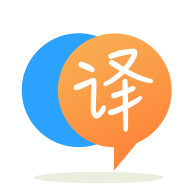
[英]Unhandled Exception: Bad state: Cannot add new events after calling close?
[英]flutter: Unhandled Exception: Bad state: Cannot add new events after calling close
我正在尝试使用 bloc 模式来管理来自 API 的数据并将它们显示在我的小部件中。 我能够从 API 获取数据并对其进行处理并显示它,但我使用的是底部导航栏,当我将选项卡和 go 更改为上一个选项卡时,它返回此错误:
未处理的异常:错误 state:调用关闭后无法添加新事件。
我知道这是因为我正在关闭 stream 然后尝试添加它,但我不知道如何修复它,因为不处理发布主题将导致publishsubject
memory leak
。 这是我的用户界面代码:
class CategoryPage extends StatefulWidget {
@override
_CategoryPageState createState() => _CategoryPageState();
}
class _CategoryPageState extends State<CategoryPage> {
@override
void initState() {
serviceBloc.getAllServices();
super.initState();
}
@override
void dispose() {
serviceBloc.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return StreamBuilder(
stream: serviceBloc.allServices,
builder: (context, AsyncSnapshot<ServiceModel> snapshot) {
if (snapshot.hasData) {
return _homeBody(context, snapshot);
}
if (snapshot.hasError) {
return Center(
child: Text('Failed to load data'),
);
}
return CircularProgressIndicator();
},
);
}
}
_homeBody(BuildContext context, AsyncSnapshot<ServiceModel> snapshot) {
return Stack(
Padding(
padding: EdgeInsets.only(top: screenAwareSize(400, context)),
child: _buildCategories(context, snapshot))
],
);
}
_buildCategories(BuildContext context, AsyncSnapshot<ServiceModel> snapshot) {
return Padding(
padding: EdgeInsets.symmetric(vertical: 20),
child: GridView.builder(
gridDelegate: SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 3, crossAxisSpacing: 3.0),
itemCount: snapshot.data.result.length,
itemBuilder: (BuildContext context, int index) {
return InkWell(
child: CategoryWidget(
title: snapshot.data.result[index].name,
icon: Icons.phone_iphone,
),
onTap: () {},
);
},
),
);
}
这是我的集团代码:
class ServiceBloc extends MainBloc {
final _repo = new Repo();
final PublishSubject<ServiceModel> _serviceController =
new PublishSubject<ServiceModel>();
Observable<ServiceModel> get allServices => _serviceController.stream;
getAllServices() async {
appIsLoading();
ServiceModel movieItem = await _repo.getAllServices();
_serviceController.sink.add(movieItem);
appIsNotLoading();
}
void dispose() {
_serviceController.close();
}
}
ServiceBloc serviceBloc = new ServiceBloc();
我没有包含 repo 和 API 代码,因为它不在此错误的主题中。
使用StreamController.isClosed
检查控制器是否关闭,如果未关闭,则向其添加数据。
if (!_controller.isClosed)
_controller.sink.add(...); // safe to add data as _controller isn't closed yet
从文档:
流控制器是否关闭以添加更多事件。
控制器通过调用 close 方法关闭。 无法通过调用 add 或 addError 将新事件添加到关闭的控制器。
如果控制器关闭了,“done”事件可能还没有传递,但是已经被调度了,再添加更多的事件已经来不及了。
如果错误实际上是由您发布的代码引起的,我只需添加一个检查以确保在调用dispose()
后没有添加新事件。
class ServiceBloc extends MainBloc {
final _repo = new Repo();
final PublishSubject<ServiceModel> _serviceController =
new PublishSubject<ServiceModel>();
Observable<ServiceModel> get allServices => _serviceController.stream;
getAllServices() async {
// do nothing if already disposed
if(_isDisposed) {
return;
}
appIsLoading();
ServiceModel movieItem = await _repo.getAllServices();
_serviceController.sink.add(movieItem);
appIsNotLoading();
}
bool _isDisposed = false;
void dispose() {
_serviceController.close();
_isDisposed = true;
}
}
ServiceBloc serviceBloc = new ServiceBloc();
我遇到了同样的错误,并注意到如果您检查 isClosed,则屏幕不会更新。 在您的代码中,您必须从 Bloc 文件中删除最后一行:
ServiceBloc serviceBloc = new ServiceBloc();
并将这一行放在CategoryPage 中initState() 之前。 这样,您的小部件正在创建和处置该集团。 以前,小部件只处理 bloc,但在重新创建小部件时永远不会重新创建它。
除了提供的解决方案之外,我认为您还应该通过以下方式排出 ServiceBloc 中使用的流allServices
:
@override
void dispose() {
...
allServices?.drain();
}
@cwhisperer 是绝对正确的。 如下所示,在小部件中初始化和处理您的块。
final ServiceBloc serviceBloc = new ServiceBloc();
@override
void initState() {
serviceBloc.getAllServices();
super.initState();
}
@override
void dispose() {
serviceBloc.dispose();
super.dispose();
}
并删除ServiceBloc serviceBloc = new ServiceBloc();
从你的class ServiceBloc
ServiceBloc serviceBloc = new ServiceBloc();
// 删除此代码 // 不要在同一页面中初始化会导致错误状态的类。
我在生产中也遇到了这个问题,我意识到我们应该在释放 Widget 时释放 BehaviorSubject(或任何其他 StreamController),或者在添加新值之前检查 Stream 是否关闭。
这是一个很好的扩展来完成所有工作:
extension BehaviorSubjectExtensions <T> on BehaviorSubject<T> {
set safeValue(T newValue) => isClosed == false ? add(newValue) : () {};
}
你可以像这样使用它:
class MyBloc {
final _data = BehaviorSubject<String>();
void fetchData() {
// get your data from wherever it is located
_data.safeValue = 'Safe to add data';
}
void dispose() {
_data.close();
}
}
如何在 Widget 中配置:
class CategoryPage extends StatefulWidget {
@override
_CategoryPageState createState() => _CategoryPageState();
}
class _CategoryPageState extends State<CategoryPage> {
late MyBloc bloc;
@override
void initState() {
bloc = MyBloc();
bloc.fetchData();
super.initState();
}
@override
void dispose() {
bloc.dispose();
super.dispose();
}
// Other part of your Widget
}
更好的是,如果您不确定在处理后不会重用流:
在关闭流之前调用流上的 drain() 函数。
dispose() async{
await _coinDataFetcher.drain();
_coinDataFetcher.close();
_isdisposed = true;
}
使用 flutter_bloc 时不必担心内存泄漏,因为使用 bloc 时,如果您已使用 bloc 提供程序注入 bloc,则无需手动关闭 bloc。 如 flutter_bloc 文档中所述,Bloc Providers 会为您开箱即用地处理这些问题。
BlocProvider 负责创建 bloc,它会自动处理关闭 bloc
您可以在您的应用程序中对此进行测试。 尝试在 bloc 的close()
覆盖上打印。
如果从导航堆栈中删除了提供该块的屏幕,则该给定块的close()
方法将被立即调用。
检查 bloc/cubit 是否由isClosed
变量关闭。 将此 if 条件包装到那些抛出异常的状态。
示例代码
class LandingCubit extends Cubit<LandingState> {
LandingCubit(this.repository) : super(LandingInitial());
final CoreRepository repository;
// Fetches image urls that needs to shown in landing page
void getLandingImages() async {
emit(LandingImagesLoading());
try {
List<File> landingImages = await repository.landingImages();
if (!isClosed) {
emit(LandingImagesSuccess(landingImages));
}
} catch (e) {
if (!isClosed) {
emit(LandingImagesFetchError(e.toString()));
}
}
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.