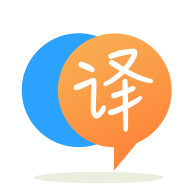
[英]Java Spring form Validator to check whether a input string contains special characters or not
[英]Java Spring form check whether a input string contains special characters or not
我正在尝试创建一个 Java Spring 表单来检查输入字符串是否包含特殊字符。 例如,当用户输入包含特殊字符的字符串时,会弹出一条消息,指出输入字符串包含特殊字符。
我的问题是如何实现java函数来检查输入字符串中的特殊字符?
模型
package com.mkyong.customer.model; public class Customer { String userName; public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } }
控制器
package com.mkyong.customer.controller; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.springframework.validation.BindException; import org.springframework.web.servlet.ModelAndView; import org.springframework.web.servlet.mvc.SimpleFormController; import com.mkyong.customer.model.Customer; public class TextBoxController extends SimpleFormController{ public TextBoxController(){ setCommandClass(Customer.class); setCommandName("customerForm"); } @Override protected ModelAndView onSubmit(HttpServletRequest request, HttpServletResponse response, Object command, BindException errors) throws Exception { Customer customer = (Customer)command; return new ModelAndView("CustomerSuccess","customer",customer); } }
客户表格
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form"%> <html> <head> <style> .error { color: #ff0000; } .errorblock{ color: #000; background-color: #ffEEEE; border: 3px solid #ff0000; padding:8px; margin:16px; } </style> </head> <body> <h2>Java Textbox Special Characters</h2> <form:form method="POST" commandName="customerForm"> <form:errors path="*" cssClass="errorblock" element="div"/> <table> <tr> <td>Keyword:</td> <td><form:input path="userName" /></td> <td><form:errors path="userName" cssClass="error" /></td> </tr> <tr> <td colspan="3"><input type="submit" /></td> </tr> </table> </form:form> </body> </html>
客户成功
<html> <body> <h2>Java Textbox Special Characters</h2> Keyword: ${customer.userName} </body> </html>
您可以拥有所有特殊字符的列表并使用正则表达式进行检查:
Pattern regex = Pattern.compile("[$&+,:;=\\\\?@#|/'<>.^*()%!-]"); // fill in any chars that you consider special
if (regex.matcher(stringToCheck).find()) {
// found
}
您还可以查看Guava's CharMatcher
(没有正则表达式且更具可读性)。 您可以像这样构建自己的匹配逻辑:
boolean isAMatch = CharMatcher.WHITESPACE
.or(CharMatcher.anyOf("[$&+,:;=\\\\?@#|/'<>.^*()%!-]")) // fill in any chars that you consider special
.matchesAnyOf(stringToCheck);
特殊字符的单独转换方法
if(checkSpecialChar("^[^<>{}\"/|;:.,~!?@#$%^=&*\\]\\\\()\\[¿§«»ω⊙¤°℃℉€¥£¢¡®©0-9_+]*$", source){
// add your staff here
}
public boolean checkSpecialChar(String regex, String source) {
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(source);
return matcher.find();
在 Spring 中,您可以像这样获取表单的值:
@RequestMapping(value = "/", method = RequestMethod.POST)
public String submit(@Valid @ModelAttribute("userName")String username){
// And now you can check with the regex pattern from hovanessyan if it contains special characters
Pattern regex = Pattern.compile("[$&+,:;=\\\\?@#|/'<>.^*()%!-]");
if (regex.matcher(username).find()) {
// found
}
}
我希望它有帮助。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.