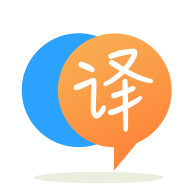
[英]Can not print in java console the min, avg, max value of a MySql table
[英]How can I print to the console this table of numbers in Java?
要求一个自然数n,我想以这种格式打印到控制台:
1
2 1
3 2 1
4 3 2 1
5 4 3 2 1
.
.
.
n . . . 5 4 3 2 1
输入4,这是我到目前为止:
1
21
321
4321
我想在数字之间添加一个空格。 这是我的代码:
import java.util.Scanner;
public class PatternTwo {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int userInput;
System.out.println("Please enter a number 1...9 : ");
userInput = in.nextInt();
String s="";
int temp = userInput;
for(int i=1; i<=userInput; i++ ) {
for (int k= userInput; k>=i; k-- ) {
System.out.printf(" ");
}
for(int j =i; j>=1; j-- ) {
System.out.print(j);
}
System.out.println("");
}
}
}
在要打印的数字前面添加一个空格,并将上面的空格加倍,使其不是金字塔。 像这样的东西:
import java.util.Scanner;
public class PatternTwo {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int userInput;
System.out.println("Please enter a number 1...9 : ");
userInput = in.nextInt();
String s="";
int temp = userInput;
for(int i=1; i<=userInput; i++ ) {
for (int k= userInput; k>i; k-- ) { // <- corrected condition
System.out.printf(" ");
}
for(int j = i; j>=1; j-- ) {
System.out.print(j);
// check if not 1 to avoid a trailing space
if (j != 1) {
System.out.print(" ");
}
}
System.out.println("");
}
}
}
编辑
感谢/ u / shash678我纠正了我的解决方案,删除了所有不必要或错误的空格
如何更清洁的解决方案,避免使用嵌套的for循环:
public static void main(final String[] args) {
final Scanner scanner = new Scanner(System.in);
System.out.print("Please enter a number 1...9 : ");
final int n = scanner.nextInt();
final ArrayList<String> suffixes = new ArrayList<>();
for (int i = 1; i <= n; i++) {
suffixes.add(0, i + " ");
final String prefix = String.join("", Collections.nCopies(n - i, " "));
final String suffix = String.join("", suffixes);
System.out.println(prefix + suffix);
}
}
下面是你的答案的修改,当k
等于i
通过修改你的k for-loops退出条件,并且当j
等于i
通过分别处理该情况,不会打印不必要的额外空格。
主要的整体变化是在你的k循环中你需要打印2个空格而不是1来实现你想要的右侧对齐:
import java.util.Scanner;
class PatternTwo {
private static void printPatternTwo(int n) {
for (int i = 1; i <= n; i++) {
for (int k = n; k > i; k--) {
System.out.print(" ");
}
for (int j = i; j >= 1; j--) {
System.out.print(j == i ? j : " " + j);
}
System.out.println();
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Please enter an integer between 1 and 9 inclusive: ");
int userNum = -1;
while (scanner.hasNext()) {
if (scanner.hasNextInt()) {
userNum = scanner.nextInt();
if (userNum >= 1 && userNum <= 9) {
scanner.close();
break;
} else {
System.out.println("ERROR: Input number was not between 1 and 9");
System.out.print("Enter a single digit number: ");
}
} else {
System.out.println("ERROR: Invalid Input");
System.out.print("Please enter an integer between 1 and 9 inclusive: ");
scanner.next();
}
}
printPatternTwo(userNum);
}
}
用法示例:
Please enter an integer between 1 and 9 inclusive: 12
ERROR: Input number was not between 1 and 9
Enter a single digit number: a
ERROR: Invalid Input
Please enter an integer between 1 and 9 inclusive: 5
1
2 1
3 2 1
4 3 2 1
5 4 3 2 1
打印数字时,从中放入一个空格。
// this works up n == 9
System.out.print(" " + j);
对于n> 9,问题是您需要在固定数量的空间上打印数字和空格,但是数字包含的字符数包含更改。 一个解决方案是使用tab(如果你想要非常大的数字,可以使用几个)。
// when printing blank spaces use a tab instead
for (int k= userInput; k>=i; k-- ) {
System.out.printf("\t");
}
// then print number with tab in front
for(int j =i; j>=1; j-- ) {
System.out.print("\t"+j);
}
如果你对溪流感到满意:
public class PatternTwo {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int userInput;
System.out.println("Please enter a number 1...9 : ");
userInput = in.nextInt();
String s = "";
int temp = userInput;
revRange(1, temp).forEach(n -> printNumbers(n, temp));
}
static IntStream revRange(int from, int to) {
return IntStream.range(from, to)
.map(i -> to - i + from - 1);
}
private static void printNumbers(int n, int max) {
IntStream.rangeClosed(0, n -1)
.forEach(num -> System.out.print(" "));
revRange(1, max - n)
.forEach(num -> System.out.print(num + " "));
System.out.println();
}
}
另一种替代方法是使用printf
分隔每行的间距,并为每个数字使用String.format
。
String.format("%1$" + length + "s", inputString)
如果inputString.length
> = length
则返回inputString
,否则inputString用空格填充,以使长度等于给定的长度。
例:
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int userInput;
System.out.println("Please enter a number 1...9 : ");// you can use this also for numbers > 9
userInput = in.nextInt();
int digits = String.valueOf(userInput).length()+1;
for(int i = 1; i<=userInput; i++){
System.out.printf("%1$"+(digits*(userInput-i+1))+"s","");
for(int k = userInput - (userInput-i); k >= 1; k--){
System.out.print(String.format("%1$"+digits+"s", k));
}
System.out.println();
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.