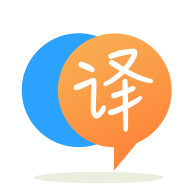
[英]How Can I Enable Sorting/Filtering on XSSFTable Columns Using Apache POI?
[英]How to create a XSSFTable in Apache POI
我正在尝试使用 Java 和 Apache POI 库创建一个包含 Excel 表格的 Excel 表格,但我无法获得 Microsoft Excel 2016 (Office 365) 可读的结果文件。 这是我的代码:
import org.apache.poi.ss.util.AreaReference;
import org.apache.poi.ss.util.CellReference;
import org.apache.poi.xssf.usermodel.XSSFRow;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
class Scratch {
public static void main(String[] args) throws IOException {
XSSFWorkbook workbook = new XSSFWorkbook();
XSSFSheet sheet = workbook.createSheet("Table Sheet");
XSSFRow row0 = sheet.createRow(0);
row0.createCell(0).setCellValue("#");
row0.createCell(1).setCellValue("Name");
XSSFRow row1 = sheet.createRow(1);
row1.createCell(0).setCellValue("1");
row1.createCell(1).setCellValue("Foo");
XSSFRow row2 = sheet.createRow(2);
row2.createCell(0).setCellValue("2");
row2.createCell(1).setCellValue("Bar");
AreaReference area = workbook.getCreationHelper().createAreaReference(
new CellReference(row0.getCell(0)),
new CellReference(row2.getCell(1))
);
sheet.createTable(area);
try(FileOutputStream file = new FileOutputStream(new File("workbook.xlsx"))) {
workbook.write(file);
}
}
}
代码运行良好,但是当我在 Excel 中打开输出文件时,我收到一条消息,指出该文件具有不可读的内容。
我试过运行官方示例,结果是一样的。 官方示例可以在这里找到: https : //svn.apache.org/repos/asf/poi/trunk/src/examples/src/org/apache/poi/xssf/usermodel/examples/CreateTable.java
我需要知道获得可读表格所需的最少代码。
我在 Windows 10 上使用 Apache POI 4.0.0 版和 Oracle JavaSE JDK 1.8.0_172。
总是不确定“官方示例”代码会发生什么。 他们似乎甚至没有经过测试。
CreateTable
使用XSSFTable table = sheet.createTable(reference);
这将创建一个表,该表具有从区域参考中给出的 3 列。 但是所有这些的id都是1,所以我们需要修复。 当然,那时不应再次创建列。
所以修复的示例代码将是:
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.util.AreaReference;
import org.apache.poi.ss.util.CellReference;
import org.apache.poi.xssf.usermodel.XSSFCell;
import org.apache.poi.xssf.usermodel.XSSFRow;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFTable;
import org.apache.poi.xssf.usermodel.XSSFTableStyleInfo;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
/**
* Demonstrates how to create a simple table using Apache POI.
*/
public class CreateTable {
public static void main(String[] args) throws IOException {
try (Workbook wb = new XSSFWorkbook()) {
XSSFSheet sheet = (XSSFSheet) wb.createSheet();
// Set which area the table should be placed in
AreaReference reference = wb.getCreationHelper().createAreaReference(
new CellReference(0, 0), new CellReference(2, 2));
// Create
XSSFTable table = sheet.createTable(reference); //creates a table having 3 columns as of area reference
// but all of those have id 1, so we need repairing
table.getCTTable().getTableColumns().getTableColumnArray(1).setId(2);
table.getCTTable().getTableColumns().getTableColumnArray(2).setId(3);
table.setName("Test");
table.setDisplayName("Test_Table");
// For now, create the initial style in a low-level way
table.getCTTable().addNewTableStyleInfo();
table.getCTTable().getTableStyleInfo().setName("TableStyleMedium2");
// Style the table
XSSFTableStyleInfo style = (XSSFTableStyleInfo) table.getStyle();
style.setName("TableStyleMedium2");
style.setShowColumnStripes(false);
style.setShowRowStripes(true);
style.setFirstColumn(false);
style.setLastColumn(false);
style.setShowRowStripes(true);
style.setShowColumnStripes(true);
// Set the values for the table
XSSFRow row;
XSSFCell cell;
for (int i = 0; i < 3; i++) {
// Create row
row = sheet.createRow(i);
for (int j = 0; j < 3; j++) {
// Create cell
cell = row.createCell(j);
if (i == 0) {
cell.setCellValue("Column" + (j + 1));
} else {
cell.setCellValue((i + 1.0) * (j + 1.0));
}
}
}
// Save
try (FileOutputStream fileOut = new FileOutputStream("ooxml-table.xlsx")) {
wb.write(fileOut);
}
}
}
}
顺便说一句:我从How to insert a table in ms excel using apache java poi中的代码也可以使用apache poi 4.0.0
。 sheet.createTable()
已弃用。 所以使用XSSFTable table = sheet.createTable(null);
反而。 因为该区域以及所有其他事物都是使用低级类设置的。 不过,代码并不比新示例多。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.