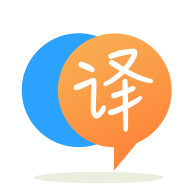
[英]How to use IF ELSE statement in DataBinder.Eval in asp.net
[英]How to assign a score system to a large if-else statement ASP.Net
我正在从事一个项目,该项目由一个医疗患者记录系统组成,该系统在其中存储了大量患者信息。 当医生/护士添加新患者时,他们必须查看大量病史情况等,例如患者是否患有癌症、是否吸烟、是否肥胖等。每个变量都是布尔值,并且是声明为 yes 或 no,值为 1 或 0。
需要患者输入表上的所有变量,因为它们是更大的风险评估计算器的一部分。 这些条件中的每一个都有特定的值,例如,如果患者患有癌症,则选择是。 这将是为每个患者添加的评分系统 +1 分。 某些变量(例如肥胖症)的答案会超过 1 个,因此它们会得到 0/1/2 分。
所有这些变量都放在一个patient.cs 类中,BMI 计算器等功能也是如此。 我在哪里以及如何为我的每个变量分配分数? (有超过 15 个不同的变量,所以它必须是一个很大的 if-else 语句)
任何援助将不胜感激。
- - - - - - - - - - - -[编辑] - - - - - - - - - - - - -------------
The code below is my patient.cs class as of now
public class Patient
{
//The patients ID
[Key]
[DatabaseGenerated(DatabaseGeneratedOption.Identity)]
public int patientId { get; set; }
[ForeignKey("ApplicationUser")]
public string Id { get; set; }
public virtual ApplicationUser ApplicationUser { get; set; }
public string firstName { get; set; }
public string middleName { get; set; }
public string lastName { get; set; }
public string gender { get; set; }
public DateTime birthdate { get; set; }
public decimal weight { get; set; }
public decimal height { get; set; }
/* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Pre-existing risk factors
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
*/
//Parity is the amount of pregnancies had
public int parity { get; set; }
// single, married, seperated or divorced
public string civilStatus { get; set; }
// yes = 4 | no = 0
public bool previousVTE { get; set; }
// Provoked by major surgery? yes = 3 | no = 0
public bool surgeryVTE { get; set; }
// yes = 3 | no = 0
public bool highRiskThrombophilia { get; set; }
// yes = | no =
public bool cancer { get; set; }
// yes = 3 | no = 0
public bool heartFailure { get; set; }
// yes = 3 | no = 0
public bool activeSystemicLupusErythematosus { get; set; }
// yes = 3 | no = 0
public bool inflammatoryPolyarthropathy { get; set; }
// yes = 3 | no = 0
public bool inflammatoryBowelDisease { get; set; }
// yes = 3 | no = 0
public bool nephroticSyndrome { get; set; }
// yes = 3 | no = 0
public bool typeIDiabetesMellitusWithNephropathy { get; set; }
// yes = 3 | no = 0
public bool sickleCellDisease { get; set; }
// yes = 3 | no = 0
public bool currentInratvenousDrugUser { get; set; }
// Family history of unprovoked / estrogen-related VTE in first-degree relative? yes = 1 | no = 0
public bool familyHistoryVTEFirstDegreeRelative { get; set; }
// yes = 1 | no = 0
public bool lowRiskThrombophilia { get; set; }
// yes = 1 | no = 0
public bool smoker { get; set; }
// yes = 1 | no = 0
public bool grossVaricoseVeins { get; set; }
// 0 = no, 1=>30 2=>40
public string obesity { get; set; }
/* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Obstetric risk factors
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
*/
// yes = 1 | no = 0
public bool preEclampsiaInCurrentPregnancy { get; set; }
// ART/IVF (antenatal only) yes = 1 | no = 0
public bool ARTorIVF { get; set; }
// yes = 1 | no = 0
public bool multiplePregnancy { get; set; }
// yes = 2 | no = 0
public bool caesareanSectionInLabour { get; set; }
// yes = 1 | no = 0
public bool electiveCaesareanSection { get; set; }
// Mid-cavity or rotational operative delivery yes = 1 | no = 0
public bool operativeDelivery { get; set; }
// Prolonged labour (> 24 hours) yes = 1 | no = 0
public bool prolongedLabour { get; set; }
// PPH (> 1 litre or transfusion) yes = 1 | no = 0
public bool PPH { get; set; }
// Preterm birth < 37+0 weeks in current pregnancy yes = 1 | no = 0
public bool pretermBirth { get; set; }
// Stillbirth in current pregnancy yes = 1 | no = 0
public bool stillBirth { get; set; }
/* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Transient risk factors
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
*/
// Any surgical procedure in pregnancy or puerperium except immediate repair of the
// perineum, e.g.appendicectomy, postpartum sterilisation yes = 3 | no = 0
public bool surgicalProcedure { get; set; }
// yes = 3 | no = 0
public bool Hyperemesis { get; set; }
// yes OHSS(first trimester only = 4 | no = 0
public bool OHSS { get; set; }
// yes = 1 | no = 0
public bool currentSystemicInfection { get; set; }
// yes = 1 | no = 0
public bool immobilityOrDehydration { get; set; }
//public string middleName { get; set; }
private ApplicationDbContext _dbContext;
public Patient()
{
this._dbContext = new ApplicationDbContext();
}
public void AddToDatabase()
{
_dbContext.Patients.Add(this);
_dbContext.SaveChanges();
}
编写一系列表示每个风险因素得分的表达式,并将它们放入一个数组中。
var riskFactors = new Func<Patient,float>[]
{
p => p.cancer ? 3.0F : 0F,
p => p.multiplePregnancy ? 2.0F : 0F
p => p.caesareanSectionInLabour ? 1.0F : 0.F,
//One expression per item in the questionnaire
};
然后您只需将它们相加即可获得患者的总分:
public float GetScoreForPatient(Patient patient)
{
return riskFactors.Select
(
factor => factor(patient)
)
.Sum();
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.