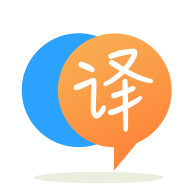
[英]Writing a 2-dimensional array table in C. How would I go about doing this. About neatness (such as dividing lines) as well
[英]How would I reallocate a 2-dimensional array in C?
我正在执行C编程,其中我从用户读取了列和行的数量,并根据给定的输入创建2D数组,并使用随机值填充条目。 如何根据用户输入删除2D数组中的特定列(例如,如果用户要删除第一列,我将如何重新分配矩阵空间?)
#include <stdio.h>
#include <stdlib.h>
double **initializeRandomMatrixPtr(double **a, int rows, int cols) {
a = malloc(rows * sizeof(double *));
for (int i = 0; i < rows; i++) {
*(a + i) = malloc(cols * sizeof(double));
for (int j = 0; j < cols; j++) {
*(*(a + i) + j) = rand();
}
}
return a;
}
void printMatrix(double **matrix, int rows, int cols) {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
printf("%d ", matrix[i][j]);
}
printf("\n");
}
}
void freeMatrix(double **matrix, int rows, int cols) {
for (int i = 0; i < rows; i++) {
free(matrix[i]);
}
free(matrix);
}
int main(void) {
int rows = -1;
int cols = -1;
int deletedColumn = -1;
// remove first column
printf("Enter number of rows:");
scanf("%d", &rows);
printf("Enter number of cols:");
scanf("%d", &cols);
double **matrix = initializeRandomMatrixPtr(matrix, rows, cols);
printMatrix(matrix, rows, cols);
freeMatrix(matrix, rows, cols);
printf("What column do you want to delete?");
scanf("%d", deletedColumn);
realloc(matrix, ) // what should I put for my second parameter?
return 0;
}
因为这是家庭作业,所以我实际上不会张贴任何代码:
要重新分配较小的数组1项(整行或整列),请执行以下操作:
您可能还需要通过至少在指向指针的外部数组中存储条目数来跟踪此数组的状态-防止在打印尺寸时需要阅读超出新尺寸列表的末尾再次或调整其大小或其他。
如果您试图从另一个方向删除条目(每个分配从外部循环中删除一个条目),则过程类似:
访问外循环中的每个条目并执行以下操作:
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.