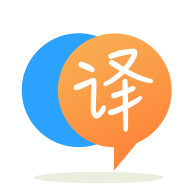
[英]How to test Spring Boot @WebFluxTest with Spring Security @Secured annotation
[英]Spring Boot 2: test secured endpoints
我有一个使用Spring Boot 2构建的项目(数据,休息,安全性...),所以在项目中我有如下的休息控制器:
@RestController
class ProductController {
private final ProductService productService;
private final ProductConverter productConverter;
private final UserService userService;
@Autowired
ProductController(ProductService productService,
ProductConverter productConverter,
UserService userService) {
this.productService = productService;
this.productConverter = productConverter;
this.userService = userService;
}
@GetMapping(EndpointPath.PRODUCTS)
PageableProductsDTO getProducts(Principal principal, Pageable pageable) {
return productService.getUsersProducts(principal.getName(), pageable);
}
@PostMapping(EndpointPath.PRODUCT)
ResponseEntity<?> createNewProduct(@Valid @RequestBody ProductDetailsDto productDetailsDto, Principal principal) {
productService.saveProduct(productConverter.createFrom(productDetailsDto), principal.getName());
return ResponseEntity.status(HttpStatus.CREATED).build();
}
}
以及安全性配置:
@Configuration
@EnableResourceServer
public class ResourceServerConfig extends ResourceServerConfigurerAdapter {
private final RESTLogoutSuccessHandler restLogoutSuccessHandler;
@Autowired
public ResourceServerConfig(RESTLogoutSuccessHandler restLogoutSuccessHandler) {
this.restLogoutSuccessHandler = restLogoutSuccessHandler;
}
@Override
public void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.anonymous()
.disable()
.logout()
.logoutUrl(EndpointPath.SIGN_OUT)
.logoutSuccessHandler(restLogoutSuccessHandler)
.and()
.authorizeRequests()
.antMatchers(GET, EndpointPath.PRODUCTS).authenticated()
.antMatchers(POST, EndpointPath.PRODUCT).authenticated()
.and()
.exceptionHandling().accessDeniedHandler(new OAuth2AccessDeniedHandler());
}
}
我也用这样的单元测试覆盖ProductController :
@ExtendWith({SpringExtension.class, MockitoExtension.class})
@ContextConfiguration(classes = {MessageSourceConfiguration.class})
@MockitoSettings(strictness = Strictness.LENIENT)
class ProductControllerTest {
private MockMvc mockMvc;
@Autowired
private MessageSource messageSource;
@Mock
private ProductService productService;
@Mock
private ProductConverter productConverter;
@Mock
private UserService userService;
@BeforeEach
void setUp() {
mockMvc = MockMvcBuilders.standaloneSetup(new ProductController(productService, productConverter, userService))
.setCustomArgumentResolvers(new PageableHandlerMethodArgumentResolver())
.setControllerAdvice(new ControllerAdvice(messageSource)).build();
}
@Test
void shouldReturnSetOfProducts() throws Exception {
// Given
String authenticatedUserEmail = "email@g.com";
when(productService.getUsersProducts(eq(authenticatedUserEmail), ArgumentMatchers.any(Pageable.class))).thenReturn(new PageableProductsDTO(new PageImpl<>(
List.of(new ProductDetailsDto("1234", "name", 1), new ProductDetailsDto("12345", "name 2", 2)))));
// When - Then
mockMvc.perform(get(EndpointPath.PRODUCTS).principal(() -> authenticatedUserEmail).accept(MediaType.APPLICATION_JSON))
.andExpect(status().isOk())
.andExpect(content().contentType(MediaType.APPLICATION_JSON_UTF8))
.andExpect(jsonPath("$.productDtos.content", hasSize(2)))
.andExpect(jsonPath("$.productDtos.totalPages", is(1)))
.andExpect(jsonPath("$.productDtos.totalElements", is(2)))
.andExpect(jsonPath("$.productDtos.content.[*].barcode", containsInAnyOrder("1234", "12345")))
.andExpect(jsonPath("$.productDtos.content.[*].name", containsInAnyOrder("name", "name 2")))
.andExpect(jsonPath("$.productDtos.content.[*].price", containsInAnyOrder(1, 2)));
}
}
除了该单元测试之外,我还想确保端点EndpointPath.PRODUCTS是安全的。 有人知道如何为此编写单元测试吗? 例如:未认证/授权用户时的预期状态401/403。
完整的Spring Boot集成测试中最简单的测试如下所示
@ExtendWith(SpringExtension.class)
@SpringBootTest
@AutoConfigureMockMvc
@DisplayName("sample boot integration tests")
public class SpringBootIntegrationTests {
@Autowired
private MockMvc mvc;
@DisplayName("pages are secured")
void pageIsSecure() throws Exception {
mvc.perform(
MockMvcRequestBuilders.get("/products")
)
.andExpect(status().is3xxRedirection())
.andExpect(redirectedUrl("http://localhost/login"))
.andExpect(unauthenticated())
;
}
}
如果没有身份验证,通常不会看到401/403。 原因Spring Security会将您重定向到授权服务器。
redirectUrl
将与重定向到授权服务器的内容匹配。
所以可能是这样的
redirectedUrl(containsString("http://authorization.server/oauth/authorize"))
如果您的端点需要令牌,则可以在测试中执行以下操作
.andExpect(status().isUnauthorized())
.andExpect(unauthenticated())
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.