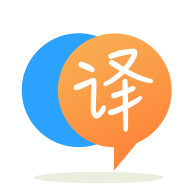
[英]Value of struct variable changes after malloc of other variable of same struct
[英]Updating a variable's value in a struct cause another variable from the same struct to be modified
我正在编写处理随时间推移交叉路口的交通并返回给定时间交叉路口有多少交通的代码。
基本上,我遇到的问题是一个结构数组,其中每个结构包含2个向量:一个具有交叉点的坐标(x,y),另一个跟踪在时间t进入那个交叉点的车辆数量(每个插槽从0到n代表时间,其中的值是当时穿越的汽车数量)。
这是结构:
typedef struct intersection {
int coords[2];
int timeslots[];
} intersection;
它包含在此结构的交集数组中:
typedef struct simulation {
int h_streets; //row (horizontal streets)
int v_streets; //column (vertical streets)
int n_cars; //cars number
int n_trips; //trips number
car car_pos [1000];
intersection intersections [];
} simulation;
然后,在读取所需数据时,使用malloc分配内存以定义灵活数组的实际数组大小:
struct simulation * this = malloc( sizeof(simulation) + (sizeof(intersection)*(h_streets*v_streets)*(sizeof(int)*(max_time+1001))) );
如前所述,当读取有关汽车从一个位置到另一个位置的行程的数据时,我要做的是,当汽车在那个时间穿过交叉点时,将时隙[t]增加一个。
问题是,当我使用while周期中包含的这一行代码时,
this->intersections[curr_int].timeslots[starting_t+travel_t]++;
来自coords数组的值被修改。
这是在每个周期仅打印出相交坐标时的示例:
------------------
i: 0, h: 0, v: 0
i: 1, h: 0, v: 1
i: 2, h: 0, v: 2
i: 3, h: 0, v: 3
i: 4, h: 1, v: 0
i: 5, h: 1, v: 1
i: 6, h: 1, v: 2
i: 7, h: 1, v: 3
i: 8, h: 2, v: 0
i: 9, h: 2, v: 1
i: 10, h: 2, v: 2
i: 11, h: 2, v: 3
i: 12, h: 3, v: 0
i: 13, h: 3, v: 1
i: 14, h: 3, v: 2
i: 15, h: 3, v: 3
------------------
------------------
i: 0, h: 0, v: 0
i: 1, h: 1, v: 1
i: 2, h: 0, v: 2
i: 3, h: 0, v: 3
i: 4, h: 1, v: 0
i: 5, h: 1, v: 1
i: 6, h: 1, v: 2
i: 7, h: 1, v: 3
i: 8, h: 2, v: 0
i: 9, h: 2, v: 1
i: 10, h: 2, v: 2
i: 11, h: 2, v: 3
i: 12, h: 3, v: 0
i: 13, h: 3, v: 1
i: 14, h: 3, v: 2
i: 15, h: 3, v: 3
------------------
------------------
i: 0, h: 0, v: 0
i: 1, h: 1, v: 2
i: 2, h: 0, v: 2
i: 3, h: 0, v: 3
i: 4, h: 1, v: 0
i: 5, h: 1, v: 1
i: 6, h: 1, v: 2
i: 7, h: 1, v: 3
i: 8, h: 2, v: 0
i: 9, h: 2, v: 1
i: 10, h: 2, v: 2
i: 11, h: 2, v: 3
i: 12, h: 3, v: 0
i: 13, h: 3, v: 1
i: 14, h: 3, v: 2
i: 15, h: 3, v: 3
------------------
------------------
i: 0, h: 0, v: 0
i: 1, h: 1, v: 2
i: 2, h: 0, v: 2
i: 3, h: 0, v: 3
i: 4, h: 2, v: 0
i: 5, h: 1, v: 1
i: 6, h: 1, v: 2
i: 7, h: 1, v: 3
i: 8, h: 2, v: 0
i: 9, h: 2, v: 1
i: 10, h: 2, v: 2
i: 11, h: 2, v: 3
i: 12, h: 3, v: 0
i: 13, h: 3, v: 1
i: 14, h: 3, v: 2
i: 15, h: 3, v: 3
------------------
[...]
(i是访问位置i处的交叉点[]的循环的计数器,而h和v是coords []数组中包含的交叉点i的水平和垂直坐标)
如您所见,即使我甚至没有使用增量函数访问该数组,某些交点的坐标也会在每个循环后进行修改
this->intersections[curr_int].timeslots[starting_t+travel_t]++;
这是导致此问题的原因。 这怎么可能? 可能是内存分配问题吗?
在这段代码中,它声明了simulation
的成员:
intersection intersections [];
intersections
是具有柔性数组成员的结构,因此上面的代码尝试创建具有柔性数组成员的结构的数组。
这行不通。 具有柔性阵列构件的结构的大小就好像省略了柔性阵列构件(除了可能存在的有关对齐填充的问题)。 在计算结构大小或执行数组索引计算时,此编译器无法考虑柔性数组的大小。
此代码尝试将结构数组与灵活的数组成员一起使用:
this-> intersections [curr_int] .timeslots [starting_t + travel_t] ++;
在此代码中,编译器无法知道this->intersections[curr_int].timeslots
具有多少个元素。 由于timeslots
是灵活的数组成员,所以intersection
每个实例在其timeslots
中可以具有不同数量的元素-程序员的目的是为每个实例提供所需的任何空间量。 由于此数量可能随每个实例而变化,因此无法将它们排列成固定大小的元素数组,并且编译器无法知道每个元素的大小。
由于编译器不知道程序员打算在每个timeslots
多少个元素,因此不知道每个intersection
有多大。 在计算this->intersections[curr_int]
的地址时,编译器仅使用结构的大小,就好像没有柔性数组成员时定义的那样(填充问题除外)。 当程序员打算使用灵活的数组成员时,此计算将是不正确的。
使用-pedantic
开关时,Clang和GCC均会发出警告。
具有灵活数组成员的结构的数组无法工作。 您必须为结构使用其他设计。 在每个intersection
, timeslots
可以是指向timeslots
数组空间的指针,而不是灵活的数组成员。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.