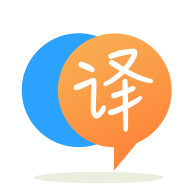
[英]Android- Unable to download image to external storage from url using json and Picasso
[英]Save Image in External Storage By using Picasso from Url , how to download image and show it in imageview
请使用此代码通过使用 Url 将图像保存在外部存储中
//Please Put your Image url In $url
Picasso.get().load($url).into(object : Target{
override fun onPrepareLoad(placeHolderDrawable: Drawable?) {
}
override fun onBitmapFailed(e: java.lang.Exception?, errorDrawable: Drawable?) {
TODO("not implemented") //To change body of created functions use File | Settings | File Templates.
}
override fun onBitmapLoaded(bitmap: Bitmap?, from: Picasso.LoadedFrom?) {
try {
val root = Environment.getExternalStorageDirectory().toString()
var myDir = File("$root")
if (!myDir.exists()) {
myDir.mkdirs()
}
val name = Date().toString() + ".jpg"
myDir = File(myDir, name)
val out = FileOutputStream(myDir)
bitmap?.compress(Bitmap.CompressFormat.JPEG, 90, out)
out.flush()
out.close()
} catch (e: Exception) {
// some action
}
}
})
和图像将保存在 sdcard 中。
使用此方法下载内存中的图像
// DownloadImage AsyncTask
private class DownloadImage extends AsyncTask<String, Void, Bitmap> {
@Override
protected void onPreExecute() {
super.onPreExecute();
}
@Override
protected Bitmap doInBackground(String... URL) {
String imageURL = URL[0];
Bitmap bitmap = null;
try {
// Download Image from URL
InputStream input = new java.net.URL(imageURL).openStream();
// Decode Bitmap
bitmap = BitmapFactory.decodeStream(input);
} catch (Exception e) {
e.printStackTrace();
}
return bitmap;
}
@Override
protected void onPostExecute(Bitmap result) {
if (result != null) {
File destination = new File(getActivity().getCacheDir(),
"profile" + ".jpg");
try {
destination.createNewFile();
ByteArrayOutputStream bos = new ByteArrayOutputStream();
result.compress(Bitmap.CompressFormat.PNG, 0 /*ignored for PNG*/, bos);
byte[] bitmapdata = bos.toByteArray();
FileOutputStream fos = new FileOutputStream(destination);
fos.write(bitmapdata);
fos.flush();
fos.close();
selectedFile = destination;
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
并像这样调用这个方法
Picasso.get().load(response.body()?.url).into(object : Target{
override fun onPrepareLoad(placeHolderDrawable: Drawable?) {
}
override fun onBitmapFailed(e: java.lang.Exception?, errorDrawable: Drawable?) {
}
override fun onBitmapLoaded(bitmap: Bitmap?, from: Picasso.LoadedFrom?) {
new DownloadImage().execute("url_here);
}
})
注意:- 复制此代码并粘贴,
Kotlin
转换器会自动将其转换为Kotlin
1- 添加到 AndroidManifest.xml
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
2- 使用此方法从 Url 使用 Picasso 下载图像:
private static void SaveImage(final Context context, final String MyUrl){
final ProgressDialog progress = new ProgressDialog(context);
class SaveThisImage extends AsyncTask<Void, Void, Void> {
@Override
protected void onPreExecute() {
super.onPreExecute();
progress.setTitle("Processing");
progress.setMessage("Please Wait...");
progress.setCancelable(false);
progress.show();
}
@Override
protected Void doInBackground(Void... arg0) {
try{
File sdCard = Environment.getExternalStorageDirectory();
@SuppressLint("DefaultLocale") String fileName = String.format("%d.jpg", System.currentTimeMillis());
File dir = new File(sdCard.getAbsolutePath() + "/savedImageName");
dir.mkdirs();
final File myImageFile = new File(dir, fileName); // Create image file
FileOutputStream fos = null;
try {
fos = new FileOutputStream(myImageFile);
Bitmap bitmap = Picasso.get().load(MyUrl).get();
bitmap.compress(Bitmap.CompressFormat.JPEG, 100, fos);
Intent intent = new Intent(Intent.ACTION_MEDIA_SCANNER_SCAN_FILE);
intent.setData(Uri.fromFile(myImageFile));
context.sendBroadcast(intent);
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}catch (Exception e){
}
return null;
}
@Override
protected void onPostExecute(Void result) {
super.onPostExecute(result);
if(progress.isShowing()){
progress.dismiss();
}
Toast.makeText(context, "Saved", Toast.LENGTH_SHORT).show();
}
}
SaveThisImage shareimg = new SaveThisImage();
shareimg.execute();
}
3-如何使用,只需调用:
SaveImage(context, "Image URL");
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.