
这是因为您正在为班级中的所有单元格使用全局变量,如果您想为每个单元格保持不同的计数,您必须为每个单元格使用不同的变量,我只是给你方向,所以找到你的方式.
为您的计数创建一个数据源并使用您用于表的相同数据源对其进行初始化,假设您有一个用于 tableview 数据源的字符串数组,让我们创建包含您的标题的列表数组并用它计数。 我在我的解决方案中为您提供了所有步骤,您只需将代码放在正确的位置:-
//Suppose this is your tableivew data source
let list : [String] = ["Title1","Title2","Title3"]
//Make a dictionary that is going to be your datasource of tableview holding the count too
var listDict : [[String:Any]] = []
for item in list{
let dict : [String:Any] = ["title":item,"count":Int(0)]
listDict.append(dict)
}
//Where you are performing the actions like in didSelectRow or in any button
let cellIndexPath : IndexPath = IndexPath(item: 0, section: 0) // Get the indexPath of Cell
//Update the count
if let count = listDict[cellIndexPath.row]["count"] as? Int{
let newValue = count + 1
listDict[cellIndexPath.row]["count"] = newValue
}
//Reload the tableview cell of update manually your choice I'm reloading the cell for short
let tableview = UITableView()
tableview.reloadRows(at: [cellIndexPath], with: .fade)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.