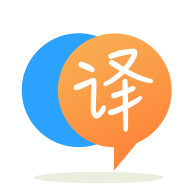
[英]Why can I not use fscanf() to read consecutive characters from a file?
[英]Can't I use fscanf() for infinite time?
#include <stdio.h>
main() {
int n;
FILE *file;
printf("We are here to create a file!\n");
file = fopen("demo.txt", "w+");
if (file != NULL)
printf("Succesfully opened!");
printf("Enter number\n");
while (scanf("%d", &n)) {
fprintf(file, "%d", n);
}
fclose(file);
}
为什么fscanf()
在这里不起作用? 在这里scanf
正常工作,但是fscanf()
在这里没有响应或无法工作。 谁能解释这个问题是什么?
您的代码有一些问题:
main
的原型是int main(void)
fopen
返回NULL
则将具有未定义的行为,因为您稍后将此空指针传递给fprintf
。 scanf()
返回0
。 相反,您应该在scanf()
返回1
进行迭代。 scanf()
在文件末尾失败,则将返回EOF
,从而导致无限循环。 fprintf()
的数字之后输出分隔符,否则所有数字都将聚集在一起,形成一个长数字序列。 main()
应该返回0
或错误状态 这是更正的版本:
#include <stdio.h>
int main(void) {
int n;
FILE *file;
printf("We are here to create a file\n");
file = fopen("demo.txt", "w");
if (file != NULL) {
printf("Successfully opened\n");
} else {
printf("Cannot open demo.txt\n");
return 1;
}
printf("Enter numbers\n");
while (scanf("%d", &n) == 1) {
fprintf(file, "%d\n", n);
}
fclose(file);
return 0;
}
关于您的问题: 为什么我不能使用fscanf()
而不是scanf()
?
fscanf()
,只要为其提供打开的流指针以供读取:如果在while (fscanf(stdin, "%d", &n) == 1)
编写程序,则程序的行为将相同。 fscanf()
从file
读取,则需要在读写操作之间执行文件定位命令,例如fseek()
rewind()
fseek()
。 但是,如果在文件的当前位置没有要读取的数字,则fscanf()
将会失败,并且由于以"w+"
模式打开file
,因此fopen()
将被截断。 通过将数字写入文件,将其倒带到开头并重新读取相同的数字等方法,可以导致无限循环。
这是一些代码说明:
#include <stdio.h>
int main(void) {
int n;
FILE *file;
printf("We are here to create a file\n");
file = fopen("demo.txt", "w+");
if (file != NULL) {
printf("Successfully opened\n");
} else {
printf("Cannot open demo.txt\n");
return 1;
}
printf("Enter a number: ");
if (scanf("%d", &n) == 1) {
fprintf(file, "%d\n", n);
rewind(file);
while (fscanf(file, "%d", &n) == 1) {
printf("read %d from the file\n", n);
if (n == 0)
break;
rewind(file);
fprintf(file, "%d\n", n >> 1);
rewind(file);
}
}
fclose(file);
return 0;
}
相互作用:
We are here to create a file
Successfully opened
Enter a number: 10
read 10 from the file
read 5 from the file
read 2 from the file
read 1 from the file
read 0 from the file
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.