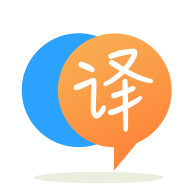
[英]How to resolve error, “The name 'myClass' does not exist in the current context” for unit test?
[英]How to resolve “The name *** does not exist in the current context” error?
我试图在此代码中添加一个Try / Catch块,但是我不知道要进行哪些更改才能使其正常工作。
有什么建议么? 收到此错误:
“错误CS0103名称'fahrenheit'在当前上下文中不存在”
class Program
{
static int FahrenheitToCelsius(int fahrenheit)
{
int celsius = ((fahrenheit - 32) * 5) / 9;
return celsius;
}
static void Main(string[] args)
{
int celsius;
Console.WriteLine("Hello! Welcome to the sauna!");
Console.WriteLine();
Console.WriteLine("Please enter your desired degrees in Fahrenheit: ");
do
{
try
{
int fahrenheit = Convert.ToInt32(Console.ReadLine());
}
catch (FormatException)
{
}
celsius = FahrenheitToCelsius(fahrenheit);
Console.WriteLine("The sauna is now set to " + fahrenheit +
" degrees Fahrenheit, which equals to " + celsius + " degrees Celsius.");
if (celsius < 25)
{
Console.WriteLine("Way too cold! Turn the heat up: ");
}
else if (celsius < 50)
{
Console.WriteLine("Too cold, turn the heat up: ");
}
else if (celsius < 73)
{
Console.WriteLine("Just a little bit too cold, turn the heat up a " +
"little to reach the optimal temperature: ");
}
else if (celsius == 75)
{
Console.WriteLine("The optimal temperature has been reached!");
}
else if (celsius > 77)
{
Console.WriteLine("Too hot! Turn the heat down: ");
}
else
Console.WriteLine("The sauna is ready!");
{
}
} while (celsius < 73 || 77 < celsius);
Console.ReadLine();
}
}
这里有两个问题。 主要的问题是Fahrenheit
需要在try
块外声明,因为您在该块外使用它(变量的作用域是声明它们的块)。 尽管通常最好在所需的最深范围内声明变量,但将其移动到定义celsius
似乎是合乎逻辑的。
第二件事是您应该使用int.TryParse
方法来验证用户输入,而不是使用try/catch
块。 它的性能要好得多,并且更干净,更有针对性。 阅读此问题的答案以获取更多信息。
如果成功,此方法将返回true
,并接受一个string
进行解析(我们直接在下面使用Console.ReadLine()
的返回值),以及一个成功将设置为转换后的整数的out
参数。
这些更改可能类似于:
private static void Main(string[] args)
{
int celsius;
Console.WriteLine("Hello! Welcome to the sauna!\n");
do
{
// Use a loop with TryParse to validate user input; as long as
// int.TryParse returns false, we continue to ask for valid input
int fahrenheit;
do
{
Console.Write("Please enter your desired degrees in Fahrenheit: ");
} while (!int.TryParse(Console.ReadLine(), out fahrenheit));
celsius = FahrenheitToCelsius(fahrenheit);
// Rest of loop code omitted...
} while (celsius < 73 || 77 < celsius);
Console.ReadLine();
}
您可以做一些不同的事情。
int celcisus
也int fahrenheit
try
,如果有任何失败,请直接try
。 看下面的代码:
try
{
int fahrenheit = Convert.ToInt32(Console.ReadLine());
}
在以后尝试使用它时,仅在try {}
块内部存在fahrenheit
。 将其移至父范围:
int fahrenheit;
while (true)
{
try
{
fahrenheit = Convert.ToInt32(Console.ReadLine());
break;
}
catch (FormatException)
{
continue;
}
}
celsius = FahrenheitToCelsius(fahrenheit);
请注意,在FormatException
情况下,没有什么要计算的,因此我在catch
块内添加了带有continue
while (true)
循环。 我也建议在这里使用Int32.TryParse
方法,它将以bool
返回解析结果,而不是引发异常。
您必须在外部作用域中声明该变量以处理异常,才能使用Calid C#程序。
这样,您将最终获得在转换成功时分配的变量,否则,您只需为用户获取值即可:
class Program
{
static int FahrenheitToCelsius(int fahrenheit)
{
int celsius = ((fahrenheit - 32) * 5) / 9;
return celsius;
}
static void Main(string[] args)
{
int celsius;
Console.WriteLine("Hello! Welcome to the sauna!");
Console.WriteLine();
Console.WriteLine("Please enter your desired degrees in Fahrenheit: ");
do
{
// you have to declare the variable out of the scope
int fahrenheit;
try
{
fahrenheit = Convert.ToInt32(Console.ReadLine());
}
catch (FormatException)
{
// and here you have to handle the exception
Console.WriteLine("Invalid value.");
continue;
}
celsius = FahrenheitToCelsius(fahrenheit);
Console.WriteLine("The sauna is now set to " + fahrenheit + " degrees Fahrenheit, which equals to " + celsius + " degrees Celsius.");
if (celsius < 25)
{
Console.WriteLine("Way too cold! Turn the heat up: ");
}
else if (celsius < 50)
{
Console.WriteLine("Too cold, turn the heat up: ");
}
else if (celsius < 73)
{
Console.WriteLine("Just a little bit too cold, turn the heat up a little to reach the optimal temperature: ");
}
else if (celsius == 75)
{
Console.WriteLine("The optimal temperature has been reached!");
}
else if (celsius > 77)
{
Console.WriteLine("Too hot! Turn the heat down: ");
}
else
Console.WriteLine("The sauna is ready!");
{
}
} while (celsius < 73 || 77 < celsius);
Console.ReadLine();
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.