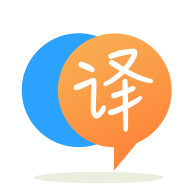
[英]How to remove set of item values of array from another array in Javascript
[英]how to remove array from another array in javascript
0: {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500, …}
1: {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500, …}
2: {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500, …}
我想删除数组中的0:{}
数组。 我该如何删除? 以及如何找到第一项的价值?
由于数组的第一个元素始终为索引0
,因此可以使用Array.prototype.shift
删除第一个元素:
const array = [{ id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500 }, { id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500 }, { id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500 }]; let remainingArray = array; remainingArray.shift(); console.log(remainingArray);
.as-console-wrapper { max-height: 100% !important; top: auto; }
可以有多种方法。
shift()
let arr = [{id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500}, {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500}, {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500}] arr.shift(); console.log(arr);
注意 : shift()
将修改原始数组。
splice()
let arr = [{id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500}, {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500}, {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500}] arr.splice(0,1); console.log(arr);
注意 : splice()
将修改原始数组。
slice
let arr = [{id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500}, {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500}, {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500}] let res = arr.slice(1) console.log(res);
let arr = [{id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500}, {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500}, {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500}] const [,...rest] = arr console.log(rest);
//try this on your console. You can use the shift operator to shift the first element.
//also to remove the last element use pop
>>var myArr = [{id : 1, name: "A"}, {id: 2, name: "B"}, {id:3, name: "C"}];
undefined
>>myArr.shift(0);
{id: 1, name: "A"}
>>myArr
0: {id: 2, name: "B"}
1: {id: 3, name: "C"}
这是有关Array.protoType.shift()的详细链接,该链接删除了第一个元素:
据我从您的问题中了解到,您必须像下面这样从Array1中删除Array2,
Array1 = 0: {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500, …}
1: {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500, …}
2: {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500, …}
Array2 = 0: {id: 1553825061863, name: "Thai Milk Tea", qty: "1", total_amount: 9500, toppings: 500, …}
如果是这样,请使用过滤器功能尝试以下操作。
var data = Array1;
var selectedRows = Array2;
var unSelectedRows = [];
var unSelectedRows = data.filter( function( el ) {
return !selectedRows.includes( el );
} );
您可以在unSelectedRows数组中获得第1个和第2个元素。
使用以下方法删除一个数组索引表单数组的简单方法
var ar = ['zero', 'one', 'two', 'three'];
ar.shift(); // returns "zero"
console.log( ar );
如果是这样的数组,则可以使用以下命令删除0索引。
var ar = [
{ id: 155382506003, toppings: 500},
{ id: 155382506002, toppings: 100},
{ id: 155382506001, toppings: 200}
];
ar.shift();
console.log( ar );
您有一个Javascript对象数组。 您可以通过以下方法删除第一个元素:
使用shift
功能,例如:
var first = fruits.shift(); // remove Apple from the front
使用splice
功能,例如-通过索引位置删除元素:
var removedItem = fruits.splice(pos, 1); // this is how to remove an item
您可以按索引访问元素的值,例如:
var first = fruits[0];
您可以使用foreach
查找字段的值,例如:
fruits.forEach(function(item, index, array) {
if (item.id === 1553825061863) {
console.log(item, index);
}
});
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.