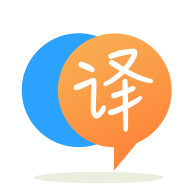
[英]How would I transfer these characters down the alphabet 3 spaces without going into other characters that aren't apart of the Alphabet?
[英]How do I modify this program to count the number of characters that aren't spaces?
对于我的作业,我需要修改以下程序。 我不能使用strings.h。
int main(void)
{
int c, countSpaces = 0;
printf("Type sentence:\n");
do
{
c = getchar();
if (c == ' ')
countSpaces = countSpaces + 1;
}
while (c != '\n');
printf("Sentence contains %d Spaces.\n", countSpaces);
return 0;
}
我尝试使用
if (c != EOF)
countSpaces = countSpaces + 1;
}
while (c != '\n');
printf("Sentence contains %d Spaces.\n", countSpaces - 1);
但这似乎是一种古怪而毫不含糊的方法。 谁能帮助我和/或向我解释如何做得更好?
提前致谢
我发布的代码对句子中的空格进行计数,我想对其进行修改以对输入句子中的所有字符进行计数。 – fbN 21秒前
在if
条件之外还有另一个计数器。
#include <stdio.h>
int main(void)
{
int c;
int countSpaces = 0;
int countChars = 0;
puts("Type sentence:");
do {
c = getchar();
countChars += 1;
if (c == ' ') {
countSpaces += 1;
}
} while (c != '\n');
printf("Sentence contains %d spaces and %d characters.\n", countSpaces, countChars);
return 0;
}
两个笔记。 foo += 1
是foo = foo + 1
简写,没有foo++
复杂性。
无阻塞if
或while
是在玩火。 最终,您会不小心将其写成。
if( condition )
do something
whoops this is not in the condition but it sure looks like it is!
始终使用块形式。
$ ./test
Type sentence:
foo bar baz
Sentence contains 2 spaces and 12 characters.
注意这是12,因为它包括换行符。 那是因为它在计算c
之后会检查c
是什么。 您可以通过将c
读为来解决此问题。 这是一个相当普通的“读取和检查” C循环习惯用法。
// Note, the parenthesis around `c = getchar()` are important.
while( (c = getchar()) != '\n' ) {
countChars++;
if (c == ' ') {
countSpaces++;
}
}
$ ./test
Type sentence:
foo bar baz
Sentence contains 2 spaces and 11 characters.
我编写这段代码来计算给定字符串的长度,就像strlen
函数一样。 我只用了scanf,即使在有空格的情况下,它也能完美工作。
#include <stdio.h>
int main()
{
char *str = calloc(sizeof(char),50);
int i = 0, count = 0;
printf("Type sentence:\n");
scanf("%[^\n]",str);
while (str[i++] != '\0')
count++; //length of the string
printf("%d",count);
return 0;
}
如果只想计算给定字符串中的字符,请使用以下代码:
#include <stdio.h>
int main()
{
char *str = calloc(sizeof(char),50);
int count = 0;
printf("Type sentence:\n");
scanf("%[^\n]",str);
for (int i = 0; str[i] != '\0'; i++)
if ((str[i] >= 'A' && str[i] <= 'Z') || (str[i] >= 'a' && str[i] <= 'z'))
count++;
printf("Sentence contains %d characters.\n",count);
return 0;
}
输出 :
Type sentence:
hello world
Sentence contains 10 characters.
从控制台( stdin
)读取一行时,我总是喜欢使用fgets()
):
#include <stdio.h>
int main(void)
{
int i;
int length = 0;
char buffer[1024];
printf( "Enter some text> " );
fgets( buffer, sizeof(buffer), stdin );
// If the user inputs > 1024 letters, buffer will not be \n terminated
for ( i=0; buffer[i] != '\n' && buffer[i] != '\0'; i++ )
{
length += 1;
}
printf( "length: %d\n", length );
return 0;
}
你可以像这样计算结果
int main(void)
{
int c, countSpaces = 0;
printf("Type sentence:\n");
do
{
c = getchar();
if (c == ' ')
countSpaces++;
}
while (c != '\n');
int countChar = c - countSpaces - 1 ; // -1 new line
printf("Sentence contains %d Spaces.\n", countSpaces);
printf("Sentence contains %d chars.\n", countChar);
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.