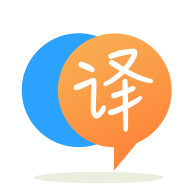
[英]How to check if phone number is already registered in firebase authentication using flutter
[英]How to implement phone number verification in flutter using firebase? (Not Authentication)
如何使用firebase实现flutter的电话号码验证? 我的应用程序需要在某个时间点验证电话号码。 不需要通过电话号码进行身份验证。 我只需要验证我的号码。 我如何实施?
在 Firebase 中,电话号码验证也会自动对用户进行身份验证。 如果用户不登录,则无法使用 Firebase 身份验证来验证用户的电话号码。
在 firebase 中确实如此,您不能只验证电话号码,电话号码验证会自动对用户进行身份验证,但这没关系。 您可以将电话号码链接到已通过身份验证的用户。 链接电话号码意味着用户可以使用他们现有的提供商(gmail、Facebook、email 等)登录,也可以使用他们的电话号码登录并使用相同的帐户密码。
请参阅示例 flutter 代码
_verifyPhoneNumber() async {
await FirebaseAuth.instance.verifyPhoneNumber(
phoneNumber: '+44 7123 123 456',
verificationCompleted: (PhoneAuthCredential credential) {
// For andriod only automatic handling of the SMS code
},
verificationFailed: (FirebaseAuthException e) {},
codeSent: (String verificationId, int? resendToken) {
// SMS Code sent show a dialogue to enter the code.
_displayPhoneNumberVerificationDialog(verificationId);
},
codeAutoRetrievalTimeout: (String verificationId) {},
);
}
在_displayPhoneNumberVerificationDialog(verificationId)
中有一个带有提交按钮的对话。 链接逻辑将是按钮的 onTap。
示例 _displayPhoneNumberVerificationDialog
_displayPhoneNumberVerificationDialogSt(String verificationId) {
FirebaseAuth firebaseAuth = firebase.FirebaseAuth.instance;
return showDialog(
context: context,
builder: (context) {
return AlertDialog(
title: Text('Enter verification code'),
content: TextField(
keyboardType: TextInputType.number,
textInputAction: TextInputAction.done,
decoration: InputDecoration(hintText: "phone number"),
onChanged: (input) {
_smsVerificationCode = input;
},
),
actions: <Widget>[
new FlatButton(
child: new Text('Submit'),
onPressed: () async {
Navigator.of(context).pop();
// Create a PhoneAuthCredential with the code
PhoneAuthCredential credential;
try {
credential = PhoneAuthProvider.credential(
verificationId: verificationId,
smsCode: _smsVerificationCode);
} catch (e) {
// Show Error usually wrong _smsVerfication code entered
return;
}
User currentUser = await firebaseAuth.getCurrentUser();
try {
// This try catch helps if you want to update an existing verified phone number and you want to verify the new one.
// We unlink the old phone number so a new one can be linked
// This is not relevant if you're just going to verify and link a phone number without updating it later.
currentUser = await currentUser.unlink("phone");
} catch (e) {
print(e);
currentUser = await firebaseAuth.getCurrentUser();
}
try {
currentUser.linkWithCredential(credential).then((value) {
// Verfied now perform something or exit.
}).catchError((e) {
// An error occured while linking
});
} catch (e) {
// General error
}
},
)
],
);
});
}
手动执行,使用PhoneNumberUtil类和代码中的手动方法来验证国家/地区代码
在这种情况下,该方法检查号码的国家/地区代码,我指定只允许带有国家/地区代码“KE”的肯尼亚号码
_checkPhoneNumber() async {
var s = phoneTextFieldController.text;
bool isValid;
if (s.length == 0) {
isValid = true;
setState(() {
_isPhoneNumber = isValid;
});
return;
}
//check if the number starts with '0' , or '07' or '254'
if (s.length < 10) {
isValid = s.length == 1 && s[0] == '0' ||
s.length > 1 && s[0] + s[1] == '07' ||
s.length > 1 && s[0] + s[1] + s[2] == '254' ||
s.length > 1 && s[0] + s[1] + s[2] + s[3] == '+254';
setState(() {
_isPhoneNumber = isValid;
});
return;
}
isValid =
await PhoneNumberUtil.isValidPhoneNumber(phoneNumber: s, isoCode: 'KE');
String normalizedNumber = await PhoneNumberUtil.normalizePhoneNumber(
phoneNumber: s, isoCode: 'KE');
RegionInfo regionInfo =
await PhoneNumberUtil.getRegionInfo(phoneNumber: s, isoCode: 'KE');
setState(() {
_isPhoneNumber = isValid;
});
}
然后在你的表格中有
child: TextFormField(
onChanged: (text) {
_checkPhoneNumber();
},
controller: phoneTextFieldController,
keyboardType: TextInputType.phone,
validator: (val) {
if (val == '') {
return 'Phone Number is required';
}
if (!_isPhoneNumber) {
return 'Phone Number format error';
}
},
decoration: InputDecoration(
hintText: "2547xx xxx xxx - Phone",
border: InputBorder.none,
icon: const Icon(
Icons.phone,
),
labelText: 'Phone',
prefixText: ' ',
suffixStyle:
const TextStyle(color: Colors.green)),
onSaved: (value) {
phoneNumberForm = value;
},
)),
然后在表单提交检查表单状态
buttonCustom(
color: Color(0xFF4458be),
heigth: 50.0,
txt: contactIdParam!=null ?"Submit Edit":"Submit",
ontap: () {
final _form = form.currentState;
if (_form.validate()) {
if(contactIdParam!=null){
_editContactRequest();
}else{
_addContactRequest();
}
} else {
print('form is invalid');
}
},
),
注意:从我的工作代码中复制了片段,我的验证将与您想要的不同,但您需要的只是逻辑
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.