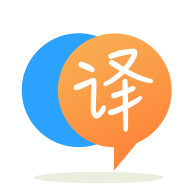
[英]how to merge two arrays to key value pairs with repeated values javascript
[英]How to merge the key-value pairs of two json arrays? - Javascript
我有两个这样的JSON数组。 我想合并它们的键值对。 它们是两者共同的一些项目,而它们是不常见的一些项目。
var jsonData1 = [
{
firstName: "Sam",
age: "10"
},
{
firstName: "John",
age: "11"
},
{
firstName: "Jack",
age: "12"
},
{
firstName: "Pam",
age: "13"
},
{
firstName: "Tom",
age: "14"
},
{
firstName: "Mitch",
age: "15"
}
];
var jsonData2 = [
{
firstName: "Sam",
city: "London"
},
{
firstName: "John",
city: "New York"
},
{
firstName: "Jack",
city: "Paris"
},
{
firstName: "Pam",
city: "Moscow"
},
{
firstName: "Roger",
city: "Shanghai"
},
{
firstName: "Don",
city: "Jakarta"
}
];
您可以在第一个数组中有一些firstName在第二个数组中没有城市。 同样在第二个数组中有一些firstName在第一个数组中没有城市。
我需要将这两个数组合并为一个数组,以防万一firstName没有年龄或城市,它将被分配为”(空白字符串)。
新数组将具有3个字段,有些项目将在2个字段中具有值。 他们有一个字段作为空白字符串。
我想使用Vanilla JS做到这一点,我不想使用Jquery,Lodash和Underscore。
有很多方法可以解决此问题,但是一种选择是基于Array#reduce()
方法,如下所示:
const jsonData1=[{firstName:"Sam",age:"10"},{firstName:"John",age:"11"},{firstName:"Jack",age:"12"},{firstName:"Pam",age:"13"},{firstName:"Tom",age:"14"},{firstName:"Mitch",age:"15"}]; const jsonData2=[{firstName:"Sam",city:"London"},{firstName:"John",city:"New York"},{firstName:"Jack",city:"Paris"},{firstName:"Pam",city:"Moscow"},{firstName:"Roger",city:"Shanghai"},{firstName:"Don",city:"Jakarta"}]; /* Use Array#concat() to combine both input arrays before performing the merge. This will ensure that all items in both arrays are processed and accounted for during the merge (which is important in situations where the input arrays differ in length). Object.values() is used to transform the dictionary resulting from reduce() to an array */ var result = Object.values( [].concat(jsonData1, jsonData2 ).reduce((dict, item) => { /* We use Array#reduce is an intermediate step to construct a dictionary which maps each unique "item.firstName" to a corresponding object that contains the merged (or yet to be merged) data for this first name */ var value = dict[item.firstName] || {} /* Use Object.assign() to merge existing data for "item.firstName" with current item being processed. Also pass default values as first argument to ensure all three key values are present in merge result */ value = Object.assign({ firstName : '', age : '', city : ''} , value, item) /* Update the dictionary with merged data */ dict[item.firstName] = value return dict }, {})) console.log(result);
一种可能的方法:
const jsonData1=[{firstName:"Sam",age:"10"},{firstName:"John",age:"11"},{firstName:"Jack",age:"12"},{firstName:"Pam",age:"13"},{firstName:"Tom",age:"14"},{firstName:"Mitch",age:"15"}]; const jsonData2=[{firstName:"Sam",city:"London"},{firstName:"John",city:"New York"},{firstName:"Jack",city:"Paris"},{firstName:"Pam",city:"Moscow"},{firstName:"Roger",city:"Shanghai"},{firstName:"Don",city:"Jakarta"}]; const result = [].concat(jsonData1, jsonData2).reduce((acc, ele) => { const existing = acc.find(x => x.firstName === ele.firstName); if(!existing) return acc.concat({firstName: ele.firstName, city: ele.city || '', age: ele.age || ''}); Object.keys(ele).forEach(x => existing[x] = ele[x]); return acc; },[]) console.log(result);
我知道您已经接受了答案,但我认为我可以提供替代方案,无论情况好坏。
var jsonData1 = [{firstName: "Sam",age: "10"},{firstName: "John",age: "11"},{firstName: "Jack",age: "12"},{firstName: "Pam",age: "13"},{firstName: "Tom",age: "14"},{firstName: "Mitch",age: "15"}]; var jsonData2 = [{firstName: "Sam",city: "London"},{firstName: "John",city: "New York"},{firstName: "Jack",city: "Paris"},{firstName: "Pam",city: "Moscow"},{firstName: "Roger",city: "Shanghai"},{firstName: "Don",city: "Jakarta"}]; var defaults = {firstName: "", age: "", city: ""}; var data = [ ...jsonData1, ...jsonData2 ]; var names = [ ...new Set(data.map(i=>i.firstName)) ]; var res = names.map(n => data .reduce((acc, jd) => jd.firstName === n ? {...acc, ...jd} : acc, defaults) ); console.log(res);
var data
使用扩展语法 (数组文字)将两个数据数组组合为一个。
var names
使用Set
创建一个唯一名称数组。
map()
遍历名称列表,为每个名称创建一个合并的对象。 合并是使用reduce()
和spread语法 (对象文字)完成的 。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.