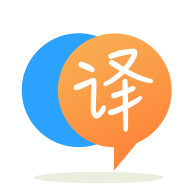
[英]How to display errors from array response after validation from laravel to vue.js (based on the index)
[英]How to display array response in vue.js and laravel?
我想在.vue
文件中显示数组数据,但我不知道该怎么做。 这是我尝试过的:
<template>
<div id="app">
<table class="table table-striped">
<thead>
<tr>
<th>Project Name</th>
<th>Type</th>
</tr>
</thead>
<tfoot>
<tr v-for="user in info">
<th>{{ user.data.user.assigned_projects.name }}</th>
<th>{{ user.data.user.assigned_projects.type }}</th>
</tr>
</tfoot>
</table>
</div>
</template>
<script>
import axios from 'axios'
export default {
el: '#app',
data() {
return {
info: null
}
},
mounted() {
axios
.get('http://api_url')
.then((response) => {
this.info = response.data
})
}
}
</script>
这是一个示例 API 响应:
{
"response":{
"error":{
"error_code":0,
"error_msg":"",
"msg":""
},
"data":{
"user":{
"id":1,
"email":"email@address.com",
"first_name":null,
"last_name":null,
"photo_url":null,
"organisation":null,
"own_projects":[
],
"assigned_projects":[
{
"id":10,
"name":"test project",
"description":"cool stuff",
"image_url":"http://image_url",
"timezone":"UTC",
"owner":15,
"created_by":15,
"created_at":"2019-02-26 16:37:03",
"updated_at":"2019-02-26 16:37:03",
"pivot":{
"user_id":1,
"project_id":10
}
},
{
"id":8,
"name":"test project 2",
"description":"",
"image_url":"",
"timezone":"UTC",
"owner":15,
"created_by":15,
"created_at":"2019-02-21 18:36:55",
"updated_at":"2019-02-21 18:36:55",
"pivot":{
"user_id":1,
"project_id":8
}
}
]
}
}
}
}
通常, v-for
指令采用v-for="item in items"
的形式,其中items
是数组或对象的数据路径。
assign_projects assigned_projects[]
的数据路径是info.response.data.user.assigned_projects
,因此v-for
将是:
<tr v-for="project in info.response.data.user.assigned_projects" :key="project.id">
<th>{{ project.name }}</th>
<th>{{ project.id }}</th>
</tr>
new Vue({ el: '#app', data() { return { info: null } }, mounted() { this.info = { "response":{ "error":{ "error_code":0, "error_msg":"", "msg":"" }, "data":{ "user":{ "id":1, "email":"email@address.com", "first_name":null, "last_name":null, "photo_url":null, "organisation":null, "own_projects":[ ], "assigned_projects":[ { "id":10, "name":"test project", "description":"cool stuff", "image_url":"http://image_url", "timezone":"UTC", "owner":15, "created_by":15, "created_at":"2019-02-26 16:37:03", "updated_at":"2019-02-26 16:37:03", "pivot":{ "user_id":1, "project_id":10 } }, { "id":8, "name":"test project 2", "description":"", "image_url":"", "timezone":"UTC", "owner":15, "created_by":15, "created_at":"2019-02-21 18:36:55", "updated_at":"2019-02-21 18:36:55", "pivot":{ "user_id":1, "project_id":8 } } ] } } } }; } })
table { border-collapse: collapse; width: 100%; } th, td { text-align: left; padding: 8px; } tfoot th { font-weight: normal; } tr:nth-child(even) { background-color: #f2f2f2 }
<script src="https://unpkg.com/vue@2.6.8"></script> <div id="app"> <table> <thead> <tr> <th>Project Name</th> <th>Type</th> </tr> </thead> <tfoot> <tr v-for="project in info.response.data.user.assigned_projects" :key="project.id"> <th>{{ project.name }}</th> <th>{{ project.id }}</th> </tr> </tfoot> </table> </div>
为了简化模板的可读性,此处应考虑计算属性:
// template
<tr v-for="project in projects" :key="project.id">...</tr>
// script
computed: {
projects() {
// empty array if `this.info` is not yet defined
return this.info && this.info.response.data.user.assigned_projects || [];
}
}
new Vue({ el: '#app', data() { return { info: null } }, computed: { projects() { return this.info && this.info.response.data.user.assigned_projects || []; } }, mounted() { this.info = { "response":{ "error":{ "error_code":0, "error_msg":"", "msg":"" }, "data":{ "user":{ "id":1, "email":"email@address.com", "first_name":null, "last_name":null, "photo_url":null, "organisation":null, "own_projects":[ ], "assigned_projects":[ { "id":10, "name":"test project", "description":"cool stuff", "image_url":"http://image_url", "timezone":"UTC", "owner":15, "created_by":15, "created_at":"2019-02-26 16:37:03", "updated_at":"2019-02-26 16:37:03", "pivot":{ "user_id":1, "project_id":10 } }, { "id":8, "name":"test project 2", "description":"", "image_url":"", "timezone":"UTC", "owner":15, "created_by":15, "created_at":"2019-02-21 18:36:55", "updated_at":"2019-02-21 18:36:55", "pivot":{ "user_id":1, "project_id":8 } } ] } } } }; } })
table { border-collapse: collapse; width: 100%; } th, td { text-align: left; padding: 8px; } tfoot th { font-weight: normal; } tr:nth-child(even) { background-color: #f2f2f2 }
<script src="https://unpkg.com/vue@2.6.8"></script> <div id="app"> <table> <thead> <tr> <th>Project Name</th> <th>Type</th> </tr> </thead> <tfoot> <tr v-for="project in projects" :key="project.id"> <th>{{ project.name }}</th> <th>{{ project.id }}</th> </tr> </tfoot> </table> </div>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.