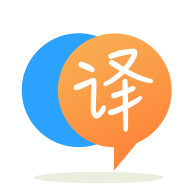
[英]Deserializing JSON using spring boot into a POJO with a list of generic objects
[英]Spring Boot - deserializing Object inside list of objects
我有一个问题。 在问题对象内部,我有主题列表。 当我尝试发布对此控制器的请求时,我的主题列表为空。 我试图验证摇摇欲坠的用户界面,我看不到列表中的任何参数。
Question.java
import java.io.Serializable;
import java.util.Date;
import java.util.List;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.JoinTable;
import javax.persistence.ManyToMany;
import javax.persistence.OneToMany;
import javax.persistence.OneToOne;
import javax.validation.constraints.NotNull;
import org.hibernate.annotations.CreationTimestamp;
@Entity
public class Question implements Serializable {
@Id
@GeneratedValue(strategy=GenerationType.SEQUENCE)
@Column(name="questionId")
private Integer questionId;
@NotNull
@Column(name="title")
private String title;
@NotNull
@Column(name="body")
private String body;
@CreationTimestamp
private Date createdAt;
@CreationTimestamp
private Date modifiedAt;
@OneToOne
@JoinColumn(name="userId")
private User userId;
@OneToMany(cascade=CascadeType.ALL)
@JoinColumn(name="question_id")
private List<Comment> commentList;
@ManyToMany(cascade=CascadeType.ALL)
@JoinTable(name="Question_topic",
joinColumns=@JoinColumn(name="question_id"),
inverseJoinColumns=@JoinColumn(name="topic_id"))
private List<Topic> topicList;
/**
* @return the questionId
*/
public Integer getQuestionId() {
return questionId;
}
/**
* @param questionId the questionId to set
*/
public void setQuestionId(Integer questionId) {
this.questionId = questionId;
}
/**
* @return the title
*/
public String getTitle() {
return title;
}
/**
* @param title the title to set
*/
public void setTitle(String title) {
this.title = title;
}
/**
* @return the body
*/
public String getBody() {
return body;
}
/**
* @param body the body to set
*/
public void setBody(String body) {
this.body = body;
}
/**
* @return the createdAt
*/
public Date getCreatedAt() {
return createdAt;
}
/**
* @param createdAt the createdAt to set
*/
public void setCreatedAt(Date createdAt) {
this.createdAt = createdAt;
}
/**
* @return the modifiedAt
*/
public Date getModifiedAt() {
return modifiedAt;
}
/**
* @param modifiedAt the modifiedAt to set
*/
public void setModifiedAt(Date modifiedAt) {
this.modifiedAt = modifiedAt;
}
/**
* @return the userId
*/
public User getUserId() {
return userId;
}
/**
* @param userId the userId to set
*/
public void setUserId(User userId) {
this.userId = userId;
}
/**
* @return the commentList
*/
public List<Comment> getCommentList() {
return commentList;
}
/**
* @param commentList the commentList to set
*/
public void setCommentList(List<Comment> commentList) {
this.commentList = commentList;
}
public List<Topic> getTopicList() {
return topicList;
}
public void setTopicList(List<Topic> topicList) {
this.topicList = topicList;
}
}
Topic.java
import java.io.Serializable;
import java.util.Date;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.OneToOne;
import org.hibernate.annotations.CreationTimestamp;
@Entity公共类Topic实现了Serializable {
/**
*
*/
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy=GenerationType.SEQUENCE)
@Column
private Integer topicId;
@Column
private String topicName;
@Column
private String topicDesc;
@CreationTimestamp
private Date createdAt;
@OneToOne
@JoinColumn(name="userId",insertable=false,updatable=false)
private User userId;
/**
* @return the topicId
*/
public Integer getTopicId() {
return topicId;
}
/**
* @param topicId the topicId to set
*/
public void setTopicId(Integer topicId) {
this.topicId = topicId;
}
/**
* @return the topicName
*/
public String getTopicName() {
return topicName;
}
/**
* @param topicName the topicName to set
*/
public void setTopicName(String topicName) {
this.topicName = topicName;
}
/**
* @return the topicDesc
*/
public String getTopicDesc() {
return topicDesc;
}
/**
* @param topicDesc the topicDesc to set
*/
public void setTopicDesc(String topicDesc) {
this.topicDesc = topicDesc;
}
/**
* @return the createdAt
*/
public Date getCreatedAt() {
return createdAt;
}
/**
* @param createdAt the createdAt to set
*/
public void setCreatedAt(Date createdAt) {
this.createdAt = createdAt;
}
/**
* @return the userId
*/
public User getUserId() {
return userId;
}
/**
* @param userId the userId to set
*/
public void setUserId(User userId) {
this.userId = userId;
}
}
春季靴类
@RestController
@RequestMapping("/qna/")
public class controller{
public ResponseEntity<?> postQuestion(@RequestBody Question){
}
}
Swagger-UI请求的对象
{
"title": "abc",
"topicList": []
}
预期要求
{
"title": "abc",
"topicList": [
{
"topicName": "topic1",
"createdAt": ""
}
]
}
在每个字段上都缺少getter / setter方法。
@Entity
public class Question implements Serializable {
private String title;
private List<Topic> topicList;
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public List<Topic> getTopicList() {
return topicList;
}
public void setTopicList(List<Topic> topicList) {
this.topicList = topicList;
}
}
public class Topic implements Serializable {
private String topicName;
private Date createdAt;
public String getTopicName() {
return topicName;
}
public void setTopicName(String topicName) {
this.topicName = topicName;
}
public Date getCreatedAt() {
return createdAt;
}
public void setCreatedAt(Date createdAt) {
this.createdAt = createdAt;
}
}
您只是没有getter和setter方法,因此,如果要在Spring MVC中返回数据,并且要传输自己定义的对象,则应该添加getter和setter方法进行注入进入春季MVC,就像那样
@Entity
public class Question implements Serializable {
private String title;
private List<Topic> topicList;
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public List<Topic> getTopicList() {
return topicList;
}
public void setTopicList(List<Topic> topicList) {
this.topicList = topicList;
}
}
你理解吗?
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.