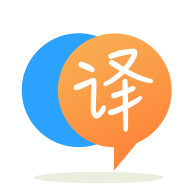
[英]Deserialize JSON with spring : Unresolved forward references Jackson Exception
[英]Jackson: Unresolved forward references when trying to deserialize JSON into java objects
很长一段时间以来,我一直被Jackson
困在一个问题上,我很想解决它。 我有一个JSON
,其中包含使用 ID 相互引用的对象,我需要将其反序列化为对象,但是在尝试这样做时,我不断收到Unresolved forward references exception
。
我曾尝试在相关类上方使用Jackson
注释@JsonIdentityInfo
,但这没有产生任何结果。
JSON 示例:
{
"customer": [
{
"id": 1,
"name": "Jane Gallow",
"age": 16
},
{
"id": 2,
"name": "John Sharp",
"age": 20
},
],
"shoppingcart": [
{
"id": 1,
"customer": 2,
"orderDate": "2015-02-19"
}
]
}
客户类
@JsonIdentityInfo(generator= ObjectIdGenerators.PropertyGenerator.class, property="id",scope = Customer.class)
@JsonIdentityReference(alwaysAsId = true)
public class Customer {
private int id = -1;
private String name;
private int age;
//getters, setters
}
购物车类
<!-- language: java -->
@JsonIdentityInfo(generator= ObjectIdGenerators.PropertyGenerator.class, property="id",scope = ShoppingCart.class)
public class ShoppingCart {
private int id = -1;
private Customer customer;
@JsonDeserialize(using = LocalDateDeserializer.class)
@JsonSerialize(using = LocalDateSerializer.class)
private LocalDate orderDate = LocalDate.now();
//getters, setters
}
我希望Jackson
给我一个ShoppinCart
对象,该对象引用了 id 为2
Customer
对象(在本例中为 John Sharp)。 但我无法让它工作,当我尝试使用ObjectMapper.readValue()
方法从 JSON 读取时,它给了我"main" com.fasterxml.jackson.databind.deser.UnresolvedForwardReference
。
我在反序列化期间遇到了与未解决的前向引用相同的问题。 我使用https://www.logicbig.com/tutorials/misc/jackson/json-identity-reference.html上提供的示例来检查并解决我的问题。 有一个非常简单的类:
public class Order {
private int orderId;
private List<Integer> itemIds;
private Customer customer;
....
}
我在 Customer 类中添加了 @JsonCreator 静态工厂方法,它没有引起任何问题。
现在,当我向 Order 类添加任何类型的 @JsonCreator(静态方法或构造函数)时,我立即得到未解析的前向引用。 有趣的是,当我添加以下构造函数时,只存在 @JsonPropery 注释:
public Order(@JsonProperty("orderId") int orderId, @JsonProperty("itemIds") List<Integer> itemIds, @JsonProperty("customer") Customer customer) {
this.orderId = orderId;
this.itemIds = itemIds;
this.customer = customer;
}
它还导致未解决的前向引用。
为了解决这个问题,对于 Order 类,你必须创建无参数的构造函数(可以是私有的)。 否则你会得到:
线程“main”com.fasterxml.jackson.databind.exc.InvalidDefinitionException 中的异常:无法构造
com.logicbig.example.Order
实例(没有创建者,如默认构造,存在):无法从对象值反序列化(无委托或基于属性的创建者)
并且不得有任何@JsonCreator 注释的方法和构造函数,或带有@JsonProperty 注释的构造函数。
这就是全部。
您应该在属性上使用JsonIdentityReference
。
@JsonIdentityInfo(generator = ObjectIdGenerators.PropertyGenerator.class, property = "id", scope = ShoppingCart.class)
class ShoppingCart {
private int id = -1;
@JsonIdentityReference
private Customer customer;
private LocalDate orderDate;
// getters, setters, toString
}
@JsonIdentityInfo(generator = ObjectIdGenerators.PropertyGenerator.class, property = "id", scope = Customer.class)
class Customer {
private int id = -1;
private String name;
private int age;
// getters, setters, toString
}
简单的例子:
import com.fasterxml.jackson.annotation.JsonIdentityInfo;
import com.fasterxml.jackson.annotation.JsonIdentityReference;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.ObjectIdGenerators;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import java.io.File;
import java.time.LocalDate;
import java.util.List;
public class JsonApp {
public static void main(String[] args) throws Exception {
File jsonFile = new File("./resource/test.json").getAbsoluteFile();
ObjectMapper mapper = new ObjectMapper();
mapper.registerModule(new JavaTimeModule());
mapper.enable(SerializationFeature.INDENT_OUTPUT);
System.out.println(mapper.readValue(jsonFile, Pojo.class));
}
}
对于您的JSON
打印:
Pojo{customers=[Customer{id=1, name='Jane Gallow', age=16}, Customer{id=2, name='John Sharp', age=20}], shoppingCarts=[ShoppingCart{id=1, customer=Customer{id=2, name='John Sharp', age=20}, orderDate=2015-02-19}]}
也可以看看:
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.