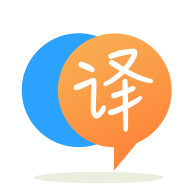
[英]React.JS Typescript - OnChange says "A component is changing a controlled input of type text to be uncontrolled in OnChange" for State Object
[英]React.js when using non state variable Getting error as A component is changing an uncontrolled input of type text to be controlled
我正在尝试使用非状态变量' newItem '来保存输入值
import React, { Component } from 'react';
class List extends Component {
constructor() {
super();
this.state = { items: [1, 2] };
this.newItem = undefined;
}
changeNewItem = e => {
this.newItem = e.target.value;
console.log(this.newItem);
};
addItem = e => {
if (e.keyCode !== 13) return;
var tmp_list = this.state.items;
tmp_list.push(this.newItem);
this.setState({ items: tmp_list }, () => {
this.newItem = '';
});
};
render() {
return (
<div>
<ul>
{this.state.items.map(item => (
<li key={item}>{item}</li>
))}
</ul>
<input
type="text"
placeholder="add item"
value={this.newItem}
onChange={this.changeNewItem}
onKeyUp={this.addItem}
/>
</div>
);
}
}
export default List;
当我在文本框中按Enter键时,该项将添加到数组中,但出现如下错误
index.js:1452警告:组件正在更改要控制的文本类型的不受控制的输入。 输入元素不应从不受控制切换为受控制(反之亦然)。 确定在组件的使用寿命期间使用受控或不受控制的输入元素。 更多信息:在App的列表(App.js:9)的div(List.js:23)的div输入中(List.js:29)(src / index.js:8)
您遇到的问题是,输入元素的初始值未定义,然后使用变量this.newItem
对其进行控制。 因此,您会收到警告,试图将不受控制的输入更改为受控制。
将值初始化为empty string
而不是undefined
。 另外,如果您希望输入组件更改值,请使用state而不是class变量
import React, { Component } from 'react';
class List extends Component {
constructor() {
super();
this.state = { items: [1, 2], newItem: '' };
}
changeNewItem = e => {
this.setState({newItem: e.target.value})
};
addItem = e => {
if (e.keyCode !== 13) return;
var tmp_list = this.state.items;
tmp_list.push(this.state.newItem);
this.setState({ items: tmp_list }, () => {
this.state.newItem = '';
});
};
render() {
return (
<div>
<ul>
{this.state.items.map(item => (
<li key={item}>{item}</li>
))}
</ul>
<input
type="text"
placeholder="add item"
value={this.state.newItem}
onChange={this.changeNewItem}
onKeyUp={this.addItem}
/>
</div>
);
}
}
export default List;
更改为不受控制的输入,并使用Comment建议的usedReact.createRef()
import React, { Component } from 'react';
class List extends Component {
newItem;
constructor() {
super();
this.state = { items: [1, 2] };
this.input = React.createRef();
}
changeNewItem = e => {
this.newItem = e.target.value;
console.log(this.newItem);
};
addItem = e => {
if (e.keyCode !== 13 || !this.newItem) return;
var new_list = this.state.items.concat(this.newItem);
this.setState({ items: new_list }, () => {
this.newItem = '';
this.input.current.value = '';
});
};
render() {
return (
<div>
<ul>
{this.state.items.map(item => (
<li key={item}>{item}</li>
))}
</ul>
<input
type="text"
placeholder="add item"
ref={this.input}
onChange={this.changeNewItem}
onKeyUp={this.addItem}
/>
</div>
);
}
}
export default List;
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.