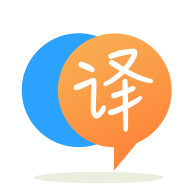
[英]generic function to create an array of nested objects from a single object of arrays
[英]Create new object from array of nested arrays that contains objects
我有以下数组与嵌套数组:
const options = [
[
{
label: "Blue",
option_id: "1"
},
{
label: "Small",
option_id: "2"
}
],
[
{
label: "Red",
option_id: "1"
},
{
label: "Large",
option_id: "2"
}
]
];
我想从每对创建一个对象数组,例如:
[
{
label: ”Blue Small“,
option_id: [1,2]
},
...
]
编辑 :感谢大家的精彩答案
在options
数组上使用.map
,并将每个子数组reduce
为一个对象:
const options = [ [ { label: "Blue", option_id: "1" }, { label: "Small", option_id: "2" } ], [ { label: "Red", option_id: "1" }, { label: "Large", option_id: "2" } ] ]; const result = options.map(arr => arr.reduce( (a, { label, option_id }) => { a.label += (a.label ? ' ' : '') + label; a.option_id.push(option_id); return a; }, { label: '', option_id: [] } ) ); console.log(result);
reduce是这些数组转换的好方法。
const options = [
[
{
label: "Blue",
option_id: "1"
},
{
label: "Small",
option_id: "2"
}
],
[
{
label: "Red",
option_id: "1"
},
{
label: "Large",
option_id: "2"
}
]
];
const newArray = options.reduce((prev,current)=>{
const label = current.map(o=>o.label).join(' ')
const optionid = current.map(o=>o.option_id)
return [...prev,{option_id:optionid,label}]
},[])
console.log(newArray)
您可以映射和收集数据。
const options = [[{ label: "Blue", option_id: "1" }, { label: "Small", option_id: "2" }], [{ label: "Red", option_id: "1" }, { label: "Large", option_id: "2" }]], result = options.map(a => a.reduce((r, { label, option_id }) => { r.label += (r.label && ' ') + label; r.option_id.push(option_id); return r; }, { label: '', option_id: [] })); console.log(result);
.as-console-wrapper { max-height: 100% !important; top: 0; }
使用map
& reduce
。 map
将返回一个新数组并在map的回调函数内,使用reduce
,默认情况下在accumulator中传递一个对象。
const options = [ [{ label: "Blue", option_id: "1" }, { label: "Small", option_id: "2" } ], [{ label: "Red", option_id: "1" }, { label: "Large", option_id: "2" } ] ]; let k = options.map(function(item) { return item.reduce(function(acc, curr) { acc.label = `${acc.label} ${curr.label}`.trim(); acc.option_id.push(curr.option_id) return acc; }, { label: '', option_id: [] }) }); console.log(k)
你可以使用map()
和reduce()
:
const options = [ [ { label: "Blue", option_id: "1" }, { label: "Small", option_id: "2" } ], [ { label: "Red", option_id: "1" }, { label: "Large", option_id: "2" } ] ]; const result = options.map((x) => { let label = x.reduce((acc, val) => { acc.push(val.label); return acc; }, []).join(','); let optArr = x.reduce((acc, val) => { acc.push(val.option_id); return acc; }, []); return { label: label, option_id: optArr } }); console.log(result);
你总是可以迭代地进行迭代,首先使用带编号的for循环来编写东西:
const options = [[{label: "Blue",option_id: "1"},{label: "Small",option_id: "2"}], [{label: "Red",option_id: "1"},{label: "Large",option_id: "2"}]]; const result = []; for(var i=0; i<options.length; i++){ var arr = options[i]; var labels = []; var ids = []; for(var j=0; j<arr.length; j++){ var opt = arr[j]; labels.push(opt.label); ids.push(opt.option_id); } result.push({label:labels.join(" "),option_id:ids}); } console.log(result);
然后扔掉指数,用forEach
const options = [[{label: "Blue",option_id: "1"},{label: "Small",option_id: "2"}], [{label: "Red",option_id: "1"},{label: "Large",option_id: "2"}]]; const result = []; options.forEach(arr => { var labels = []; var ids = []; arr.forEach(opt => { labels.push(opt.label); ids.push(opt.option_id); }); result.push({label:labels.join(" "),option_id:ids}); }); console.log(result);
然后外部循环可以简单地map
:
const options = [[{label: "Blue",option_id: "1"},{label: "Small",option_id: "2"}], [{label: "Red",option_id: "1"},{label: "Large",option_id: "2"}]]; const result = options.map(arr => { var labels = []; var ids = []; arr.forEach(opt => { labels.push(opt.label); ids.push(opt.option_id); }); return {label:labels.join(" "),option_id:ids}; }); console.log(result);
并尝试reduce
内循环:
const options = [[{label: "Blue",option_id: "1"},{label: "Small",option_id: "2"}], [{label: "Red",option_id: "1"},{label: "Large",option_id: "2"}]]; const result = options.map(arr => (lists => ({label:lists.labels.join(" "),option_id:lists.ids})) (arr.reduce((lists,opt) => { lists.labels.push(opt.label); lists.ids.push(opt.option_id); return lists; },{labels:[],ids:[]})) ); console.log(result);
arr.reduce
事件是这里的lists =>
函数的一个参数(它取代了前面片段中的简单return
行)。 我只是想保持join
,但它是一个propably有点过头这种方式。 因此,保持显式变量没有任何问题。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.