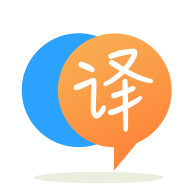
[英]How would you go about scanning a file in java and counting the number of alphanumerical characters and vowels of that file?
[英]How to find the number of characters, vowels and lines in a file using java?
该程序接受一个输入,然后询问用户是否想输入更多。 如果是,则给定的输入存储在下一行。
我编写的以下代码显示除字符数外的所有内容为零。
有人可以帮忙吗。
import java.io.*;
import java.util.*;
public class Ex_3 {
public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
Scanner sc2 = new Scanner(System.in);
try
{
FileOutputStream fout = new FileOutputStream("file4.txt");
FileInputStream fin = new FileInputStream("file4.txt");
while(true)
{
System.out.println("Enter info in file:");
String s = sc.nextLine();
fout.write(s.getBytes());
System.out.println("Would you like to enter more data?");
char c = sc2.next().charAt(0);
if(c=='n')
{
break;
}
fout.write("\r\n".getBytes());
}
fout.close();
int chars=0,vowels=0,lines=0,words=0;
int i=0;
while((i=fin.read())!=-1)
{
if((char)i!='\n'||(char)i!=' ')
{
chars++;
}
else if((char)i==' ')
{
words++;
}
else if((char)i+48=='a'||(char)i+48=='e'||(char)i+48=='i'||
(char)i+48 =='o'||(char)i+48=='u')
{
vowels++;
}
else if((char)i=='\n')
{
lines++;
}
}
System.out.println("Number of characters:"+chars);
System.out.println("Number of vowels:"+vowels);
System.out.println("Number of words:"+words);
System.out.println("Number of lines:"+lines);
fin.close();
sc.close();
sc2.close();
}
catch(Exception e)
{
System.out.println(e);
}
}
}
我查看了您的代码并进行了一些更改和注释。 其中有一些错误。
//Use concrete imports!
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.Scanner;
public class Main {
public static void main(String args[])
{
//You only need one scanner
Scanner sc = new Scanner(System.in);
try
{
FileOutputStream fout = new FileOutputStream("file4.txt");
FileInputStream fin = new FileInputStream("file4.txt");
while(true)
{
System.out.println("Enter info in file:");
fout.write(sc.nextLine().getBytes());
//sometimes the line break is not recognized from the shell
fout.write('\n');
System.out.println("Would you like to enter more data?");
if( sc.nextLine().charAt(0) == 'n' )
{
break;
}
}
fout.close();
sc.close();
int chars=0,vowels=0,lines=0,words=2;
int chars=0,vowels=0,lines=0,words=1;
// With fin.read() you read 32 Bit integers from your input stream,
// which means that you have 4 chars "boxed" in one integer.
int sizeOfStaticArray = 1024;
//(ugly solution btw)
byte[] arr = new byte[sizeOfStaticArray];
fin.read(arr);
byte i;
for( int j = 0; j < sizeOfStaticArray; j++)
{
i = (byte)arr[j];
if(i == 0)
break;
//You don't need casts to char
if( i != '\n' && i != ' ')
{
chars++;
}
//not else if. This is the main issue
if( i == ' ' )
{
words++;
}
else if(i == 'a'||i =='e'|| i == 'i'|| i =='o' || i == 'u')
{
vowels++;
}
else if( i == '\n' )
{
lines++;
//increment word because the start of a line has no space
words++;
}
}
fin.close();
System.out.println("Number of characters:"+chars);
System.out.println("Number of vowels:"+vowels);
System.out.println("Number of words:"+words);
System.out.println("Number of lines:"+lines);
}
catch(Exception e)
{
System.out.println(e);
}
}
}
输入:
Enter info in file:
aaaaa hhhhh zzzzz eeeee
Would you like to enter more data?
y
Enter info in file:
uuuuu ggggg ggggg iiiii
Would you like to enter more data?
n
Number of characters:40
Number of vowels:20
Number of words:8
Number of lines:2
希望有帮助,祝你好运!
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.