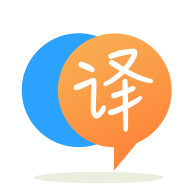
[英]How to create a doubly circular linked list with a head and tail pointer in C?
[英]Doubly linked list in C: Why do I have to explicitly reassign head and tail even when they are not changed?
我有一个双向链表,您可以在其中通过指向头或尾节点的指针的指针添加到头或尾。
这样,您可以将头和尾指针更新为最新的头或尾节点的地址。
我有那些在其自己的函数中启动并存储在同时包含两者的结构中的“指针指针”。
当我添加到尾巴或头部时,必须显式保存旧的头部并重新分配,而尾巴则相反。 否则,结构会发生变异,并且头部也变为尾部,或者尾部变为头部。
我试图了解发生了什么。 也许头/尾巴保持结构必须静态/全局定义?
资料来源:
#include <stdio.h>
#include <stdlib.h>
typedef struct dcl_node {
char *content;
struct dcl_node *next;
struct dcl_node *prev;
} Node;
Node *create_node (char *content) {
Node *n = malloc(sizeof(Node));
n->content = content;
n->next = NULL;
n->prev = NULL;
return n;
}
typedef struct dc_list {
struct dcl_node **head;
struct dcl_node **tail;
} DCList ;
DCList *init_list (char *content_head, char *content_tail) {
Node *head = create_node(content_head);
Node *tail = create_node(content_tail);
head->next = tail;
tail->prev = head;
DCList *list = malloc(sizeof(DCList));
list->head = &head;
list->tail = &tail;
return list;
}
void insert_head (char *content, DCList *list) {
Node *old_head = *list->head;
Node *old_tail = *list->tail; // note the saving here
Node *node = create_node(content);
node->next = old_head;
old_head->prev = node;
*list->head = node;
*list->tail = old_tail; // and reassigning here
}
void insert_tail (char *content, DCList *list) {
Node *old_head = *list->head; // note the saving here
Node *old_tail = *list->tail;
Node *node = create_node(content);
node->prev = old_tail;
old_tail->next = node;
*list->head = old_head; // and reassigning here
*list->tail = node;
}
int main (int argc, char *argv[]) {
DCList *list = init_list("c", "d");
insert_head("b", list);
// If I don't explicitly save and reassign the tail node,
// in this case both head and tail would become the "b node".
printf("head: %s\ntail: %s\n",
(*list->head)->content, (*list->tail)->content);
return 0;
}
因为list->head
和list->tail
是init_list
函数中指向局部变量的指针。
当init_list
返回时,这些变量将被销毁,并且可以重复使用存储在其中的内存。
碰巧的是,当将它们保存在insert_head
和insert_tail
保存的head
和tail
变量(可能是!)将获得相同的内存地址,因此不会被覆盖。 否则,旧的head
可能会被node
覆盖。
这是一个大概的解释-很难说出编译器实际上在做什么。 但是关键是init_list
返回时head
和tail
被破坏,然后您的list->head
和list->tail
指向可以重用的空闲内存。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.