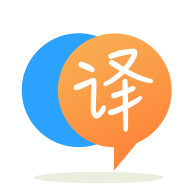
[英]microservice doesn't fetch properties from spring-cloud-config-server microservice
[英]How to get list of files managed by Spring-Cloud-Config-Server
我有 3 个 Spring-Boot 应用程序正在运行:
对于 Spring-Cloud-Config,我使用本地 git URI 来填充数据。 本地仓库在master
分支上,文件结构如下:
./myMicroService
|-- application.properties
|-- foo.txt
|-- bar.txt
根据文档,我可以像这样访问文本文件:
http://localhost:8080/myMicroService/default/master/foo.txt http://localhost:8080/myMicroService/default/master/bar.txt
哪个有效,但我如何获得 Spring-Cloud-Config 服务器提供的可用 *.txt 文件的完整列表?
我试过这个:
http://localhost:8080/myMicroService/default/master
它只返回application.properties
及其值。
鉴于没有针对此的 OOTB 解决方案,我向我的配置服务器添加了一个新的请求映射:
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Collection;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import javax.servlet.http.HttpServletRequest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.config.server.EnableConfigServer;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
import org.springframework.core.io.Resource;
import org.springframework.core.io.ResourceLoader;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@EnableConfigServer
@EnableEurekaClient
@RestController
public class ConfigServer {
public static void main(String[] args) {
SpringApplication.run(ConfigServer.class, args);
}
@Value("${spring.cloud.config.server.git.uri}")
private String uri;
@Autowired private ResourceLoader resourceLoader;
@GetMapping("/{name}/{profile}/{label}/listFiles")
public Collection<String> retrieve(
@PathVariable String name,
@PathVariable String profile,
@PathVariable String label,
HttpServletRequest request)
throws IOException {
Resource resource = resourceLoader.getResource(uri);
String uriPath = resource.getFile().getPath();
Path namePath = Paths.get(uriPath, name);
String baseUrl =
String.format(
"http://%s:%d/%s/%s/%s",
request.getServerName(), request.getServerPort(), name, profile, label);
try (Stream<Path> files = Files.walk(namePath)) {
return files
.map(Path::toFile)
.filter(File::isFile)
.map(File::getName)
.map(filename -> baseUrl + "/" + filename)
.collect(Collectors.toList());
}
}
}
获取 myMicroService 的文件列表:
curl http://localhost:8888/myMicroService/default/master/listFiles
结果:
[
"http://localhost:8888/myMicroService/default/master/application.properties",
"http://localhost:8888/myMicroService/default/master/foo.txt",
"http://localhost:8888/myMicroService/default/master/bar.txt"
]
前面的解决方案假设你的spring.cloud.config.server.git.uri是https类型的。 下面的解决方案将引用包含从回购中提取的文件的本地文件系统,并将适用于任何回购类型。 端点还接受搜索路径并采用以下格式:
/{name}/{profile}/{label}/{path}/listFiles
/{name}/{profile}/{path}/listFiles?useDefaultLabel
@Configuration
@EnableAutoConfiguration
@EnableConfigServer
@RestController
public class CloudConfigApplication {
private UrlPathHelper helper = new UrlPathHelper();
private SearchPathLocator service;
public static void main(String[] args) {
SpringApplication.run(CloudConfigApplication.class, args);
}
public CloudConfigApplication(SearchPathLocator service) {
this.service = service;
}
@RequestMapping("/{name}/{profile}/{label}/**/listFiles")
public List<String> retrieve(@PathVariable String name, @PathVariable String profile,
@PathVariable String label, ServletWebRequest request,
@RequestParam(defaultValue = "true") boolean resolvePlaceholders)
throws IOException {
String path = getDirPath(request, name, profile, label);
return listAll(request, name, profile, label, path);
}
@RequestMapping(value = "/{name}/{profile}/**/listFiles", params = "useDefaultLabel")
public List<String> retrieve(@PathVariable String name, @PathVariable String profile,
ServletWebRequest request,
@RequestParam(defaultValue = "true") boolean resolvePlaceholders)
throws IOException {
String path = getDirPath(request, name, profile, null);
return listAll(request, name, profile, null, path);
}
private String getDirPath(ServletWebRequest request, String name, String profile, String label) {
String stem;
if (label != null) {
stem = String.format("/%s/%s/%s/", name, profile, label);
} else {
stem = String.format("/%s/%s/", name, profile);
}
String path = this.helper.getPathWithinApplication(request.getRequest());
path = path.substring(path.indexOf(stem) + stem.length()).replace("listFiles", "");
return path;
}
public synchronized List<String> listAll(ServletWebRequest request, String application, String profile, String label, String path) {
if (StringUtils.hasText(path)) {
String[] locations = this.service.getLocations(application, profile, label).getLocations();
List<String> fileURIs = new ArrayList<>();
try {
int i = locations.length;
while (i-- > 0) {
String location = String.format("%s%s", locations[i], path).replace("file:", "");
Path filePath = new File(location).toPath();
if(Files.exists(filePath)) {
fileURIs.addAll(Files.list(filePath).filter(file -> !Files.isDirectory(file)).map(file -> {
String URL =
String.format(
"%s://%s:%d%s/%s/%s/%s%s?useDefaultLabel",
request.getRequest().getScheme(), request.getRequest().getServerName(),
request.getRequest().getServerPort(), request.getRequest().getContextPath(),
application, profile, path,
file.getFileName().toString());
if(label != null) {
URL = String.format(
"%s://%s:%d%s/%s/%s/%s/%s%s",
request.getRequest().getScheme(), request.getRequest().getServerName(),
request.getRequest().getServerPort(), request.getRequest().getContextPath(),
application, profile, label, path,
file.getFileName().toString());
}
return URL;
}).collect(Collectors.toList()));
}
}
} catch (IOException var11) {
throw new NoSuchResourceException("Error : " + path + ". (" + var11.getMessage() + ")");
}
return fileURIs;
}
throw new NoSuchResourceException("Not found: " + path);
}
}
获取列表:
curl http://localhost:8080/context/appName/profile/label/some/path/listFiles
示例结果:
[
"http://localhost:8080/context/appName/profile/label/some/path/robots.txt"
]
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.