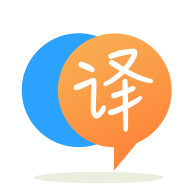
[英]How can I calculate the difference between two times in 24h format using Java?
[英]How can I calculate the difference of chars between two strings and make them equal in Java?
我在学校开始使用Java,但在这个练习中遇到了麻烦。 我有两个数字,我必须找出这两个字符之间的字符差,并使字符数相等,我必须添加多个零作为缺失的字符。 因此,例如,如果我有10和100,则输出将为010和100。我想在理解如何方面有所帮助,有了这两个数字的string.length,我可以检测到缺失的字符并加0。
import java.util.Scanner;
public class exercise {
public static void main(String[] args) {
Scanner myObj = new Scanner(System.in);
System.out.println("Enter n1");
System.out.println("Enter n2");
String n1 = myObj.nextLine();
String n2 = myObj.nextLine();
System.out.println("n1 is: " + n1);
System.out.println("n2 is: " + n2);
System.out.println("length n1: "+ n1.length());
System.out.println("length n2: "+ n2.length());
}
}
我不会为您提供解决方案代码,但会为您提供有关带有上下文的String.length方法的信息。
假设您有两个字符串变量:
n1 = "18214120"
n2 = "100"
n1.length()将产生8,n2.length()将产生3。利用此信息,您知道n1是包含更多字符的数字,并且您希望n2填充0直到n2.length()= n1 .length(),您要在n2的左边添加几个零?
那么要填充的零的数量必须等于:
n1.length()-n2.length()
8-3 = 5。
因此,在n2的左边加上5 0将使它成为00000100,我相信这是您想要的。
得到丢失的字符
int charsMissing = n1.length() - n2.length();
添加额外的零作为丢失的次数。
if (charsMissing > 0) // check if there actually are missing in {
for (int i = 0; i < charsMissing; i++) {
n1 = "0" + n1
}
}
重要的是要知道在更改String对象时,可以使用+
运算符将两个字符串连接在一起。 例如:
String s1 = "hello";
String s2 = "world";
String combined = s1 + s2;
System.out.println(combined);
这将输出:
helloworld
如果要在字符串前面加上数字0,则可以使用以下代码:
String zeroAdded = "0" + someString;
由于您不想为添加的每个零创建新的字符串,因此Java允许您串联一个字符串并将结果分配给它自己。 上面的示例也可以写成:
String someString = "0" + someString; //The value of someString will be overwritten with the result of the concatenation.
现在您所要做的就是重复此步骤X次,即Xs的长度差。 可以使用for循环或while循环。 选择哪个是一个完整的主题,我建议使用谷歌搜索这些术语以获取示例和比较。 对于这些类型的练习,尽管性能或可读性之间的差异可以忽略不计。 这是一个for循环的示例:
int difference = Math.abs(n1.length() - n2.length()) //Taking absolute value, because we don't know which string was the longest.
for(int i = 0; i < difference; i++){
if(n1.length() > n2.length()){ //n1 is longer, so prepend the 0 to n2
n2 = "0" + n2;
}
if(n1.length() < n2.length()){ //n2 is longer, so prepend the 0 to n1
n1 = "0" + n1;
}
}
System.out.println("n1 is now: " + n1);
System.out.println("n2 is now: " + n2);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.