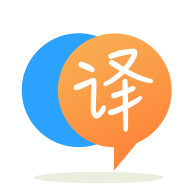
[英]C++ - Using array/pointers in vector (or other container) constructor that expects type Iterator. How is that possible?
[英]C++ Iterator range using pointers to an array
题
我不想将数组的大小作为索引参数传递。
对于我的merge_sort
,我想使用迭代器范围概念优化参数。 我似乎无法弄清楚如何尊重迭代器范围并访问我的数组。 我可以尊重访问诸如指数low
和high
的recursive_merge_sort
,但似乎并没有被访问阵列本身就是一个直观的方式。 我一直使用有关C ++指针和数组的出色指南作为起点。
我的Merge Sort C ++ 11 C ++ 17问题使这个概念浮出水面,我喜欢使用迭代器范围来减少排序参数数量的想法。
码
void recursive_merge_sort(int* low, int* high) {
// deference to get starting index "low" and ending index "high"
if(*(low) >= *(high) - 1) { return; }
int mid = *(low) + (*(high) - *(low))/2;
// what's the correct syntax to access my array from the iterator range
// int* d = some how deference low or how to get the data array they iterate on
recursive_merge_sort_v(d + low, d + mid);
recursive_merge_sort_v(d + mid, d + high);
merge(d + low, mid, d + high);
// delete d;
}
void merge_sort(int* data) {
// what's the correct syntax to access my array from the passed in iterator range
// is this event possible? incorrect syntax below
recursive_merge_sort(data + 0, data + std::size(*(data)));
}
int main()
{
int data[] = { 5, 1, 4, 3, 65, 6, 128, 9, 0 };
int num_elements = std::size(data);
std::cout << "unsorted\n";
for(int i=0; i < num_elements; ++i) {
std::cout << data[i] << " ";
}
merge_sort(data);
std::cout << "\nsorted\n";
for(int i=0; i < num_elements; ++i) {
std::cout << data[i] << " ";
}
}
评论部分来自bayou的解决方案
雷米·勒博(Remy Lebeau):“当您通过指针传递数组时,您将丢失有关该数组的所有信息。仅通过指针/迭代器就无法返回原始数组,因为取消引用只会给您数组的单个元素,而不会给您数组本身。通过指针传递数组时,您别无选择,只能将数组大小作为另一个参数传递;否则,请按引用传递数组,如果需要支持不同大小的数组,请使用模板,因此编译器可以推断出引用的数组大小。”
迭代器被建模为充当指针。 它们具有相同类型的重载运算符:至少要取消引用和递增(某些还具有递减,随机访问等)。
最先进的迭代器接口是随机访问,其功能完全类似于原始指针(根据设计)。
因此,所有(原始)指针基本上都是进入C样式(连续)数组的随机访问迭代器。 查看以下内容以可视化C样式数组的开始/结束迭代器:
int vals[] = { 0, 1, 2, 3, 4 };
int *begin = vals;
int *end = vals + 5;
v vals[]
0 1 2 3 4 ...
^ begin ^ end (1 past the end of array)
vals[2] == begin[2]
vals[4] == begin[4]
etc.
因此,基本上,您只是将begin迭代器视为数组的前部,并且只是不取消引用begin迭代器之前的任何地方,也不要在结束迭代器的位置或之后进行取消引用,因为标准C ++约定规定end迭代器在末尾是1的范围。
这是使用迭代器之类的指针的示例:
void print(int *begin, int *end)
{
// for each element in the range (starting at begin, up to but not including end)
for (; begin != end; ++begin)
{
// print the element
std::cout << *begin << '\n';
}
}
int main()
{
// declare a C-style array
int arr[] = { 10, 5, 2, 6, 20 };
// for the sake of example, print only the middle 3 elements
// begin = arr + 1
// end = arr + 4
print(arr + 1, arr + 4);
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.