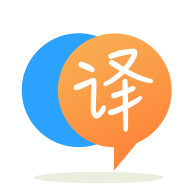
[英]How to implement this in functional programming using Function<T,R> in Java
[英]Java: How to properly manipulate BigDecimal array using functional programming?
那么,如何使用函数式编程获得此代码的结果:
public static final List<BigDecimal> numbers = Arrays.asList(
new BigDecimal("15"), new BigDecimal("10"), new BigDecimal("17"),
new BigDecimal("30"), new BigDecimal("18"), new BigDecimal("23"),
new BigDecimal("5"), new BigDecimal("12") );
BigDecimal totalOfReducedNumbers = BigDecimal.ZERO;
for(BigDecimal number : numbers) {
if(number.compareTo(BigDecimal.valueOf(20)) > 0)
totalOfReducedNumbers =
totalOfReducedNumbers.add(number.multiply(BigDecimal.valueOf(0.9)));
}
System.out.println("Total of reduced numbers: " + totalOfReducedNumbers);
其中抛出“ 总数减少:47.7 ”
如何使用 map(),reduce()等函数式编程工具获得相同的结果 ?
通过执行相同的操作,首先过滤值(您只需要大于20的值)。 然后将这些值乘以0.9
,最后通过执行加法来减少项。 喜欢,
BigDecimal TWENTY = BigDecimal.valueOf(20);
BigDecimal POINT9 = BigDecimal.valueOf(0.9);
System.out.println("Total of reduced numbers: " + numbers.stream()
.filter(x -> x.compareTo(TWENTY) > 0)
.map(x -> x.multiply(POINT9)).reduce((a, b) -> a.add(b)).get());
产出(按要求)
Total of reduced numbers: 47.7
并且,正如评论中所建议的那样,我们可以通过方法引用进一步改进,并且使用orElse
比原始get()
更安全。 喜欢,
System.out.println("Total of reduced numbers: " + numbers.stream()
.filter(x -> x.compareTo(TWENTY) > 0)
.map(x -> x.multiply(POINT9))
.reduce(BigDecimal::add)
.orElse(BigDecimal.ZERO));
for循环实现是典型的“reduce”模式(在其他一些语言中也称为“fold”,“Aggregate”模式)
你正在寻找filter
- > reduce
方法:
BigDecimal reduce =
numbers.stream()
.filter(n -> n.compareTo(BigDecimal.valueOf(20)) > 0)
.reduce(BigDecimal.ZERO,
(a, b) -> a.add(b.multiply(BigDecimal.valueOf(0.9))));
您可以通过缓存BigDecimal.valueOf(20)
和BigDecimal.valueOf(0.9)
来进一步减少构造的对象数量,如@ Elliot的答案所示,即:
BigDecimal TWENTY = BigDecimal.valueOf(20);
BigDecimal POINT9 = BigDecimal.valueOf(0.9);
BigDecimal reduce =
numbers.stream()
.filter(n -> n.compareTo(TWENTY) > 0)
.reduce(BigDecimal.ZERO,
(a, b) -> a.add(b.multiply(POINT9)));
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.